are my getters and setters correct?
Before I work on the rest of my code, please let me know if there are any errors in my getters and setters
using System;
using System.IO;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
public class Menu
{
private string dishName;
private int dishType, dishPrice;
//def constructor
class Menu() { }
//const with param
public Menu(String dishName, int dishType, int dishPrice)
{
SetName(dishName);
SetType(dishType);
SetPrice(dishType);
}
//GETTERS
/ Returns the name of the dish.
@return: dish name
*/
public void GetName() { return dishName; }
/ Returns the type of dish ordered.
@return: returns either appetizer, entree, or dessert
*/
public int GetType() { return dishType; }
/ Returns the height of the rectangle.
@return: height of the rectangle
*/
public interface GetPrice() { returns dishPrice; }
//SETTERS
/ Updates name of dish.
@param width: the updated dish name
*/
public void SetName(string dishName) { this.Name = dishName; }
/ Updates the type of dish.
@param width: the updated dish type
*/
public void SetType(string dishType) { this.Type = dishType; }
/ Updates the type of dish.
@param width: the updated dish type
*/
public void SetPrice(string dishPrice) { this.Price = dishPrice; }
public override string ToString() { return string.Format(dishName + "(" + dishPrice + ")", GetName(), GetPrice()); }
}
173 Replies
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/They're correct in that they will work
But... they're useless
C# has properties for that
It ain't Java
yeah you'd never see this in normal C# code
you'd just use autoproperties
The "constructor" is also uh... Not a constructor.
I don't think this even compiled
looks like it should, the formatting is just messed up
GetName is void and returns a string.
oh i see
This does not compile.
my eyes must automatically block out impossible code
Not with that attitude.
(Also,
returns
does not work as a substitute for return
)this strikes me as (invalid) java code written by someone who learned C at uni
This strikes me as a wtf.
interface
😄
@Dinny please re-join us, we need feedback on our feedbackseems like the correct course of action is "actually test your code and make sure it compiles before asking for advice on good practices"
I think the getters and setters should be the least of his concern.
apologies, i didn’t see earlier messages
allow me a moment to read up
i have two separate files
this one was getting all the constructors, getters, setters, and the override to string and stuff
the second file calls upon this one to actually do thing
okay, but this file doesn't compile
it's not valid C#
well that’s the assignment anyways
did you try it before asking for help?
well yes
that’s
your IDE should be screaming at you
why i’m here
it’s not
that’s why i’m here
what editor are you using?
it’s saying no syntax errors no warnings or anything
i’m using visual studios
either way the compiler will output errors if you try to run the code
it’s not outputting anything
i’m trying to put things in an output file
do you have a project?
okay, so let's start from the very beginning
yes, what pob asked
yes
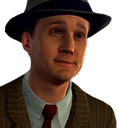
so you have a .sln, .csproj, and one or more .cs files?
.cs
i have two of those
that's not a project
oh
ugh this shii is so hard
i’m gonna throw up
just go into VS's file menu, click new project, and console application (i'm assuming that's where it is, i don't use VS)
C# applications are based on projects, you can't run loose .cs files
i don’t have that option on VS :/
one second
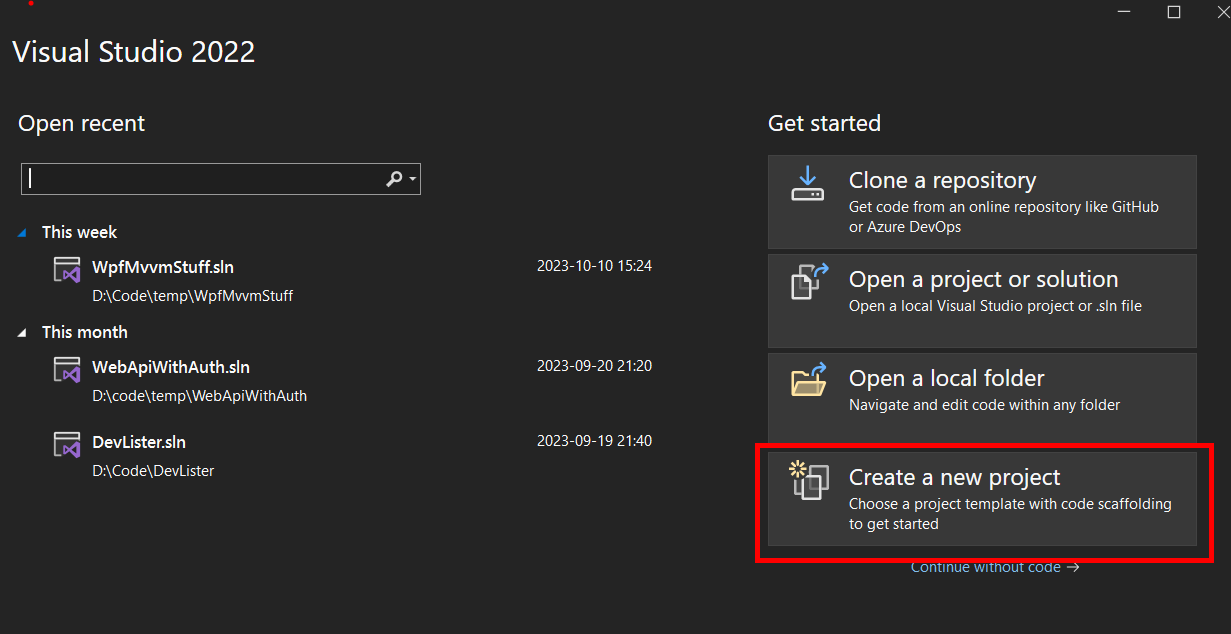
i went there
i had to go all the way out
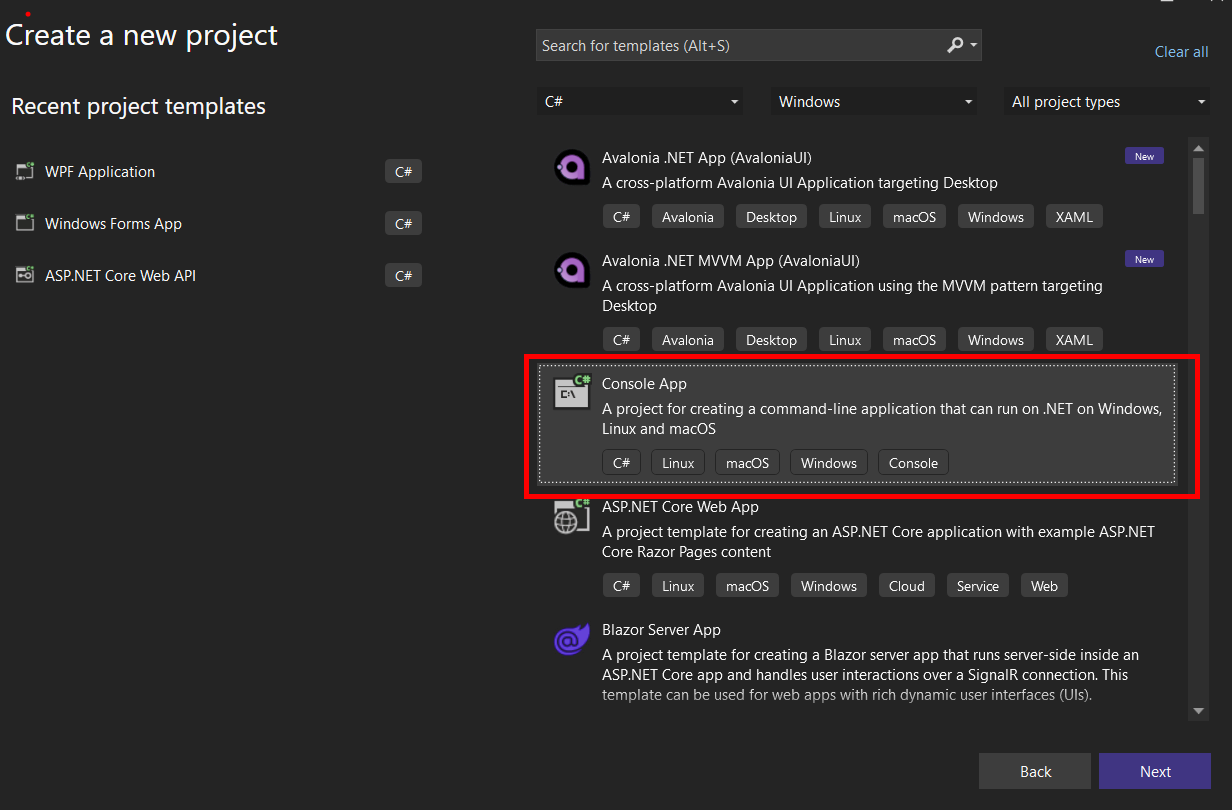
yes i’m using that
okay
Well, your first file clearly didn't
didn’t what
have a project file
i’m not sure how tho
i went through the same steps
C# and .NET is entirely project based. You never open a folder or a file directly
for both files
you open the project file
can you send a screenshot of your solution explorer window?
with your project open
one moment
sorry the picture would not load for some reason
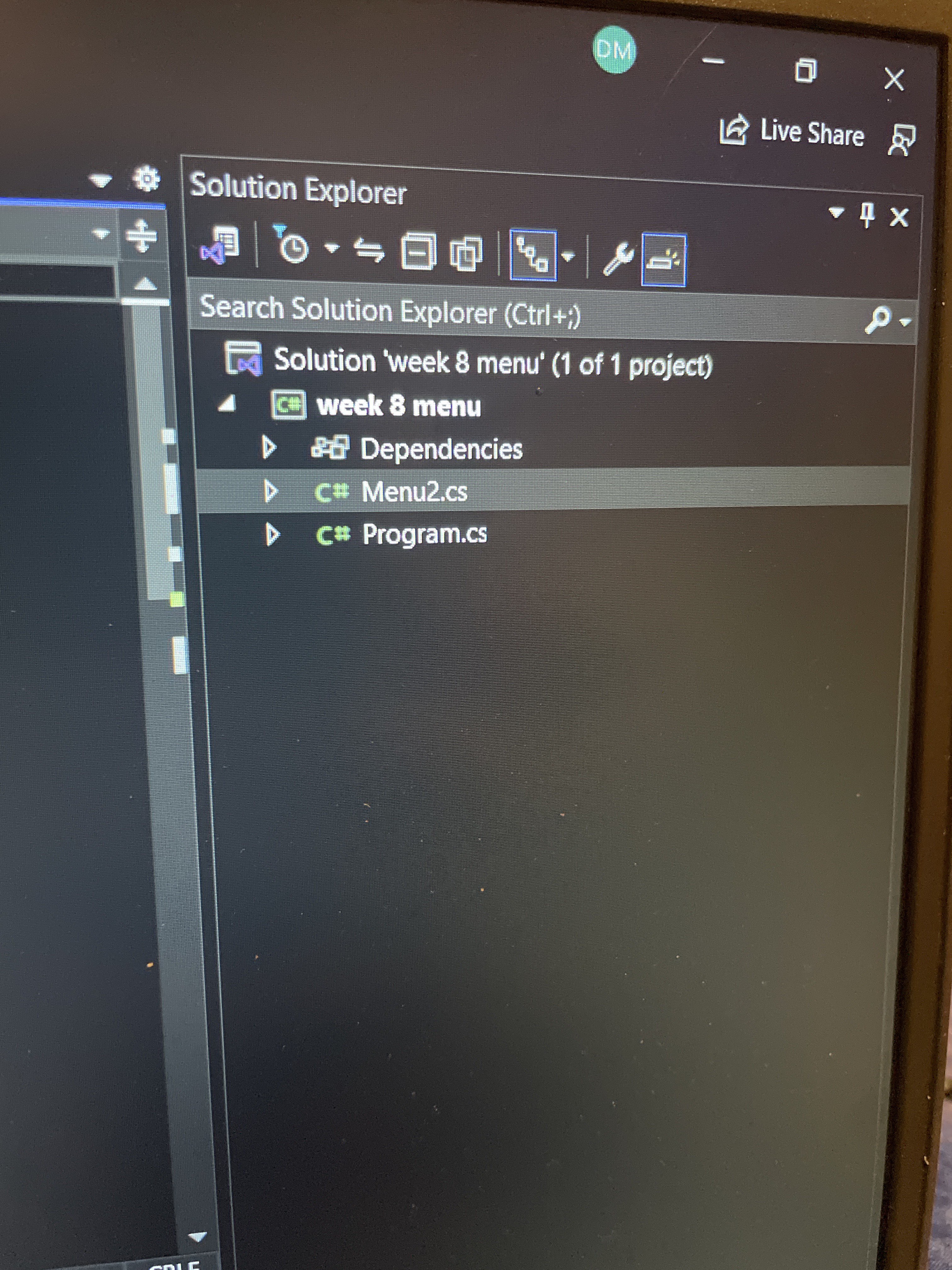
it’s working now tho
your program or the picture?
the picture
okay, so what happens if you click the run button in the toolbar at the top of VS
ok now i’m getting errors
i added in the stream reader stuff since i last posted too tho idk if that’s contributing to it
right now i'm focused on making sure your IDE is working correctly
alright
you should be seeing some red squiggles in your code and errors listed in the problems window at the bottom, is that what's happening?
yes
alright, seems like it's fine now
i see a couple green squiggles as well
those are just suggestions, they won't stop your code from compiling
the red ones are what need to be fixed
alright
once you've fixed the reds, you might want to take a look at the greens too thou 🙂 but red takes priority
ty i will keep that in mind as well
omg there’s so much red now
yup!
Lets just say that just that snippet of "getters and setters" contained quite a bit of literal nonsense code
using keywords where they dont belong, and typos in keywords etc
i was following my profs example which is why it looks this way
but i’m sure where i went wrong bc our code is quite similar
professors are teaching people to write get/set methods in C#? 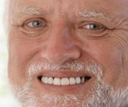
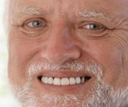
he’s honestly teaching way too much at once
like this is a beginner class, i should not be struggling this hard
i was doing fine in python and c++
my point is what they're teaching you isn't how you should be doing it
😨😨😨
but that tends to happen in CS classes
😭😭
we’re u taught differently
were *
i'm 99% self taught
😨
then how did u teach urself set and get bc clearly im doing it wrong :/
dropped out of college when i got a job offer
oh wow congrats
i picked it up at some point
same story for me. 99% self taught.
dang
that’s p cool
i feel if i had a different prof i wouldn’t be struggling this hard
anyway, the way you should be doing this is with properties, auto-properties specifically https://learn.microsoft.com/en-us/dotnet/csharp/programming-guide/classes-and-structs/properties
Properties - C# Programming Guide - C#
A property in C# is a member that uses accessor methods to read, write, or compute the value of a private field as if it were a public data member.
like i’m doing great in my other coding classes
the thing you are doing wrong with getset is that C# already has it built in with something called "properties"
if that’s the case, idky he’s teaching it this way
but your weird usage of
class
, interface
, void
and return
was the problem thou
thats why most of us went "wtf"i’m going wtf
😭
the code wasnt just conceptually wrong, it was syntactically wrong too
as in, this is so wrong the compiler will not even let it run
his lectures and way of explaining gives me a stroke. i end up having to watch youtube videos after class
ugh
it might help if you try to explain to us why you used some of those keywords in the places you did
i have literally no hope for this class this shouldn’t be that hard
because my prof did
i'm skeptical
one moment
i mean, i've had professors give me code that won't work right before but not this bad
this is part of his sample code
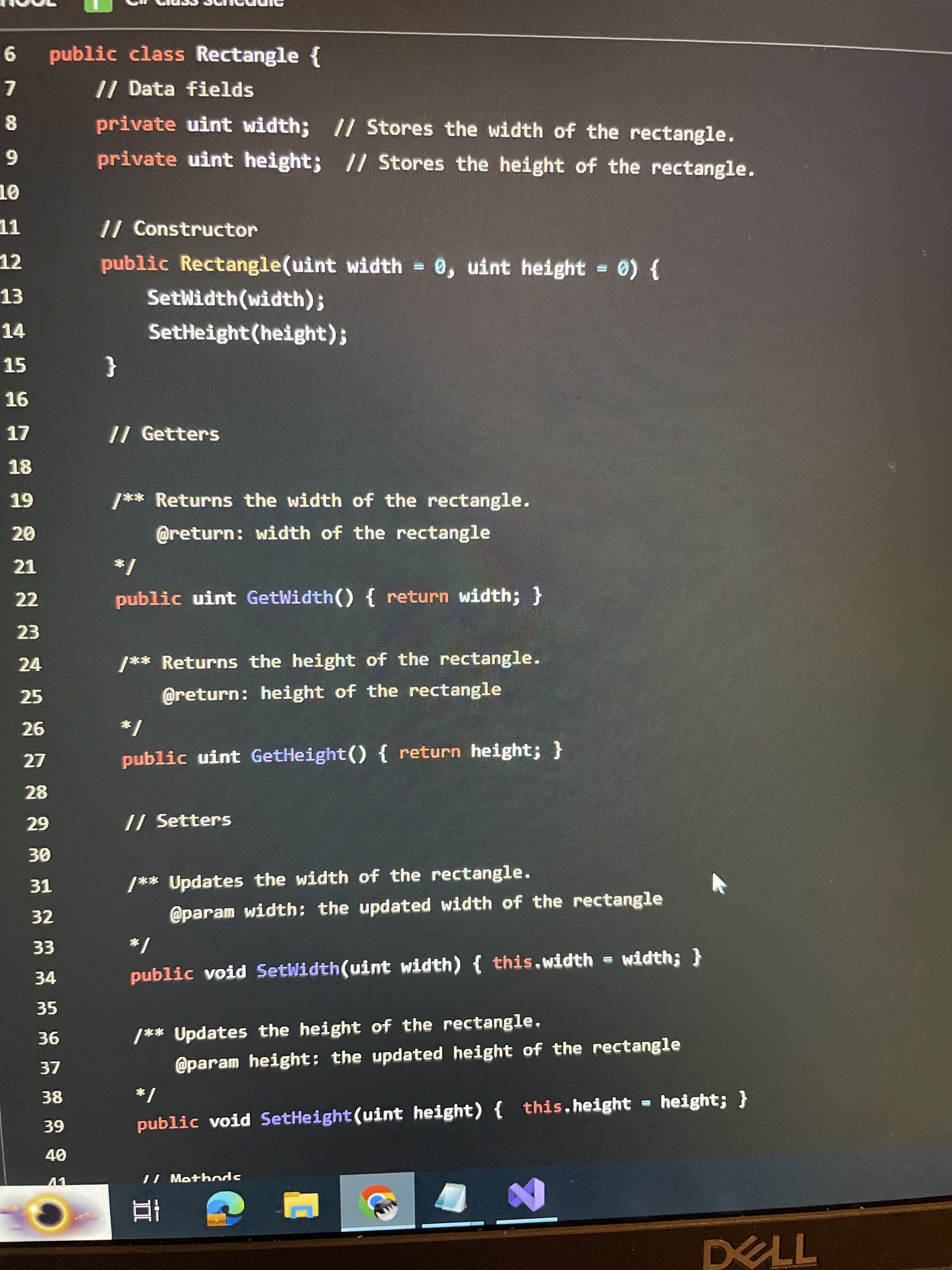
he’s doing a rectangle and i’m reading items from a menu
i had to tweak some things tho
yes, so your professor did not do what you're doing wrong
sigh
Still goofy code though.
yeah, but just "weird practices" compared to "literal nonsense"
look at how your professor is defining the get and set methods and try to find the problems with yours
Tell your proffessor to stop using C# 2.0
😭
Alright one moment
ok so i got rid of most of the wrrors
but i’m unsure got to fix this o e
$codegif
post your updated code using a code block, like in this gif
wait how do i put my code in that gif
i’ve never done that before
just type the characters
then paste your code
like i type $code gif and then late my code ?
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/oh
lmao alr one moment
i didnt mean to hit enter
i have squiggles under dishPrice right after it says SetPrice
oh wait'hang on
You also goofed up the codeblock
It seems to me you need to pay more attention to details
i figured it out
im trying dawg
i am still new at this lol
but i got rid of the red squiggles finally
Yeah but look
looking
You had both a gif literally showing you how to do it, and the verbatim text to write to make a block
but you still decided to put
cs
on a new line instead of directly after the tripple backticki clicked the wrong character and i accidentally hit enter
i dont think thats a crime
then you fix it?
discord supports editing
:////
ok the embed is there tho now
yeah but if you'd fix it, you'd see why we want it done properly
i did tho
nope
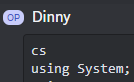
im getting drilled into bc i left a cs in there or is it somehting else
i was just following the gif
no you are not
look at the gif again
look where it places the
cs
look where you placed it
these are not the same
you've done python, so you should know what significant whitespace isi was told that that didn't matter in c# tho and in c++ well unless we were working with input an doutput files
and*
but this is discord, not C#
okay dawg
i will just fix it
please do
There we go.
is this better
ok cool
Do you see how much easier that is to read?
yes i do
👍
but i had got rid of the squiggles now so i think i am good
i just need to work on the errors in my input an doutput file now
Okay, now with that fixed.. Your getters and setters look good, ignoring the fact that they should be properties
this is how you'd write this code in Java
if my prof taught me to do it the way u mentioned i def would have, otherwise he has not shown us that yet
he tends to be more difficult than need be honestly
Yeah, not much you can do about that. Follow the profs way for now
just know that this isnt how modern C# does it
idk why he isn't teaching us the modern way then
that's so strange
and he's been using c# fo ryears too
by modern, I mean since 2003 btw :p
given that info, idk why he's teaching it the way he is if the way u mentioned is how most people do it
but if its's right for now, i'll stick to it
hopefully he will teach an updated version as class goes on
this is how this would look written with properties
wth
that looks so much cleaner
mhm
and nicer to look at
thats why properties were invented 🙂
bro my prof is so extra
this looks way easier
wanna know another thing?
yes go for it
the way he has you comment your code
thats not how we do that either
😭
this is so aggy
how do u comment ur code
when commenting a class, method, constructor, property etc, we use
///
, aka summary comments
and our tooling supports theselook at this:
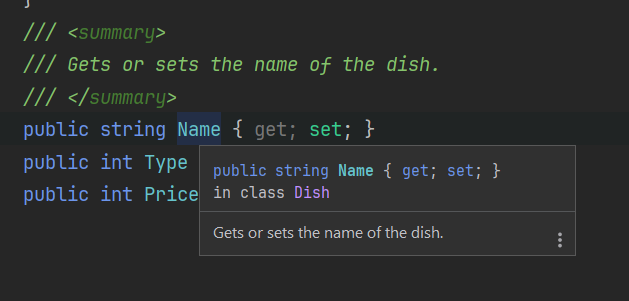
when I hover my cursor over the property, I get to see the comment I wrote
this is true even when accessing it from another class etc
its how the built in documentation works too
he always makes us use docstring and he said he will take off a lot of points if we fail to use them
://///
this all is so backwards for me
i truly don't understand why he;s teaching it this way
probably out of touch with current standards
I'm sticking to my original opinion that your prof is an old-school C developer who knows Java
///
are docblocks in C#
/**
is, what, PHP?i believe so bc i am pretty sure he teaches java at our college too
C, C++ as well.
he has also been at that college for years so i don't doubt it
tho i would have chosen a different prof but he is the only one that teaches c# at my college
Just follow his crazy ideas for now
but be aware that he is many many years out of date
when i switch to a 4 year uni, i feel i'd learn what u guys are telling me
it's gonna be so jarring
well thank you guys anyways, i truly appreciate u help and patience.
not really
its mostly style and missing properties
I will have to keep in mind or next time that my prof teaching style is out of datr
the core logic will be the same
the thinking will be the same
yeah i agree
those are the important parts of programming
but it was still shocking to see
syntax is easy to re-learn 🙂
i have faith, I'll just have to keep studying and what not
thank you for your input i truly appreciat eit
it***
good luck!
thank u 😄