❔ ✅ :white_check_mark: Trying to have a program read a word in a string and add to a counter
I have to make a program that reads the different letters, consonants and some words as repeated through out the text. I have been able to do it for vowels and consonants, but I do not know what to do for complete specific words in the text.
310 Replies
Can you provide more context?
Like what you're trying, what you're expected to output
Yes, basically I have to make a program that reads how many times a letter or a word is repeted in a text, a.k.a. a string. My issue is that I dont know how to make it read a full word such as "Hello".
So far it counts letter by letter and adds +1 every repetition.
Easiest way would be splitting the string on whitespace
That way you get an array of individual words
Thats another issue, it has to be done with tools used in class and that is something we have not seen yet
Yes, it depends on what output you're expecting
I was thinking switch
So you're looking at making your own parser, basically
For instance: string = "Hello hello hello"; and the console shows: There are 3 Hello in your text.
What tools have you used in class?
is "ZZZZZ" considered two words if you're looking for ZZZ?
switch case, else if, and so on
Or even three
Not so advanced tools yet
Ah ok
That's pretty limiting. No for/foreach?
For each too, while as well
For
Some arrays
Lol
Let me share a screenshot of my work so far
Angius
REPL Result: Success
Result: List<string>
Compile: 635.947ms | Execution: 51.530ms | React with ❌ to remove this embed.
There
A very manual way to split a string into words
But we have not seen things like IsWhiteSpace
Ah, well, RIP
You can try just checking
== ' '
Okay but maybe we can explain what in the world is going on
It will work only with spaces, tho
Exactly my issue
You have an input string, right?
Instead of spoon-feeding you the solution let's arrive at it
okay
yes
Yes, so you have an input string and you want to count the words?
Yes
So "for each" word you have to do something
Like the repetition of certain words
Yes
And if you're looking for certain words you have to save them somehow
Yes?
Do you know how to declare variables?
This is an example of what I did to count the consonants
else if (char.Parse(letter.ToUpper()) == 65 char.Parse(letter.ToUpper()) == 69
char.Parse(letter.ToUpper()) == 73 char.Parse(letter.ToUpper()) == 79
char.Parse(letter.ToUpper()) == 85)
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/Using ASCII table values
Why use ASCII values?
And not just
theChar == 'g'
They didnt teach us that
They didn't teach you what?
It's a "trick"
theChar
You have comparison in your code,
==
You are using chars
Everything seems to be thereFair, but then my issue remains for words
'g' and 103 have the same value
Well,
'g'
and (char)103
do
Or (int)'g'
and 103
Words are similar
You can iterate over your string and check
How do you do that?
So if you have a string separated by regular spaces you can just write a for each-loop
foreach (string word in yourLargeString.Split(' '))
But for instance I have never used Split
hm okay, do you need to do it without that?
Most probably yes
In that case you need to iterate over each character and check if a character is space
char == ' '
and separate words that way
Like in my example, yeah
But why a space?
space as a word separator
What delimites words in a sentence?
But is that going to count every word in the text?
Depends how you implement it
you could count how many spaces you encounter
I have counted the spaces with char == 32
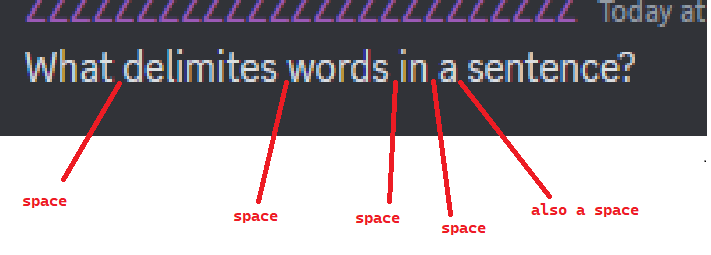
Why not
char = ' '
?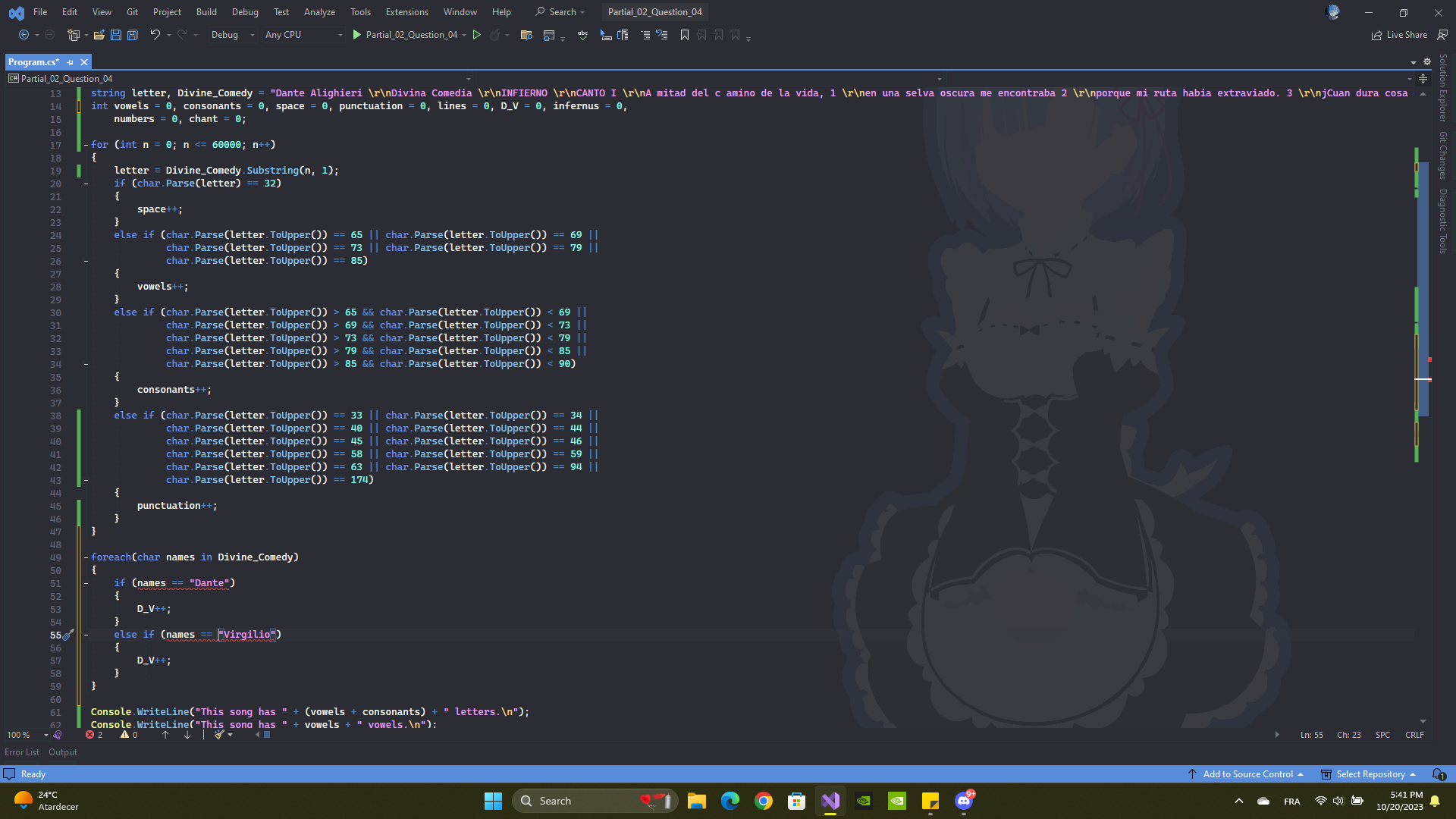
Finally got it
The word I need to count is "Dante", which is inside the string
Wait, you want to only count the occurences of
Dante
?Dante, Virgilio, chant and infernus
Ah
Those four basically
Well, you would still run a parser in a loop, then
I... think that's what you're doing
Yes
All that useless code kinda obstructs what's happening, and I can't remember what ASCII value each char has lol
Sorry
Nothing to be sorry for, we all started by writing bad code
It works to count letter, but not a whole world
But you really could use to replace all those
char.Parse(letter.ToUpper()) == 65
with just letter == 'v'
etcBit would I have to repeat that for each letter?
The way you're doing it right now, yes
But with much less code
And the ability to actually, you know, see the letter
Who tf can remember what letter
65
isI was about to say, this counts the letters in Divine_Comedy
Yes
But I'm looking for a way to get the word Dante and count it
Okay, so use .split
Can't
Because teachers are stupid
The thing we didn't use Split in class
Yea
So
Loop over all the letters
.
If you encounter
' '
that's a new wordWhat in the world
Yeah
If you encounter
'd'
you migh't be at Dante
, so keep going at it
See if the next one is a
, then n
, etcYes, but it has to count how many times the word Dante appeats
If it's not
d
, then maybe it's v
In that case, start looking for letters in vergil
If you find all letters of a given word
Increment a variableBut what about Dante and Dog? That would ass two
Oh I get what you mean, nvm
Yeah, so if the next letter after
d
is a
, see if the next ones are nte
With a loop?
If you have
o
after d
, well, that's no longer Dante
Yeah
A for
loop maybe even
So you can look ahead
If string[i]
is d
, then check if string[i+1]
is a
, string[i+2]
is n
, etcFor Divine_Comedy = D
then A?
I'm so sorry, it's very stupid to not use the built-in functions to accomplish what you're doing. Why are they teaching you this way?
It's very convoluted
I don't know, this man is truly stupid
They're teaching the 1997 way, which is about when the teacher probably stopped learning
It's all too common
So an if for the string and it keeps checking?
Yes, you would certainly use an
if
Could use a substring instead of checking individual letters, if that's something you usedWe did
That'll make things easier, then
if string.Substring == "D"
When you encounter a space at index
i
, check if the substring at i + 1
with length 5
is equal to dante
That means you got the word
Boom
If not, check if the substring at i + 1
of length 6
is vergil
EtcSo when my n = space then I try the substring?
ye
That would result in an
IndexOutOfRangeException
when the string ends thoughIt could, true
So make sure that
i + 1 + 6
for, say, vergil
doesn't exceed string lengthThe leght is like 60000
Don't use 60000, use
string.Length
insteadYou can still run into out of range exception if the last word is
cat
and you're trying to get a substring of length 6In my case the last word has nothing to do with the ones i am cpunting
But you will still be checking it
If it's
dog
You would still check substring of length 5
for dante
And a substring of length 6
for vergil
And You can't, because it's only 3 characters
So, index out of rangeBut its like a 9 letter word
Sigh
Okay, sure
Don't check for it, then
You could check if, for example,
i + 5
does not exceed string.Length
But know that the code will break if the last word is less than 5 letters
Sorry, I am trying my best to understant
I have something like this
for (int n = 0; n <= 60000; n++)
{
letter = Divine_Comedy.Substring(n, 1);
if (char.Parse(letter) == 32)
{
Divine_Comedy.Substring(n, letter.Length + 1);
}
}
uh
You can get the char with just
divineComedy[n]
no need for substring there
That would mean you get a char
, too, and not a string
So you can do letter = ' '
Without the parsing and magic numbersfor (int n = 0; n <= 60000; n++)
{
letter = Divine_Comedy.Substring(n, 1);
if (letter = ' ')
{
Divine_Comedy.Substring(n, letter.Length + 1);
}
}
Like that?
Well, almost
Divine_Comedy.Substring(n, letter.Length + 1);
this does nothing
letter
will always have the length of 1
dante
has 5 letters
So, if the divineComedy[n]
is ' '
, check if divineComedy.Substring(n+1, 5) == "dante"
Preferably .ToLower()
the divineComedy
string beforehand, though
Or use .Equals("dante", StringComparison.OrdinalIgnoreCase)
to ignore the caseOh, you're allowed to use substring?
for (int n = 0; n <= 60000; n++)
{
if (Divine_Comedy.Substring(n + 1, 5) == "dante");
{
D_V++;
}
}
Like this?
Substrings yes
IndexOf?
We used Index positions
Something like that, yeah
And how do you add the ToLower, or where?
whereN
...?I meant where
To your string, ideally
var divineComedy = "the text of divine comedy...".ToLower();
You can add to the string?
Yes
Omg stupid ass teacher I have
Angius
REPL Result: Success
Result: string
Compile: 452.559ms | Execution: 36.748ms | React with ❌ to remove this embed.
I got like 6000
Then added the ToLower and now I have 0
..?
How'd you do that?
Maybe it wasnt that
I deleted it and still get 0
Then something else is fucky-wucky
for (int n = 0; n <= 60000; n++)
{
if (Divine_Comedy.Substring(n + 1, 5) == "Dante")
{
D_V++;
}
}
I have this
Well, if your string has been
.ToLower()
ed, it will... not have uppercase characters anymore
So "Dante"
will not be found
Look for "dante"
I took the ToLower out and ran it like that
Put the ToLower and ran dante, still nothing
0 names
This problem is pissing me off
Because you're not allowed to use realistic functions
It is to me too 🥲
I know
I cant figure out the issue
Post your code
In it's entirety
$paste
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
You're not checking if the current character is a whitespace here
the = ' '
Yes
Although I'd assume it should still work
for (int n = 0; n <= 60000; n++)
{
letter = Divine_Comedy.Substring(n, 1);
if (letter = ' ')
{
if (Divine_Comedy.Substring(n + 1, 5) == "dante")
{
names++;
}
}
}
Like that?
Yes, if you called
ToLower
on Divine_Comedy
I did
letter
would be a string
, wouldn't it?Cannot convert char to string on the = ' '
And you use
letter = ' '
instead of letter == ' '
Isnt that declared in the variables?
Like this
.Substring()
returns a string
' '
is a char
Square peg does not fit round holeSo letter should be declared as char?
Yes
See my example
But then the other part is marked under red
Have you used
IndexOf
?Then fix that other part
What use for indexof would there be?
Checking for the next whitespace to get start/end indices of the next word
Trying to spoiler tag it
discord is making my life hard
It sort of works without edge cases
Yeah, fair enough. Otherwise we'd find
danteian
and it'd count for dante
I am still confused
Its so stupid
@TheOne1984
I am not sure if we used that
Is it the same as the index position ?
Yes
The index of a selected character
Cant we do it this way?
Should be able to. What error(s) are you currently getting?
Cant convert char to string on the = ' '
(char)' '
or (string)' '
That's because
letter
is a string
Yes, so do I char.Parse it?
no, just typecast it
C# is a nice friend that way
You could do
== " "
, but it's better to just change letter
to char
Or use the indexer already
^
Instead of getting a substring of length 1
This will be the last time I mention it
Do what you will with this information
ZZ++ is correct
Idk that
But the rest of the code uses letter as string, even though ZZZZZZZZ suggest using =
-> (int)2.3f is typecasting to 2 (integer)
but listen to ZZ
char letter = Divine_Comedy[0];
I tried the == " " and still get 0
letters are strings
do you know of ASCII?
Yes, i used it for the other part
Well, there's no need to. You could change the code
Oh no...
I am so lost
This is not a problem that should be hard
it's stupid
Its hard because we cant use other tools
Yes, it's artificially hard for no reason
I tried solving it
put it in a spoiler up above
but I don't want to spoon-feed you the solution
I saw it, dont know many of those tools
it was only loops and indexof?
Oh true
By god I hope you can use that
It's so stupid though
I've worked with .NET and C# for 8 years
literally never had encountered this situation
"you can't use split"
He didnt even teach us Split
And I still cant do this
It's a hard problem when you can't use library functions
You have to reinvent the wheel
🥲
Do you get it to work with my algorithm?
I detest copy-paste but what the fuck
Havnt tried it, seems to advanced compared to my level
How.. how can you make it less advanced?
I mean
Not using split is making it much less advanced
for (int n = 0; n <= Divine_Comedy.Length; n++)
{
letter = Divine_Comedy.Substring(n, 1);
if (letter == " ")
{
if (Divine_Comedy.Substring(n + 1, 5) == "dante")
{
names++;
}
}
}
Like that was going somewhere
are you forced to use that?
Its the way he taught us
wait you're using substring there
Yes
wtf
That he taught us
no wait wtf
are you allowed to use substring???
yes
I stick to this until we can use sensible methods
Bug prone but the premise is weird af
this will literally never happen to you in real life
Pain...
I dont know how to solve this
Show us what you got right now
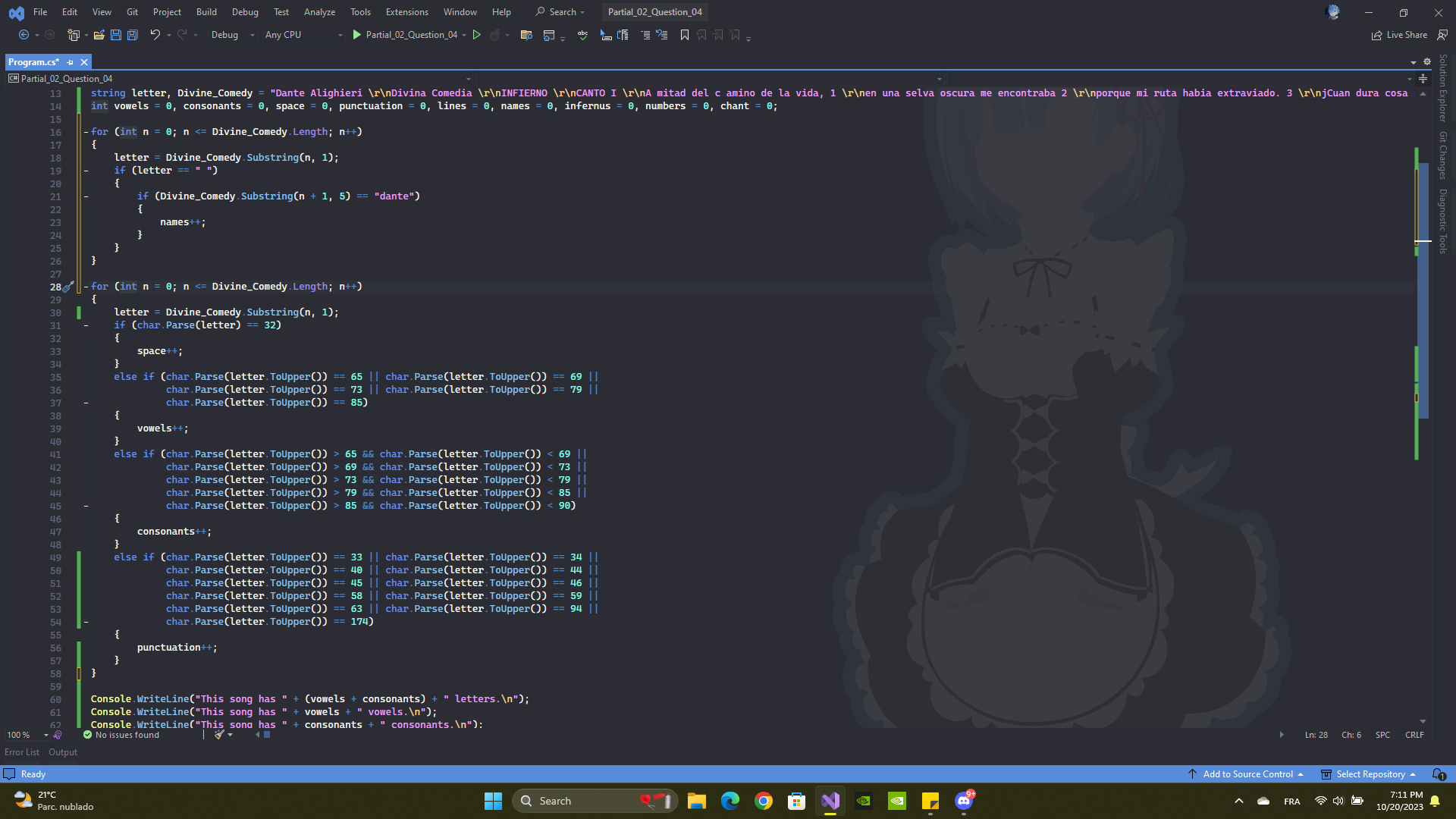
deus nobiscum sit
so
eh
your assignment is to expand the for-loop?
if I interpret this problem correctly?
My assignment is to count the Dantes
okay
the vowels, consonants and punctuations are a hint
How so?
I suppose you're supposed to have an if-clause for 'd'
I mean, you could use other methods if you consider the
string
type a toolor 'D'
like if (char.Parse(letter.ToUpper())) == 'D')
Ill do anything that solves this
If "D" then names++
Not really, use substring to follow up on "ante"
because you checked if "D" was present
or you check substring with current index (D) + "ante"
you're going to be interrupted by the consonant if-clause since D is a consonant
so you need to implement your if-clause in the consonant space
So if D, the ante, then names ++
Yeah, and D only occurs in this case
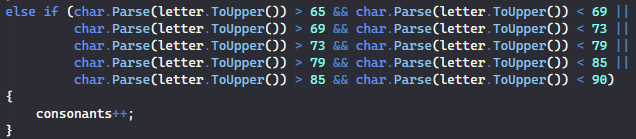
Yes
and if you're in that case you can check the substring
I think that's what your professor's getting at
So after consonants++
if (char.Parse(letter.ToUpper())) == 'D')
if ante
names++;
That's the pseudo-code, yeah
you got it my friend
just let the professor's framework do whatever the fuck he thought was good
and scalpel in the thing you apparently were supposed to
sorry for cursing
Its fine
I still cant figure this out, i get your idea
you're almost there
you just need to substring words
I get lots of errors after the consonats++
What do the errors say
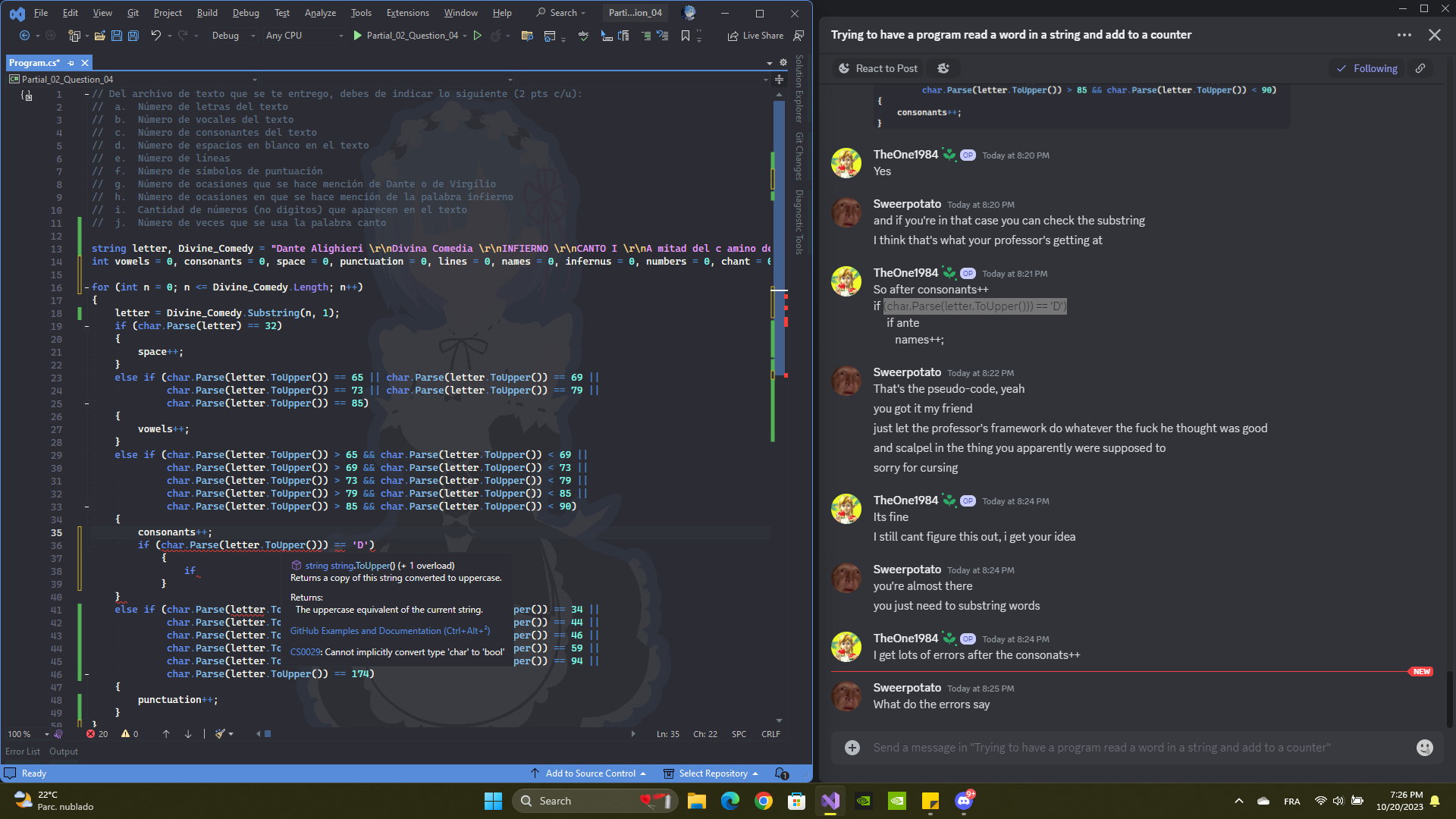
Remove the
)
after ToUpper())
That fixed some issues
What does it say now?
if (char.Parse(letter.ToUpper()) == 'D')
{
if()
{
names++;
}
}
I have this
There's an empty
if
statement, put the Substring
part in thereLike Divine_Comedy.Substring(n +1, 5)
Yeah, but remove the
+1
partoh ok
It says D_C is null
{
consonants++;
if (char.Parse(letter.ToUpper()) == 'D')
{
if(Divine_Comedy.Substring(n, 5))
{
names++;
}
}
}
What's
D_C
?Divine_Comedy, to simplify
Uhh, that's not true
Can I see the error?
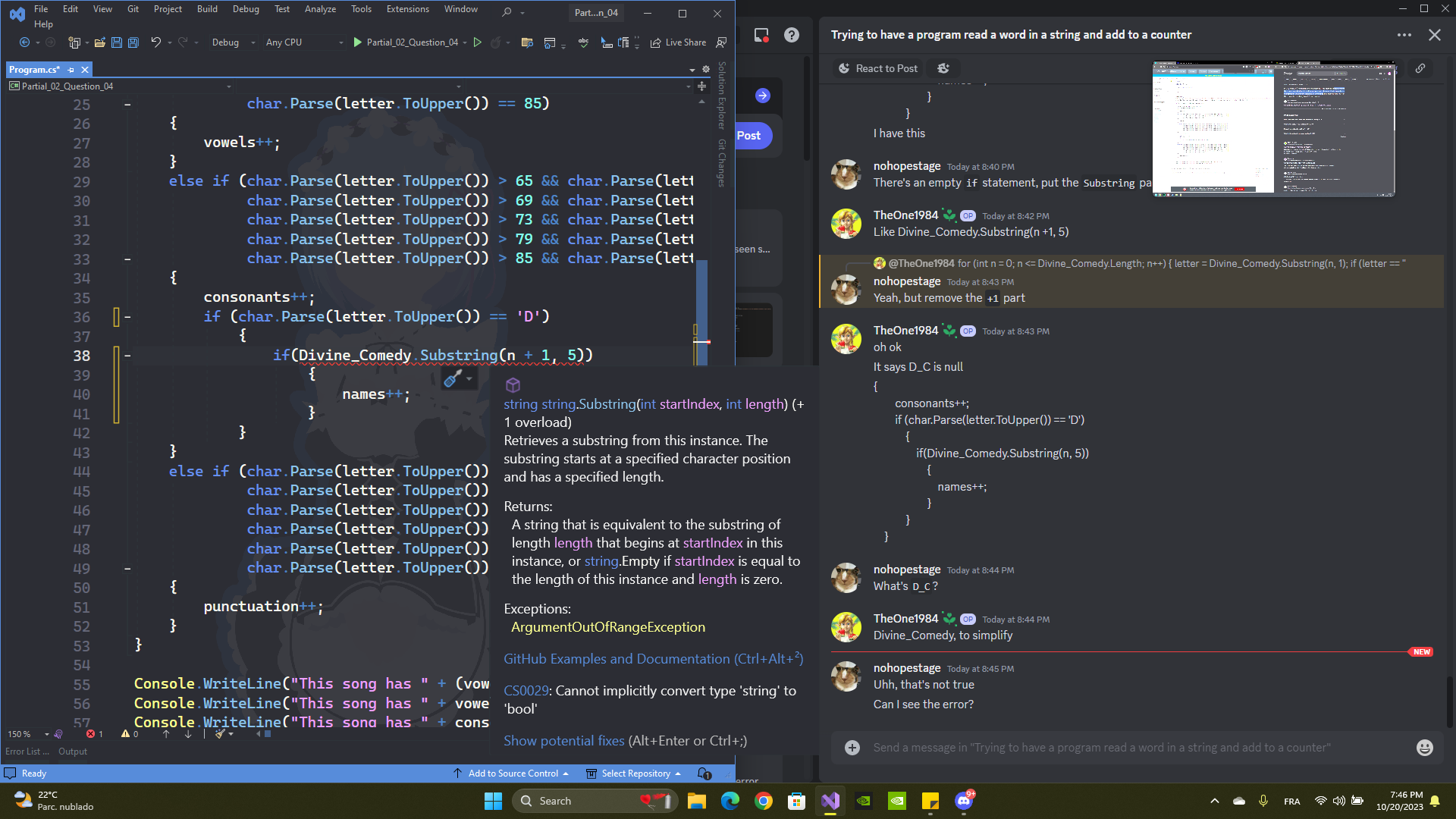
Do
if (Divine_Comedy.Substring(n, 5) == "Dante")
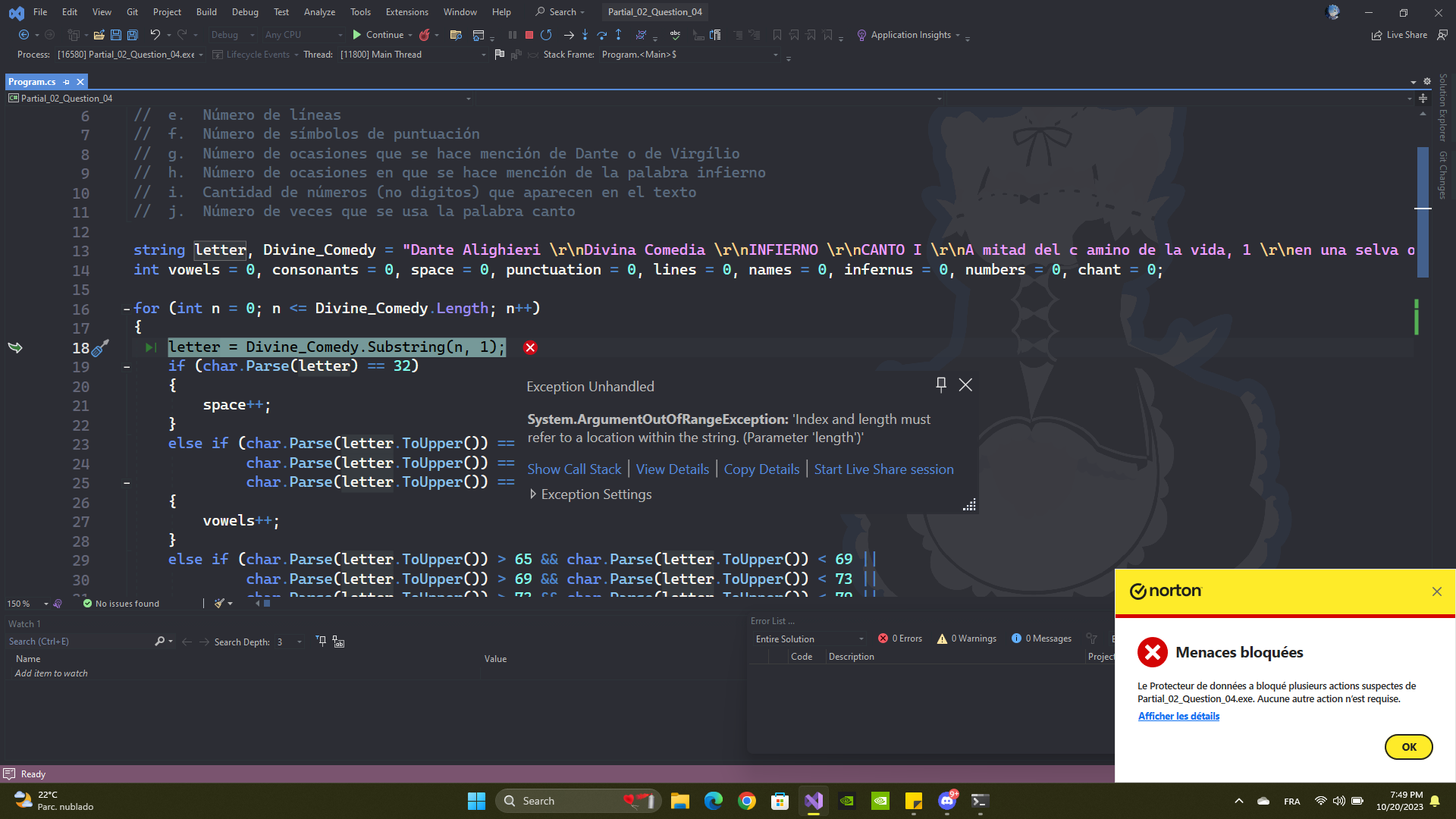
Change
<=
to <
at the for(...)
lineSolved the mistake, but names = 0
You're doing it like this, right?
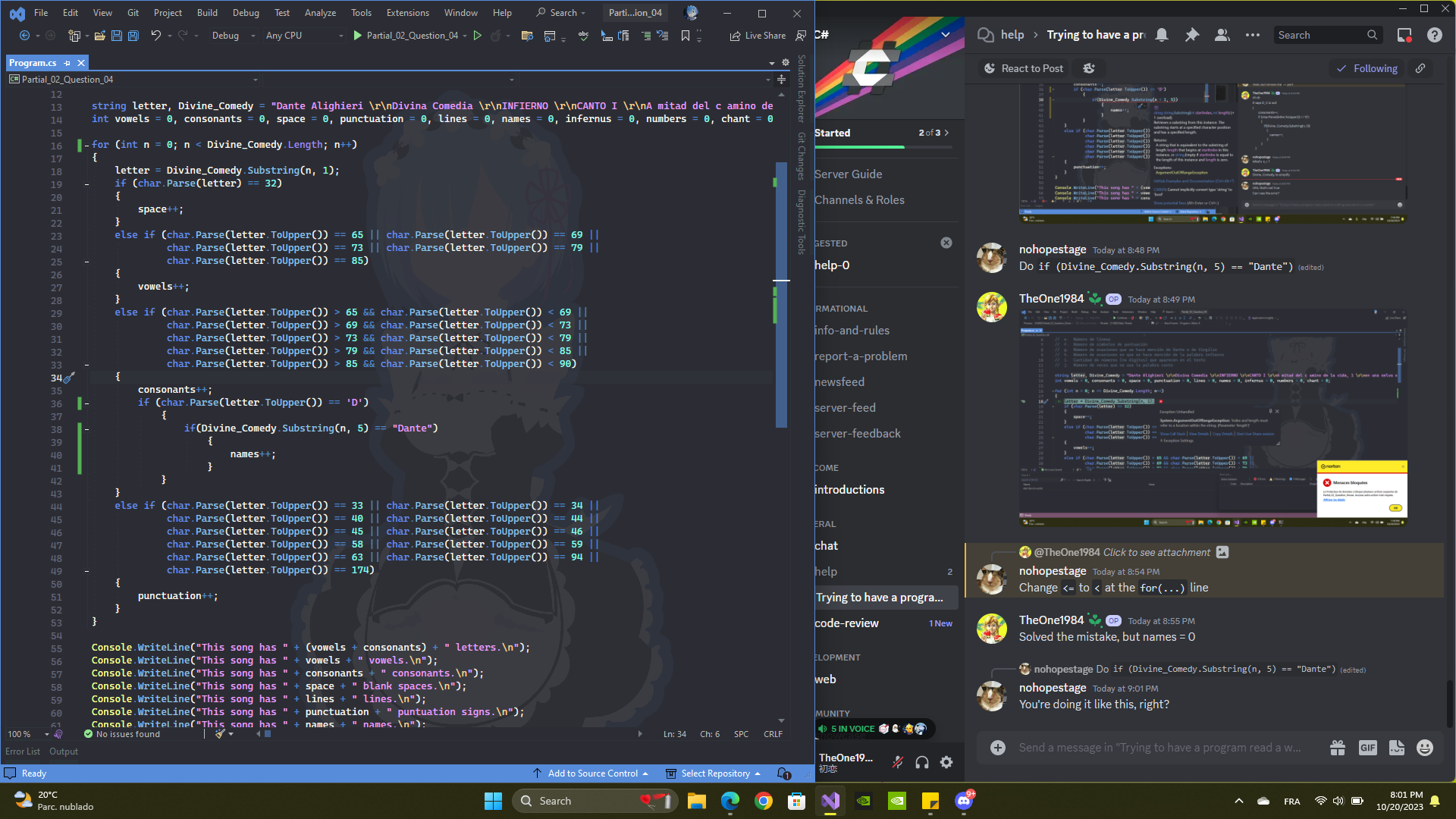
Yes
Remove the first
if
statement after consonants++;
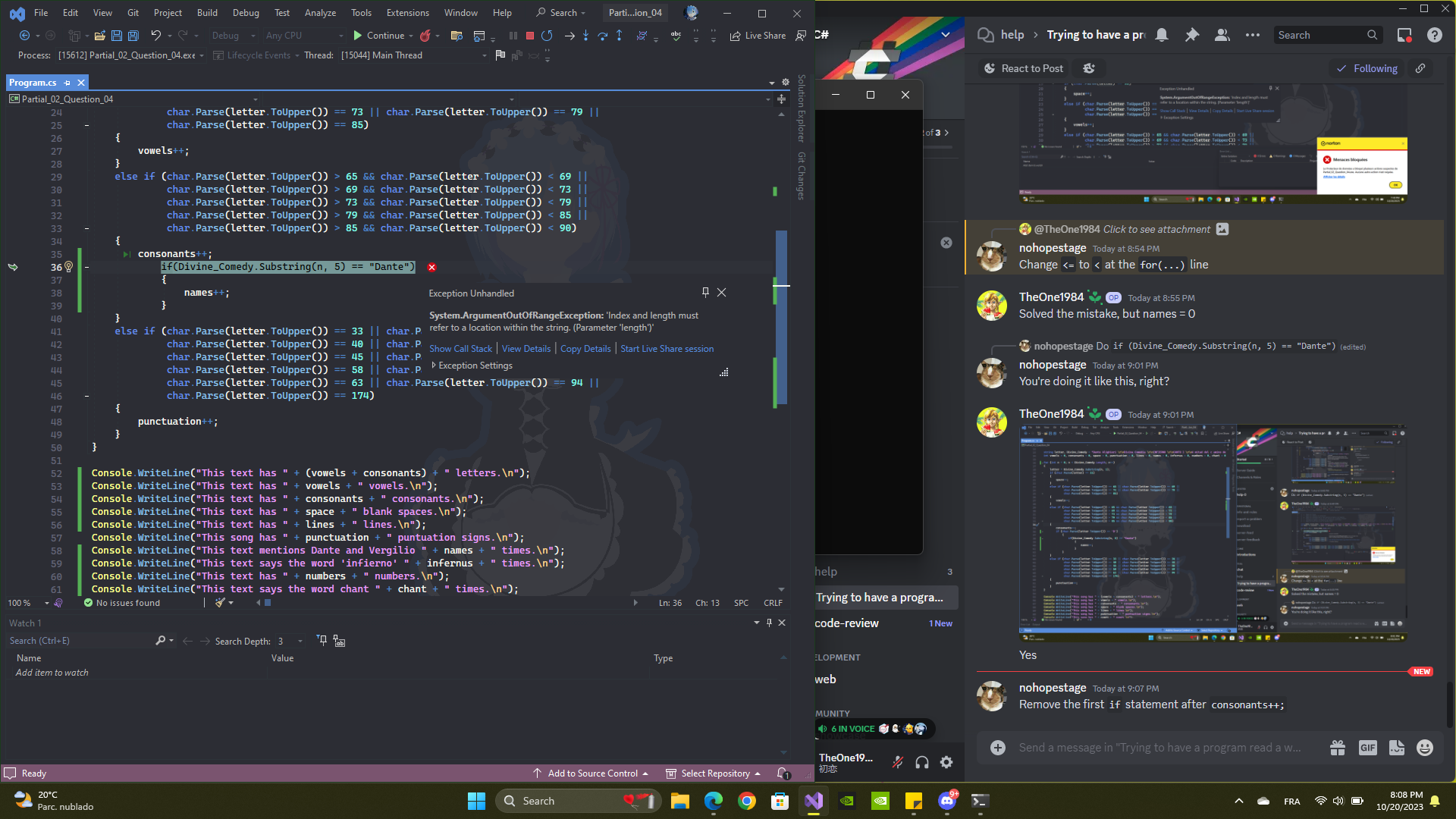
I got this
That's better, not sure why the first check fails though
And how do we fix this?
Replace it with
if (n + 5 < Divine_Comedy.Length)
And the "Dante"?
No, like this
Do I put it again like that?
Yes, but use the
if
statement I provided instead of the old oneFor the second one, right?
The first one
I did that and names = 0
I changed the second one and names = 17,000 +
Is it this with the Dante check inside?
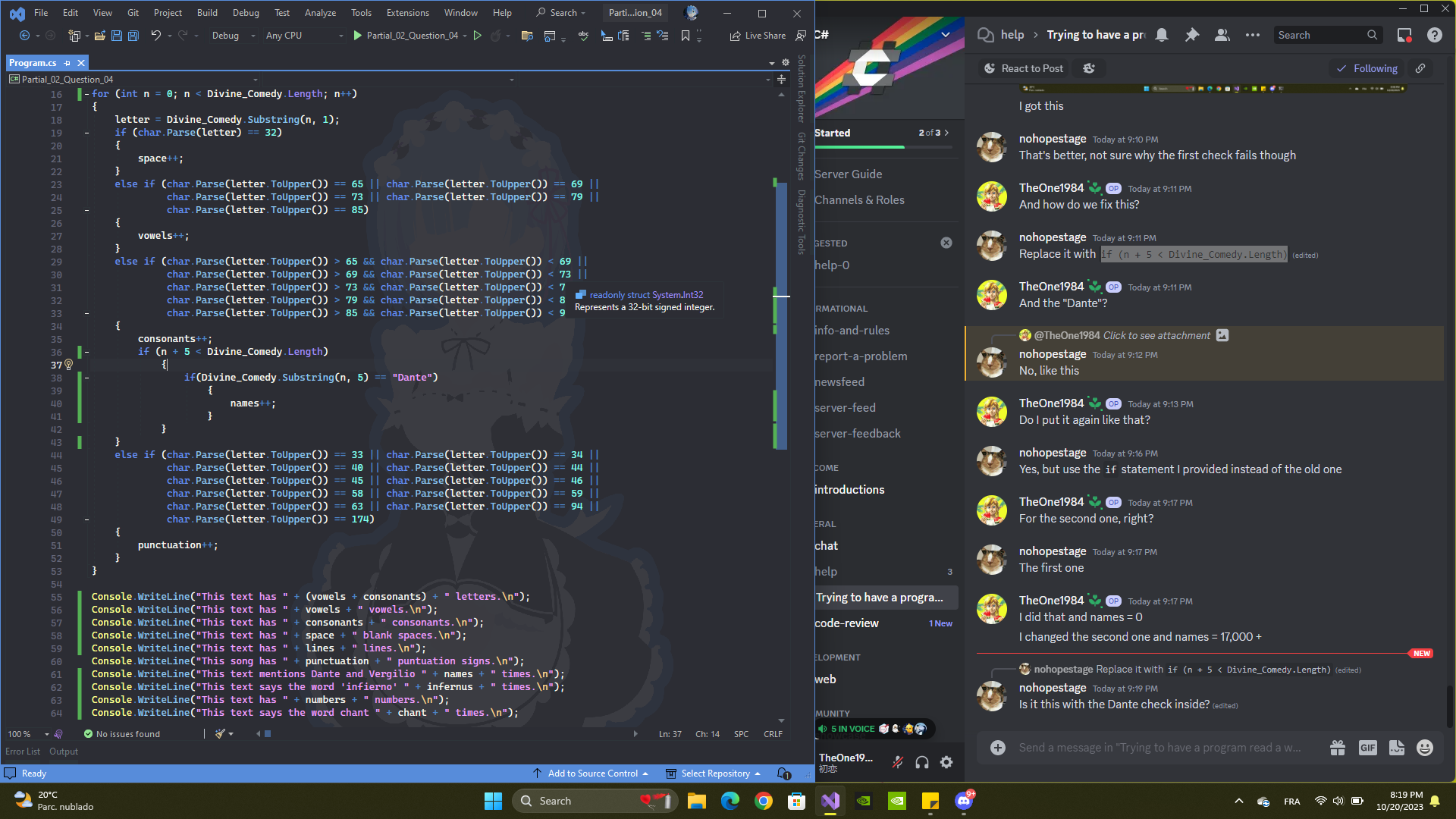
Yes, and names = 0
Can you post your code? $paste
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
int vowels = 0, consonants = 0, space = 0, punctuation = 0, lines = 0, names = 0, infernus = 0, numbers = 0, chant = 0;
for (int n = 0; n < Divine_Comedy.Length; n++)
{
letter = Divine_Comedy.Substring(n, 1);
if (char.Parse(letter) == 32)
{
space++;
}
else if (char.Parse(letter.ToUpper()) == 65 char.Parse(letter.ToUpper()) == 69
char.Parse(letter.ToUpper()) == 73 char.Parse(letter.ToUpper()) == 79
char.Parse(letter.ToUpper()) == 85)
{
vowels++;
}
else if (char.Parse(letter.ToUpper()) > 65 && char.Parse(letter.ToUpper()) < 69
char.Parse(letter.ToUpper()) > 69 && char.Parse(letter.ToUpper()) < 73
char.Parse(letter.ToUpper()) > 73 && char.Parse(letter.ToUpper()) < 79
char.Parse(letter.ToUpper()) > 79 && char.Parse(letter.ToUpper()) < 85
char.Parse(letter.ToUpper()) > 85 && char.Parse(letter.ToUpper()) < 90)
{
consonants++;
if (n + 5 < Divine_Comedy.Length)
{
if(Divine_Comedy.Substring(n, 5) == "Dante")
{
names++;
}
}
}
else if (char.Parse(letter.ToUpper()) == 33 char.Parse(letter.ToUpper()) == 34
char.Parse(letter.ToUpper()) == 40 char.Parse(letter.ToUpper()) == 44
char.Parse(letter.ToUpper()) == 45 char.Parse(letter.ToUpper()) == 46
char.Parse(letter.ToUpper()) == 58 char.Parse(letter.ToUpper()) == 59
char.Parse(letter.ToUpper()) == 63 char.Parse(letter.ToUpper()) == 94
char.Parse(letter.ToUpper()) == 174)
{
punctuation++;
}
}
I'll play around and see if I can figure it out
Console.WriteLine("This text has " + (vowels + consonants) + " letters.\n");
Console.WriteLine("This text has " + vowels + " vowels.\n");
Console.WriteLine("This text has " + consonants + " consonants.\n");
Console.WriteLine("This text has " + space + " blank spaces.\n");
Console.WriteLine("This text has " + lines + " lines.\n");
Console.WriteLine("This song has " + punctuation + " puntuation signs.\n");
Console.WriteLine("This text mentions Dante and Vergilio " + names + " times.\n");
Console.WriteLine("This text says the word 'infierno' " + infernus + " times.\n");
Console.WriteLine("This text has " + numbers + " numbers.\n");
Console.WriteLine("This text says the word chant " + chant + " times.\n");
All but the giagant string
Post it on this website
BlazeBin - qlmwxeejmxes
A tool for sharing your source code with the world!
Can't copy it on my phone. Please post it on https://dotnetfiddle.net/
C# Online Compiler | .NET Fiddle
Test your C# code online with .NET Fiddle code editor.
C# Online Compiler | .NET Fiddle
Test your C# code online with .NET Fiddle code editor.
Change
<
to <=
With you if chang?
Hmm?
Your new if change?
Yes
In this one
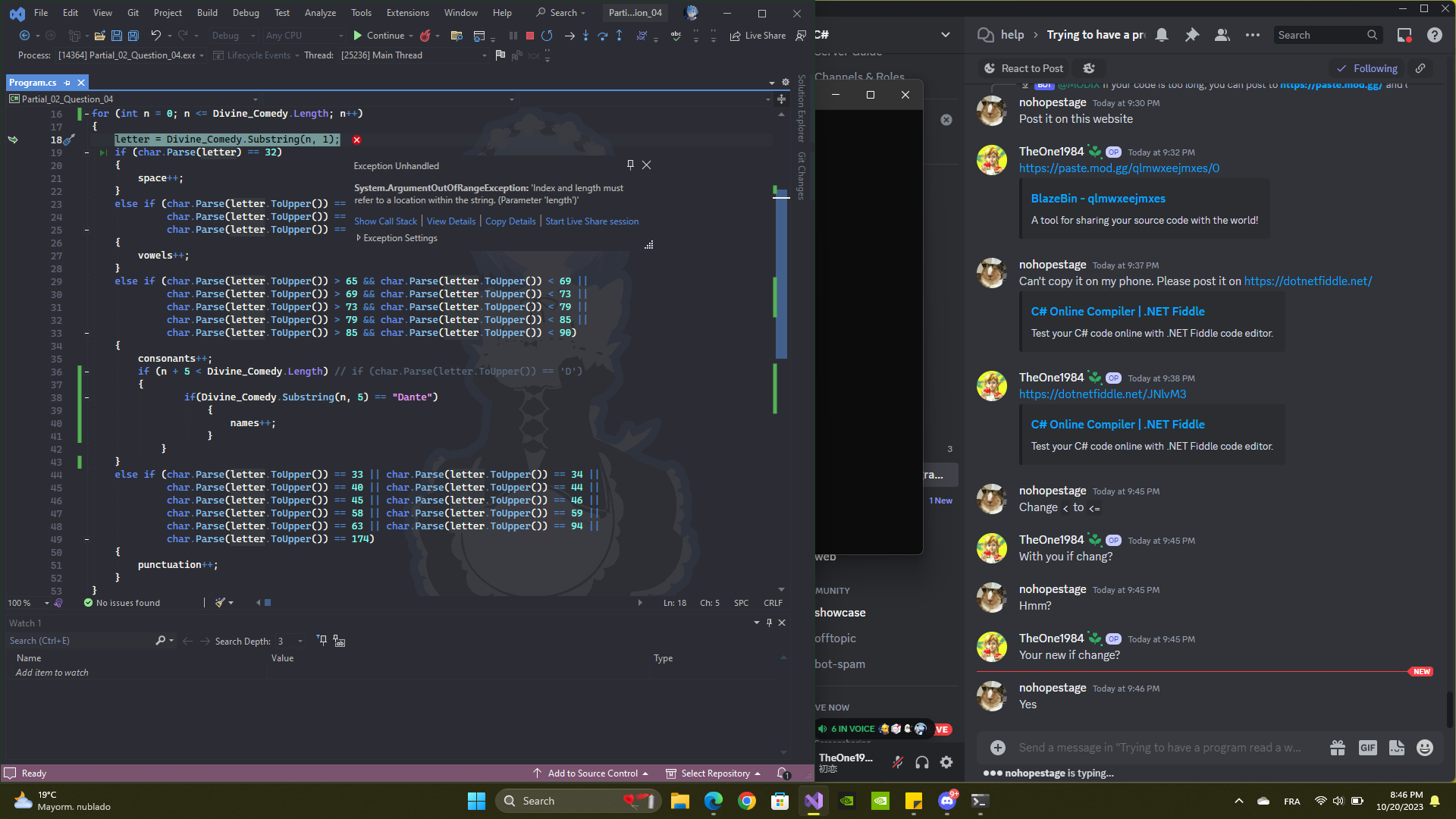
Error
No, this
Oh, let me try
names = 0
What's the code now?
consonants++;
if (n + 5 <= Divine_Comedy.Length)
{
if(Divine_Comedy.Substring(n, 5) == "Dante")
{
names++;
}
}
}
That's weird, it's working for me
It is???
Can you share it?
C# Online Compiler | .NET Fiddle
Test your C# code online with .NET Fiddle code editor.
It works on the web, bt not mine
Just copy the code then
I did
It works with the small string
Not the big one
Can you share the big one?
Sure
Thats the big string only
Oh, you have
ToLower
there. So you need to check for dante
Or just remove ToLower
Oooooh
It says 4 four Dante and like 33 for Virgilio
Two for Dante and 33 for Virgilio
I think it works
It works
Thank you so muc all that helped me, I dont want to tag you so I dont bother you, but Thank You So Much
Did you remove
ToLower
?
$closeUse the /close command to mark a forum thread as answered
Assuming you did. Glad it works for you
🎉
Just in case, 4 is not accurate because there are words that have dante in them
Oh yeah, fixed that after it got marked
ty
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.