❔ Call API From Web API on Blazor Server
Hello respected community members,
I am new to Blazor Server and I am currently trying to fetch data from a .NET Core Web API to populate a data table. I am struggling with getting the data and then displaying it in my Blazor component.
I have an HttpClient service and I'm sending a GET request to my API endpoint. The API is working correctly.
However, I am having trouble deserializing this JSON response and showing it in a table in my Blazor component.
Here is the pertinent part of my code:
25 Replies
what's the error you have ? 🤔
I cant display the data here
the API itself is working properly
I mean the error when deserializing the json
you have an exception or something ?
Yeah, what's happening that you don't want to happen, or what's not happening that you want to happen?
There is no error, as the app returns No data found even though the API return result.
Is
getProspectError
true or is prospects
null?so the deserialization isn't the issue
it's before
prospects is null
Yes. When I there is no data to deserialize
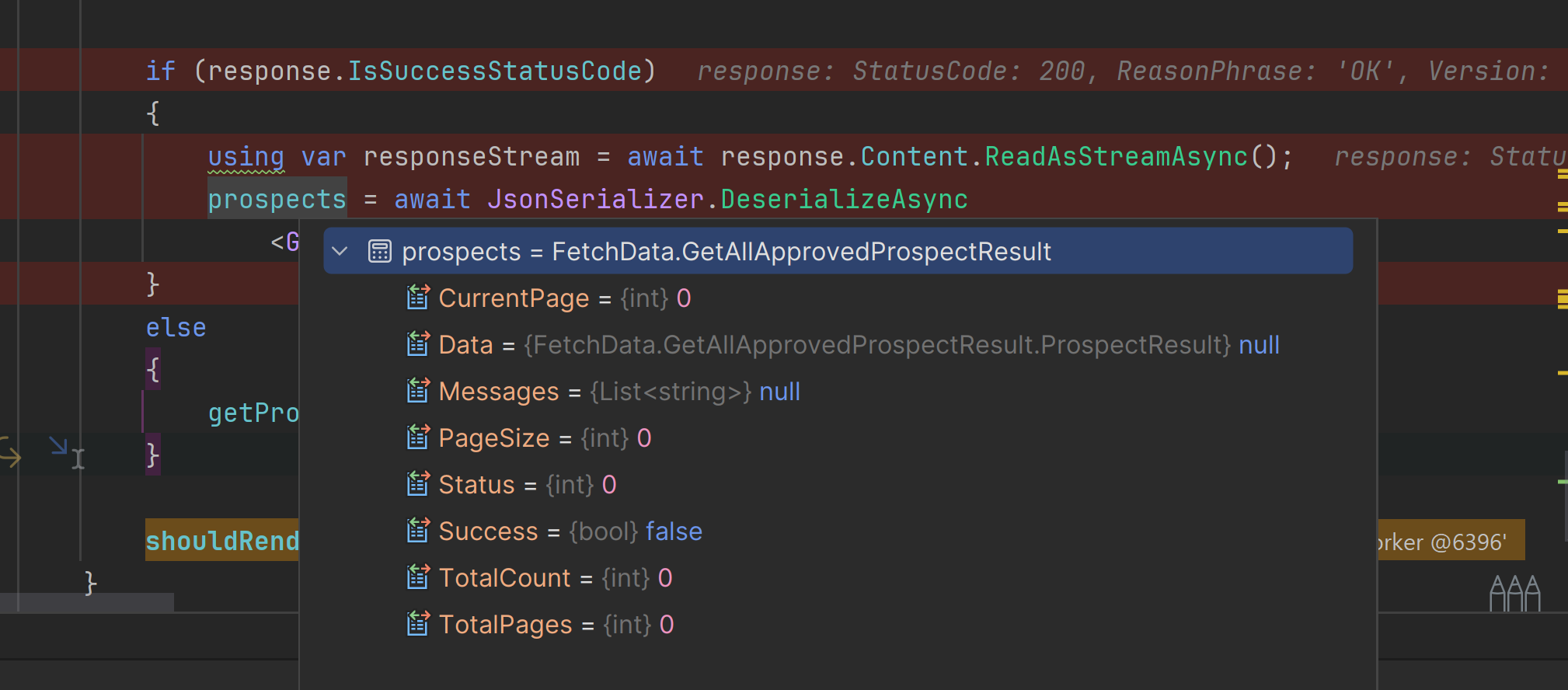
that's not null
if you read the stream manually as string what's inside ?
for me the first things to verify is check what's in your response string
one possibility is that the json property names are not being appropriately mapped to your class's property names
This the response
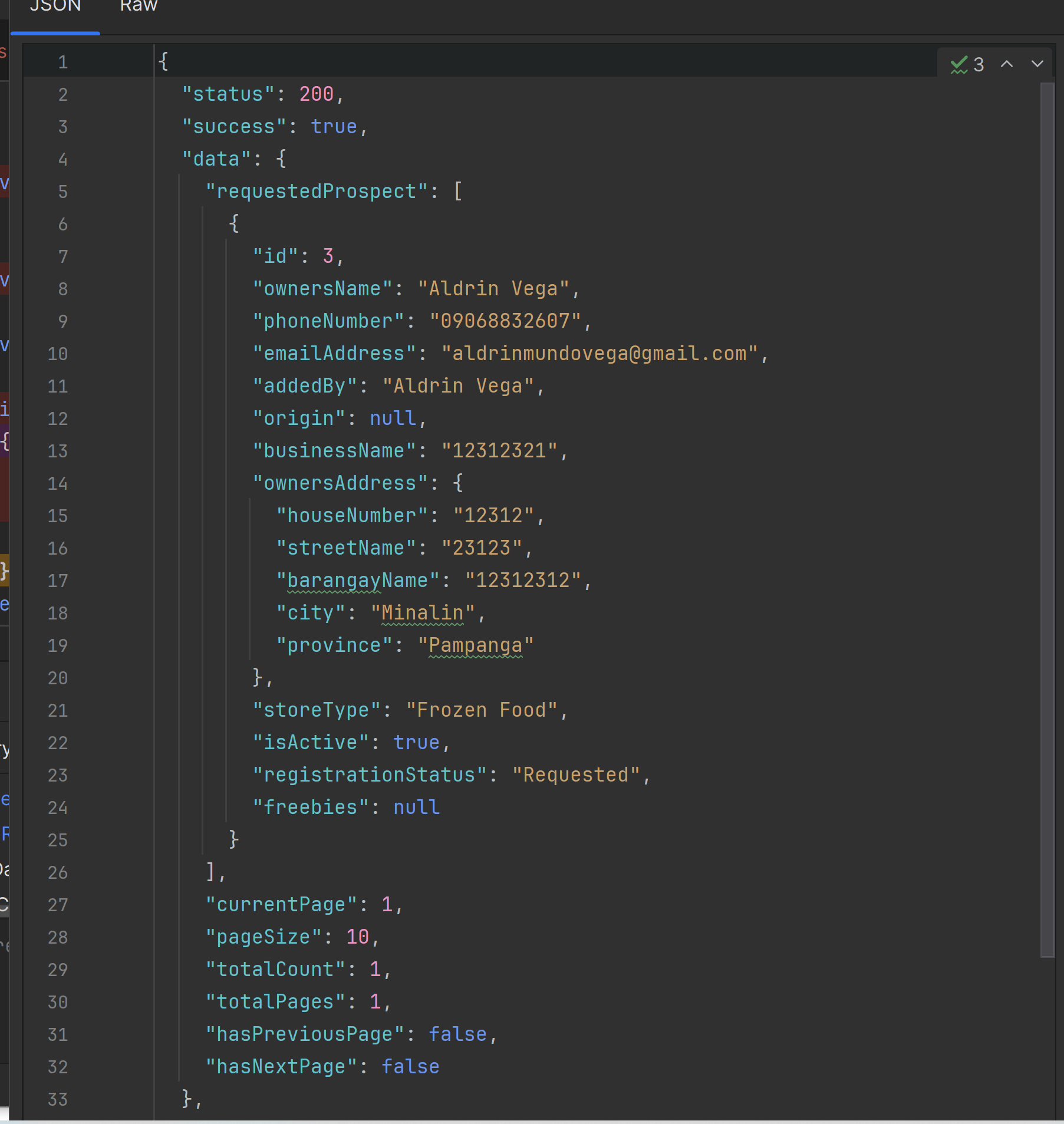
did you create your model based on this json or manually ?
I created this GetAllApprovedProspectResult
How to enable case-insensitive property name matching with System.T...
Learn how to enable case-insensitive property name matching while serializing to and deserializing from JSON in .NET.
oh yeah the insentive could be the issue
Based on the result from api for example the data.requestedProspect should match to the GetAllApprovedProspectResult Data.ProspectResult?
Should be Data.RequestedProspect?
it should have the same name yeah
I always use this website to create my model
https://json2csharp.com
Convert JSON to C# Classes Online - Json2CSharp Toolkit
Convert any JSON object to C# classes online. Json2CSharp is a free toolkit that will help you generate C# classes on the fly.
or the ide feature
You can either configure the deserializer to be case insensitive or you can add
[JsonPropertyName("propertyName")]
on each of your propertiesThat's a great tip, thank you! I hadn't realized that the JSON property naming could be causing the issue. I'll try setting the deserializer to be case insensitive and see how that works.
Thanks, I will check this one too!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.