✅ Help with Homework
I have an exercise for homework in order to practice.
I have completed it to the extent of my abilities, however, one of the 10 run tests in the online platform does not provide the required output.
Any ideas? :/
20 Replies
(Some on the CW information is probably not correct, my only idea is that it has something to do with the math.pow logic. However, it seems to work fine for the ther 9 tests :/)
Do you know what values does the non-passing test use?
that's the issue, I don't
I think I need alternative
to double
the value might be bigger
than double can store
Angius
REPL Result: Success
Result: double
Compile: 99.280ms | Execution: 11.396ms | React with ❌ to remove this embed.
I doubt it
tried decimal as well
but math.pow can't do it with decimal
and when I did (decimal)Math.Pow I get runtime error 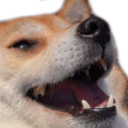
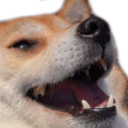
I wonder if it goes over the alloted execution time...?
it doesn't
Try calculating the value just once instead of twice
it gives a specific error if it does
Huh
on the website
that I submit the code to
so it's not the time that's an issue here 😦
Yeah, no idea, everything seems perfectly fine
Well
@ZZZZZZZZZZZZZZZZZZZZZZZZZ apparently adding using System.Numerics; and making the value BigInteger instead of double and doing BigInteger.Pow fixed it.
Only issue is i don't know why
I don't think we have studied that 😐
Huh
I wonder if
ulong
would've worked
Actually, I wonder if rounding the value and casting it to an int
or uint
would've workeddoesn't
tested now
Perhaps, because of floating point rounding errors, the value sometimes is
813223.000000000032
or some suchi have no idea at this point...
Anyway
Thank you very much for your 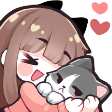
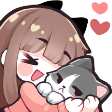
Anytime 

Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.