❔ Connection Timeout argument passed doesn't work for a SqlConnection
I have the following connection string:
I have a WPF with a control calling a MessageBox to show whether the connection to the database is established or not and I want Connection.Open() to terminate early instead of the user having to wait 15 seconds for the failed connection message to be displayed, but Connection Timeout=2 seems to do nothing.
An open connection gives an instantaneous response, while the failed connection despite the argument, loads with the average time no matter what the timeout is set to.
This is the code for the sqlconnection call:
57 Replies
confirm the value of
connectionString
with the debuggerI have done so

oh
the space
I tried setting the connectionString explicitly now through a method call without using the configurationmanager still doesn't work
Which one?
"Connection Timeout"
Yeah it should set the timeout value I am using it in a WPF with a messagebox
right
And I want a quick response to the message box instead of the user having to wait 15 secs for a failed connection
you said that
remove the space
Tried already, doesn't work
Ill try again
Yep same result
try building it manually with SqlConnectionStringBuilder
Check this out - apparently its readonly?
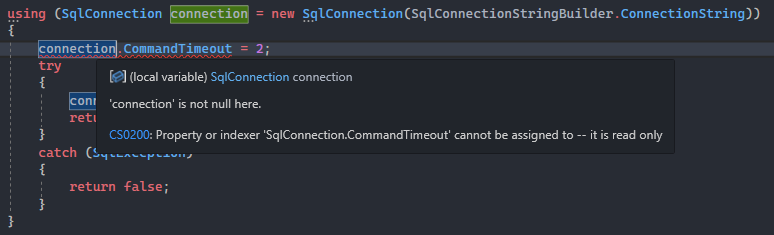
Doesn't really makes sense in my head
uh
yeah
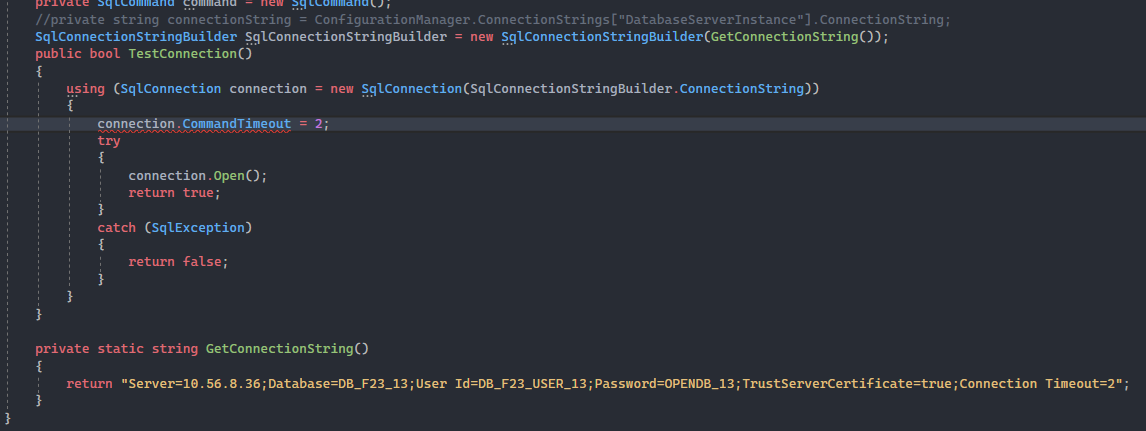
it's not mutable on the connection
Even though I am setting it in the ConnectionStringBuilder it still doesnt seem to work
use SqlConnectionStringBuilder
Tried so - it still buggers
buggers how?
The wait time is still 30 secs
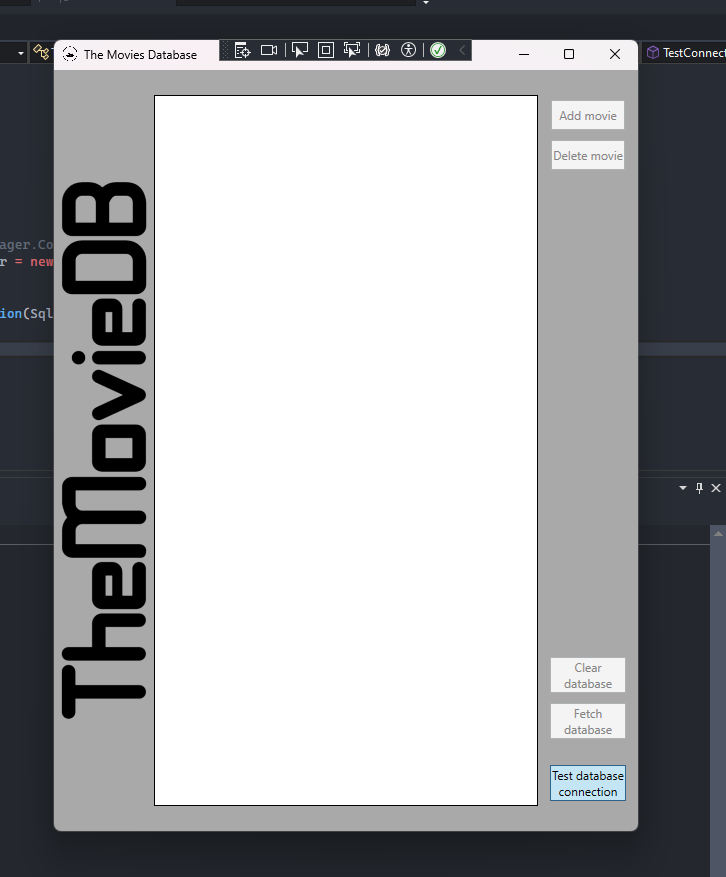
wait for what?
For the connection timeout
Check it out even the debugger confirms both the timeout for the command and connection is 2 secs and yet the wait time still goes for the default value
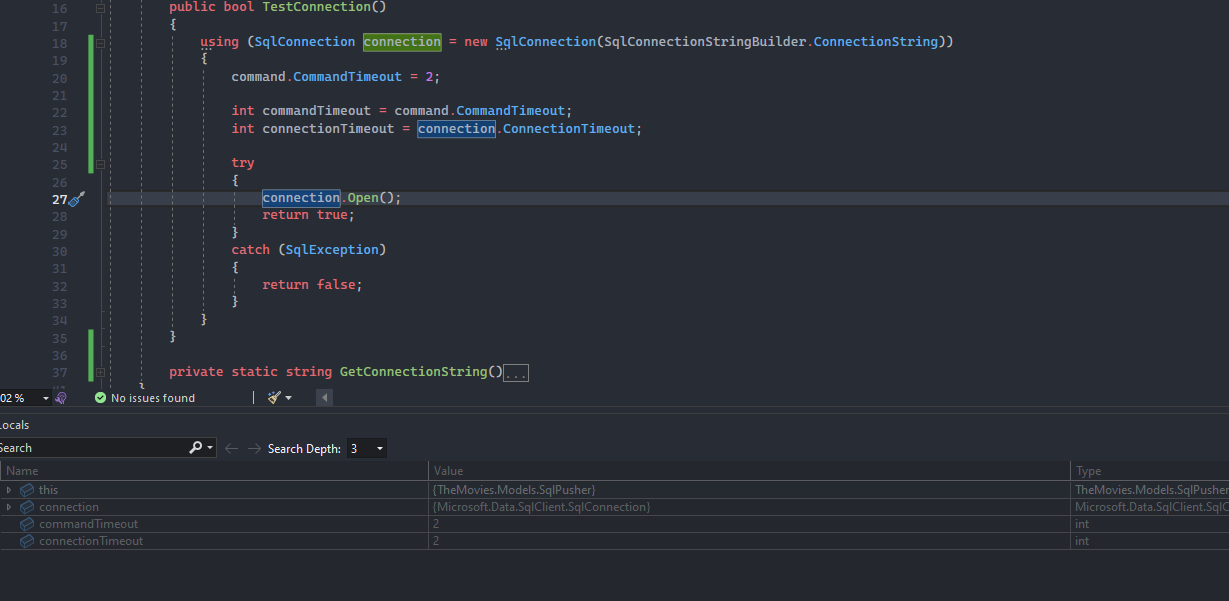
so, the connection likely isn't the problem
define "wait time"
For the command or connection?
neither?
the wait time still goes for the default valuewhat is "wait time"? how are you measuring that?
Well either it's CommandTimeout or ConnectionTimeout
But we have confirmed these by the debugger to be 2 seconds, so it doesn't make sense what could be causing the issue
assumptions based on nothing
Well I can't seem to narrow down the issue in any other way
I have no idea what could be causing the problem
what issue?
The issue I am experiencing with the wait time
Or rather, the issue the user will be experiencing
Perhaps I could ping the database and return a boolean value? Dose it make sense to use the Open.Connection to test whether a connection can be established?
the issue the user will be experiencingwhich is what, specifically?
Waiting 30 seconds on a stale screen with the WPF for the messagebox to be returned stating the user could not connect to the database
great
what triggers this messagebox?
The try catch block
what try catch block?
from your initial message? I don't see a messagebox anywhere in there
I'll have to screenshot the WPF XAML and my ViewModel class
But basically the try catch catches any sqlexceptions which prompts the messagebox
And the wait time is long due to the connection.Open() trying to load for 30 secs instead of the defined time
Ill try something else
ConnectionState Enum (System.Data)
Describes the current state of the connection to a data source.
what exception are you getting?
Basing it on ConnectionState instead to see if it speeds up things as the value is based on an enum instead of trying to establish a connection
Doesnt matter I am catching any sql exception - if my VPN is not toggled on it fails as it should
nonsense
look at the exception
you're trying to determine why an exception is occurring, but the exception doesn't matter?
nothing could be more meaningful
I fixed it by doing this
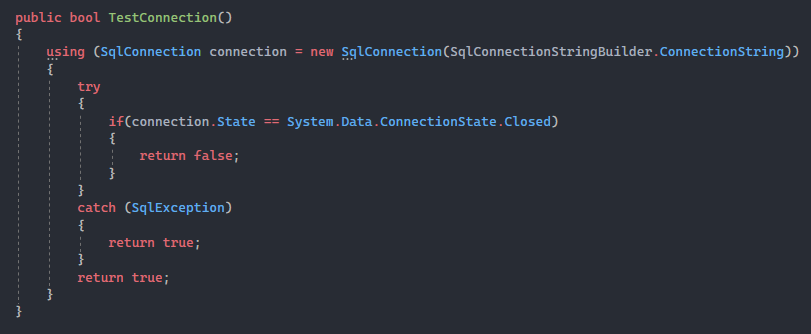
that fixes nothing
look at the exception
It fixed it
The messagebox is instantenous now
Or wait
look
at
the
exception
You're right - it fixed nothing lol
How do I see it? In the debugger autos window?
excpetions should be pausing the debugger the moment they throw
ensure that all excpetions are selected for first-chance catching, withing the "Exception Settings" widget
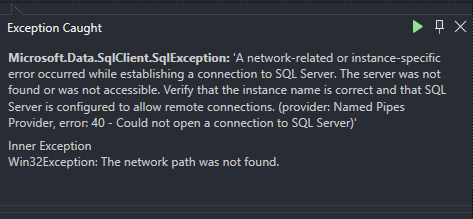
so, you said you're currently running on a VPN?
The WPF is developed for usage with a VPN
I am testing the functionality of the feature by trying to turning the VPN off and testing the database conection
The program is working as intended but I want the prompted messagebox to appear faster by decreasing the connection timeout
see what happens if you ditch the VPN, and just specify a bogus connection
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.