❔ EFCore Change Tracker Confusion
Assuming a entity
Order
with a relationship to entity Table
Shouldn't the "sameOrder" be fetched from the tracker and do a single query for the table only? Since it's already being tracked..
Because in my application I'm seeing it fetches the whole order from the start...
Unless there's some kinda of rule about relationships that I couldn't find12 Replies
Seems like the answer relies here
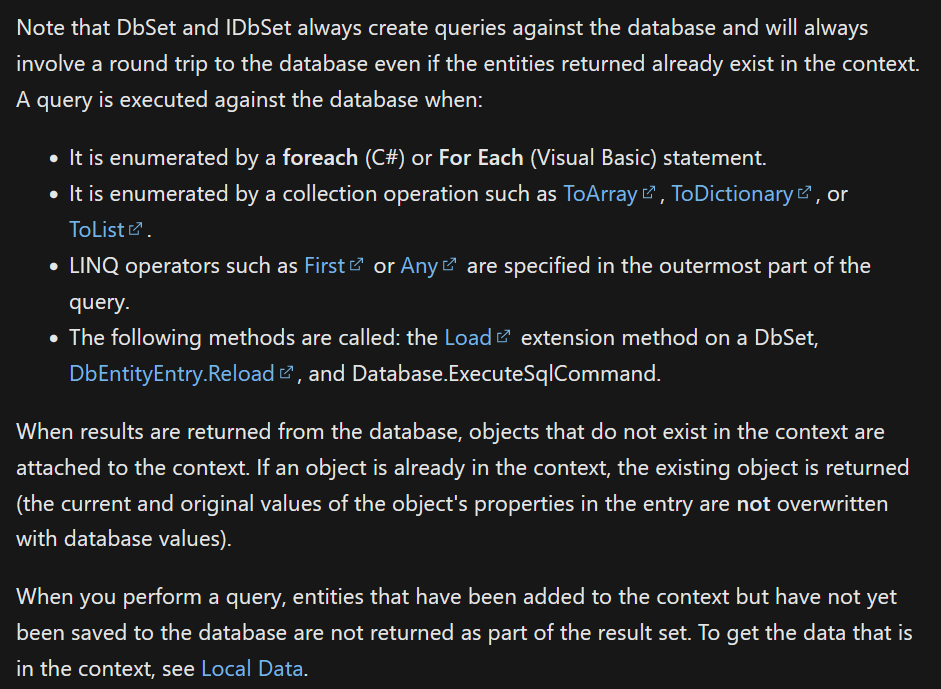
what if the order was modified in the database between saving it and getting it back?
the change tracker tracks local modifications, it's not a caching layer per se
I read somewhere that reading an entity that already exists in the changetracker, returns the entity from the changetracker
that is especially true with the "Include"
there's a pitfall too
Seems like it only works with
Find
using linq operators will always do a db roundtrip
even with or without includeyes, i wouldn't want it to work any other way
it works like that on include though
thats strange
if you query something and include orderby
then query it again and do the same thing
the include will not work
define "not work"
literally, it will ignore it
it's in the ms documentation
https://learn.microsoft.com/en-us/ef/core/querying/related-data/eager#filtered-include
look at
Caution
i have never used a filtered include
yeah I accidently did it... and then I assumed tracked entities are always returned
Stay away from it
yeah that syntax is wrong if you're just trying to get orders
nvm, read wrong
syntax is still wrong either way
Yep, in my case when i did a filtered include
i was trying just to orderby the collection
but it didn't work
and it was this " bug "
so I assumed changetracker always returns the tracked entities
seems like not the case unless you're using
Find(key)
this seems to always return the tracked entity if found
anything else just does a db roundtripWas this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.