❔ Amateur game dev LF help with some basic C#/programming knowledge
Hey all. Just for reference my knowledge in programming is limited to a university intro course for C++ back in 2021 and currently taking the Unity tutorial series on Unity's website for making basic games. My goal is to use Unity along with my digital asset creation skills to make a video game for my university thesis project. My major is in studio arts with a concentration in animation. Because programming takes some time to get used to and become fluent with I wanted to familiarize before my pre-thesis semester.
I'll be posting some very fundamental questions here that I'm still trying to wrap my head around. Once I understand them I'll never need to ask again. I find difficulty with many of the tutorials that explain programming as if I've been programming for several years. This skill is very abstract so while it is difficult I just need to learn and understand a little differently.
Question 1: what is the function of a "library" such as using System.Collections; or using UnityEngine;? Is it a reference tag kind of like the title or chapter in a cookbook, such that you couldn't cook without having read that first?
Question 2: What is class? Why are there so many? is there a relationship between a library and class?
58 Replies
there's a bit of terminology i should clear up real quick
those things that come after
using
are namespaces, a single library can contain any number of namespacesI see
they're just ways to categorize classes, so you dont have everything accessible at once if you dont need it
otherwise you'd have several thousand classes all show up in your intellisense, which isnt fun, and they can all conflict with each other
Does it affect file size, or does it just affect function of your program?
it doesn't, they're just used by your IDE and the compiler to figure out what things you want accessible
you dont even need to have the
using
if you didn't want to use the classes in them, things are accessible as UnityEngine.Vector3
for exampleso ignoring namespaces doesn't affect the program?
it doesn't, they're purely a thing for our convenience
Ok. So knowing them is cool but I can skip them and focus on the stuff beneath it
IDEs will automatically add them nowadays anyway when you try to use something from a namespace you don't already have a
using
forJust wanted to be sure it wasn't an issue of needing to reference in anything and the program just not execute
as for classes, they're a lot of things?
their biggest job is being a container for data, and they usually have methods on them for reading or changing that data they contain
Ok. That all leads me to a related question: I'm trying to understand the hierarchy of "code blocks" in a script. Can they be broken down into subsections that work similarly among all programs? Such as namespaces above containers above methods?
if i understand the question, yeah, programs follow a pretty normal style
Is there a picture you can share or something I should google to explain this visually?
explain what visually, exactly
Sort of like an organizational chart for a company
You have your boss, your supervisors, your foremen, then your laborers for example (example of a construction crew)
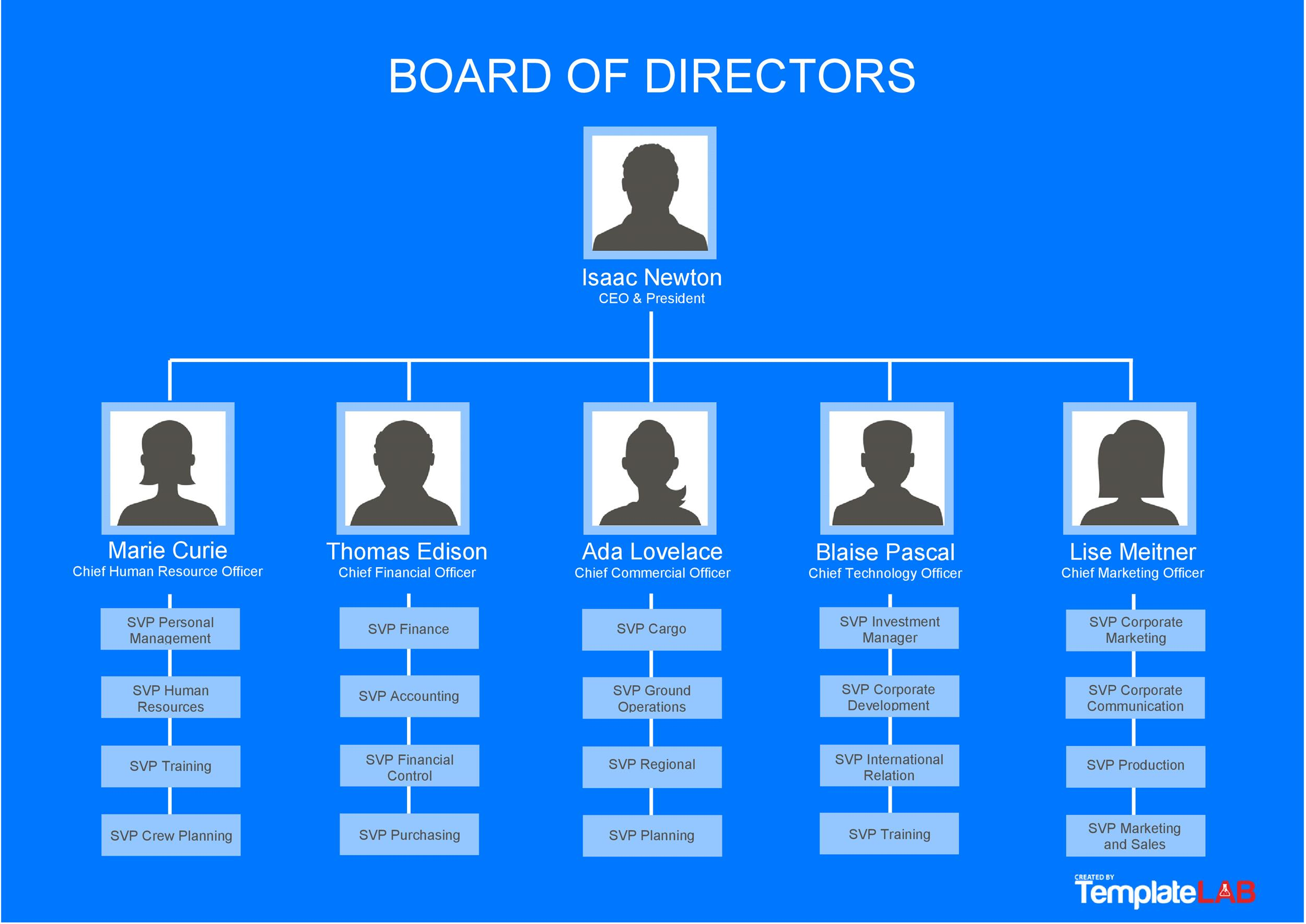
This comes to mind
But if I'm wrong feel free to call me out
well, i can do this
Assembly (a project, essentially)
- Namespaces
- Classes
- Methods
- Fields
- Properties (methods that look like fields)
- Events
- Structs
- Methods
- Fields
- Properties
- Events
- Interfaces
- Methods
- Properties
Yes! That's great
Thank you
classes and structs are (for the most part, they have one very big difference that isnt important at this point) the same thing
Ok, stuff I'll probably learn soon. I'm doing the 8 hr microsoft tutorial a little bit every day. Very good stuff there as well.
and interfaces are a different thing than those two that can help classes and structs say "i can do these things"
i feel a bit bad since that kinda dumps a lot on you, but it at least shows that heirarchy
So the namespaces are just there to say "hey, this is the kinda stuff we're using here", the classes, structs, and interfaces are the bigger picture items, and all the content inside those code blocks dictate how the program functions.
No not at all, I may not have understood the hierarchy otherwise
namespaces are just categories for "types", which is the name for classes, structs, and interfaces as a group
General Structure of a C# Program - C#
Learn about the structure of a C# program by using a skeleton program that contains all the required elements for a program.
I guess "skeleton" is the term I should be looking up as well
or just structure
yeah that shows the other "types" i omitted since they're not super important
delegates and enums
and it shows that namespaces can contain other namespaces, though you never really see that
Ok. Last question for now as I've taken up a bit of your time here: what is the easiest way to understand what a container is
If container is a broad term that can relate to a ton of different things, what's a few container examples that explains why it's a container?
Like I understand that an array dictate the position in a list from 0 to whatever number
Like a folder to me is a digital container
well, when i said "container", i just meant literally, in the source code, thats where you put your logic and data, so it "contains" those things
So does it function like a chapter title in a text book or does it allow things to execute as well?
I'm sorry if this is all asked really Barney style, I've just been struggling this bad lol
like if i have this
its a container for a first name and a last name, it stores those things inside itself
For context, what does Get do? Is it for input?
its just taking the first and last name and turning them into one string
so i can do something like this
Aaron
REPL Result: Success
Console Output
Compile: 588.862ms | Execution: 84.565ms | React with ❌ to remove this embed.
that myStudent "instance" of the class will store its own first and last name, to be accessible later
does that make any sense, lmao
Oh just barely lol
I'm trying to understand how all the lines relate, but that can be done another time
I'm struggling to find a real world metaphor for what classes are and do
I feel ya
Most people who explain online don't do it so well, so don't feel bad. If it were simple then thousands of people wouldn't all be asking lmao.
the GetFullName method and that last line aren't super important to what i'm trying to show there
public string GetFullName()
{
return FirstName + " " + LastName;
}
These absolutely relate, correct?
yes, thats one whole method
Like the immediate code block written beneath the string is meant for that string alone, so long as the } ends the method line
public
isn't super important, but then string
is the kind of data the method will give back, and GetFullName
is the name of the method, with the inside of the ()
being an (empty) list of the extra data it needs to do its job
{
is where the method's body starts, where it can actually do work, and }
ends that bodyI agree
its not optional, thats a requirement by the language to have
you are required to show where classes and methods start and end with braces
or it will yell at you 

Right, such that a sentence ends with a period.
or a line ends with ;
return FirstName + " " + LastName;
The return part
I'm not sure what that's fore
thats how you give back data (here a string) to whatever asked for it
Console.WriteLine(myStudent.GetFullName()); makes the text values of public string FirstName and public string LastName show in text
As Console means "text" essentially
the
FirstName + " " + Lastname
is just taking the first name, putting a space after it, then putting the last name after thatand WriteLine means "display it in text"
to write a new line of text to the consoile, yeah
I agree
I'm just not sure the purpose of return
methods can contain multiple lines that do different things, so it needs to know when you want to give back the final result
whatever called GetFullName wants some data back, here it wants the full name
return is saying "i have the full name you wanted, so now i will give that data back to you and stop working"
Ok so after the data is called, in this case to be presented in text-based form, the program is concluded after it displays
Otherwise it would just print infinitely?
Or probably error lol
yeah, the repl is a bit weird since it lets you put code anywhere on the top level
but in a normal program, if a method doesn't return any data (it has
void
as its return type), you don't need return
it will just end after the last lineHmm I seee
Thank you so much for taking the time to explain this, I have zero programmer friends and have struggled using tutorials and sites like quora or w3schools to explain this stuff for the minds of 5 year olds
Once I recognize the patterns and organization better it will all click, but I feel useless doing tutorials and practicing all while pretending I know how this stuff works or relates
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.