❔ Creating a json array and reading a specific property
Hello!
So i have a json file as followed
I want to read let's say everything from
embed1
as if it was its own file. The thing to consider is that it has to be a string since the parameter is a string since im reading it like this
I am getting this error and idk what to do... Unexpected character encountered while parsing value: A. Path '', line 0, position 0.
.20 Replies
Just deserialize it all proper-like instead of rawdogging it with
JObject
?Did exactly that.
My code is now
code seems to work now as intended
You're still rawdogging it with
JObject
for some godforsaken reason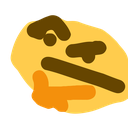
You should deserialize it to a proper class
So you don't need to use magic strings that are prone to typos and what not
so your solution is to simply just create a class holding these values and assign them default values incase they aren't used?
Are they ever not used?
well the thing is, EmbedBuilderUtils holds a lot of values that I don't use such as
Fields[]
Thumbnails
, Images
, Footer
You can only have the properties you do use
And deserialize to that
may i ask, what exactly is considered a bad practice for my code? is there a certain reason this approach is not good?
im assuming the way its used could be subject to a lot of issues
Angius
REPL Result: Success
Result: Foo
Compile: 585.296ms | Execution: 57.417ms | React with ❌ to remove this embed.
Typos would be one thing
In my example, you can access
data.Name
With autocomplete and all that good stuff
Not data["Nmae"]
which would just be a runtime crashi do use that approach as part of my project
the only reason i didn't use it here is because the embed needs to have certain things in there to be fully parsed
i would clutter the class with default values which would then be output to my embed
which i have no control over
Write the class once and forget about it ¯\_(ツ)_/¯
But sure, whatever works
i will see if i can create it and go on from there
thank you!!! 

$jsongen
Instantly parse JSON in any language | quicktype
Whether you're using C#, Swift, TypeScript, Go, C++ or other languages, quicktype generates models and helper code for quickly and safely reading JSON in your apps. Customize online with advanced options, or download a command-line tool.
Convert JSON to C# Classes Online - Json2CSharp Toolkit
Convert any JSON object to C# classes online. Json2CSharp is a free toolkit that will help you generate C# classes on the fly.
You can just generate it 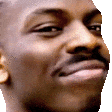
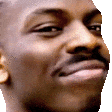
bookmarked
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.