ā EF Core delete error
hi, i tried to fix it but nothing works,
so i have dbcontext
and
so, error only happens when chat have Include (connections / messages). how to make on delete chat don't care about this things?
9 Replies
what error?
and show the code that's causing the error
1 sec, windows update
so, error happens when i call this action
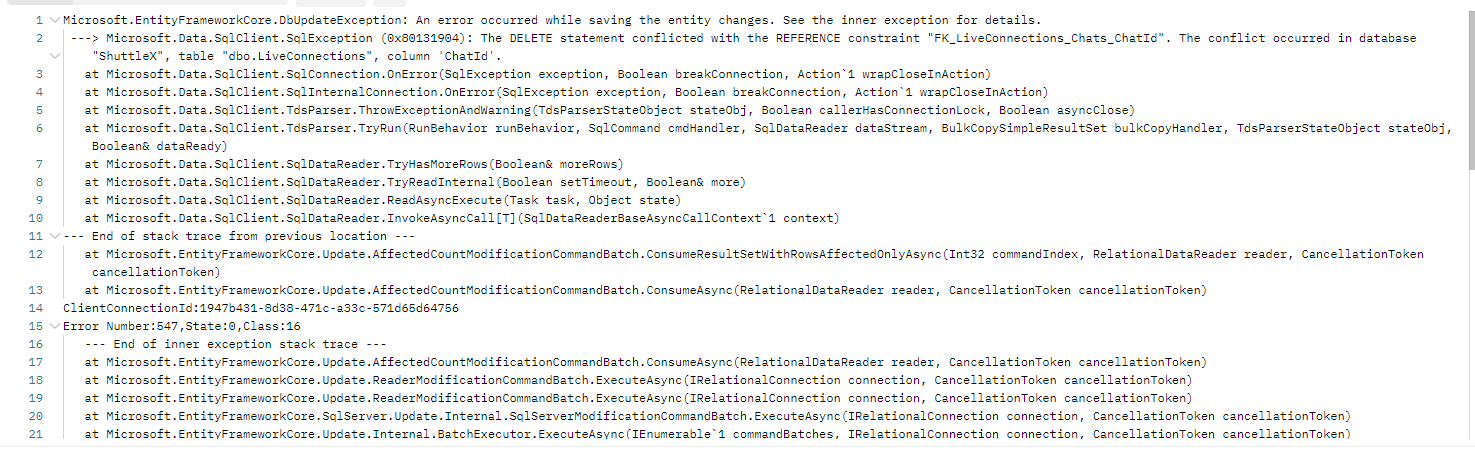
how is your OnDelete set up?
LiveConnections.ChatId would be referring to a Chat that no longer exists
so you either need to manually remove that connection yourself, or set up Cascade or SetNull as the deletion action
i gues OnDelete don't exists in my code, atleast i don't wrote it
so, when i was googling problem, they sain i need to set up cascade delete
but I haven't figured it out how
š¦
also, that EnsureCreated in your db context constructor is gonna bite you
EnsureCreated is not compatible with migraitons
so, i just need to figure out how to cascade delete?
for that thing work
yeah
ok
thanks a lot