children.forEach typescript problem
I have this problem in the forEach with typescript.
Property 'forEach' does not exist on type 'ResolvedChildren'.
Property 'forEach' does not exist on type 'number'.ts(2339)
12 Replies
Wouldn't it be better to add a class name to the parent, then use CSS to select every :nth-child(even) and apply the background color there?
Children can be a lot of things, including those that do not have a forEach method
If that doesn't work for you, there's
children(...).toArray()
. However, you still need to filter its elements for DOM nodes.
This just wraps children into an array that aren't already one.
I'd still recommend the CSS way.Why not use something like
<For each={items}>{(item, i) => <div class={cx(i() % 2 && "my-whitesmoke-class", ...otherClasses)}>foo</div></For>
You can get cx
from https://cva.style/docs/api-referenceor you use
classList={{'even' : i() % 2 == 0 }}
to make this even simpler.
But even so, why add classes if you can already select the nodes in question?Better to stay away from
classList
, Ryan seems to think that adding this feature wasn't the best idea and may be removed in a future version of Solid https://www.youtube.com/watch?v=87D15Gu1d6w&t=7605sRyan Carniato
YouTube
Building SolidJS v1.8
The upcoming SolidJS v1.8 release might not be the most flashy but it has us re-examining our foundations on the server. Join me as I talk through the thinking and features that go into the latest Solid release.
[0:00] Preamble
[11:00] Introducing Solid 1.8 - Overview
[22:15] Solid 1.8 - De-duping Streaming Serialization
[37:15] Solid 1.8 - Nes...
Ah, forgot that. In any case, don't use a class unless you need it. A CSS selector is fine in this case.
I'll try the classes, I was doing it with the style because it's something really simple the even childs only need to have background gray but maybe stay away with the style method.
I'll try that too, typescript is so tricky sometimes..
I don't think that is about my lack of knowledge(of course i have a lot to learn) that typescript is tricky sometimes, I think it overcomplicates the code, and sometimes it is not that necesary.
Also the types are not really types for what i read here and there.. I'm not against typescript, but sometimes it is tricky I think.
https://medium.com/@iamchrisgrounds/the-problem-with-typescripts-types-1ee92ba7ec39
@Toka Yep, the article is "intense" but well for me at least it's strange the behaviour described in there.
And then I'm calling like this:
which works fine but i had to type the children as any[] for some reason it didn't work otherways.
I'll look into cx() some day if i have time.
I'll change the css classes for more correct ones too
dude ..
I'll try to make constructive criticism, about your criticism xD
instead of saying it's wrong, better point what you thing is wrong
I don't know if it is how i read you but you seem rude
okok
what i try to accomplish is basically the best way to do this:
To compose big components of more atomic ones when calling them. And in this component DescriptionList I wanted to compartmentalize the jsx too. So in the case I wanted to use the Row in another component i could. I hope that makes sense
In ssr i don't know how this would be done, but I'm using csr
I'm coming from react so I'm used to do things in a certain way. But ofc react has virtual DOM
yep agreed haha
But you are not using jsx then no? idk I liked the jsx way
in the Rows I mean
yep yep
I only don't like to call Row as a function. It's a preference of aesthetics hahah more than anything else
but if some day i need to do ssr maybe i go with that
didn't work as expected, I thing cause there are children of children
looking libraries there's a suid component library (MUI port for solidjs) that makes things the way I like (maybe a bit too much) but should be possible to be done in solidjs
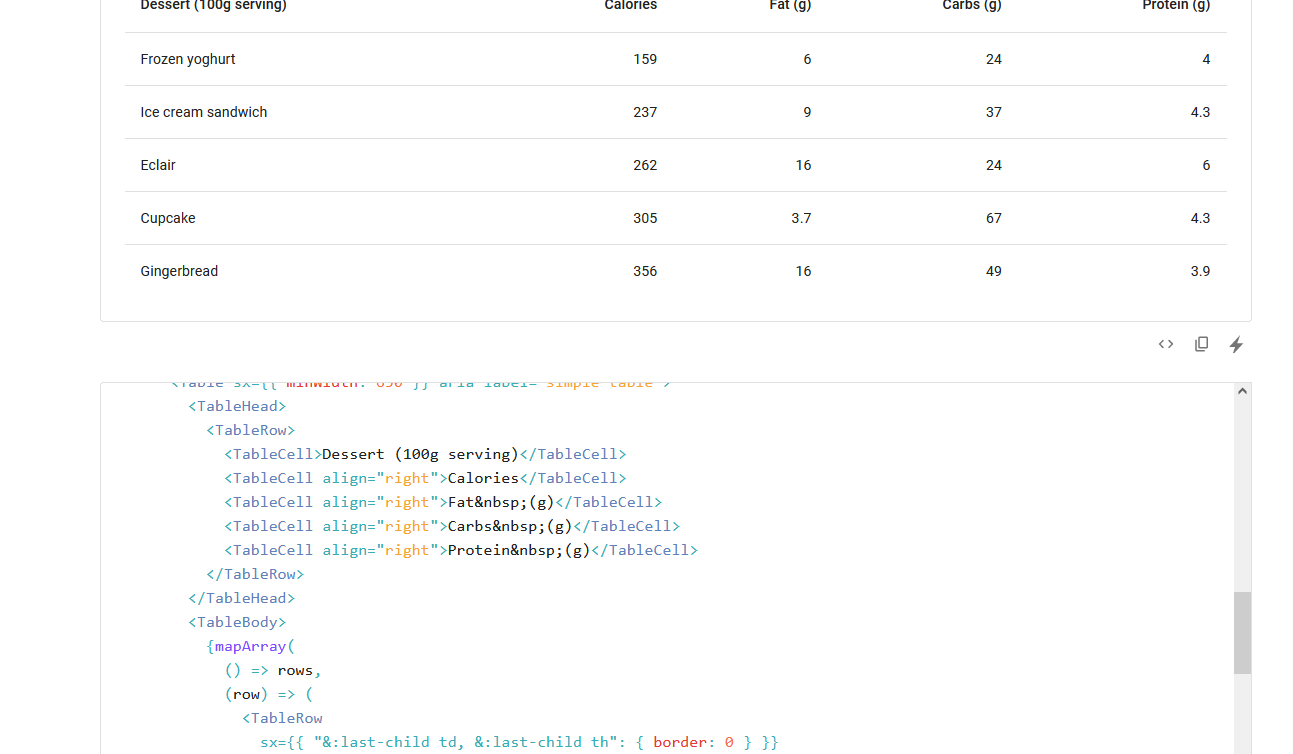
a bit ugly that sx tho xd
and the mapArray too
but well at least is really readable and organized
sry i forgot haha
This I thing is really beautiful
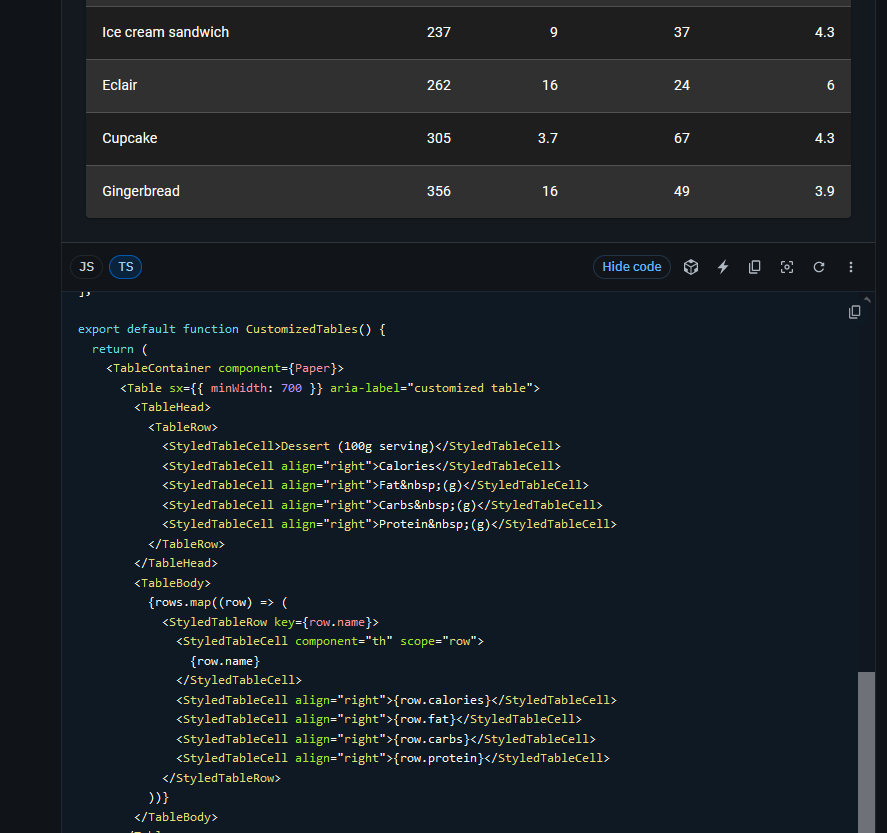
it's a more generic list what I'm trying to do but something similar to that
inputs?
ah yeah
It's very well ported really
a component library but can't say much more xd
yep
Thank you!!!