Testing conditional rendering in child component
Hi, I am trying to test a particular interaction in my component.
I have two components, let's call them
Parent
and Toast
.
Toast
is a child of the Parent component.
Below is a code snippet from the Toast component:
The Parent
component has a button that fires an API call. If there is an error in sending the API call, the signal that controls the visibility of the Toast
component is set to True
The problem Im facing is that the code inside the <Show> does not seem to re-render and Im not sure why. I also console.log the value of toastVisibility and I can confirm that its initially set to false and after clicking the button, its set to true7 Replies
This is my test:
Once the button is clicked, I expect the fallback of the <Show>, i.e "not showing toast" to not be in the document. But after inspecting the DOM after the user clicks, I see that its still in the DOM
Whats strange is that I can see these console.logs running after the click event, which implies that the reactivity is already triggering the re-evaluation of derivedSignal()
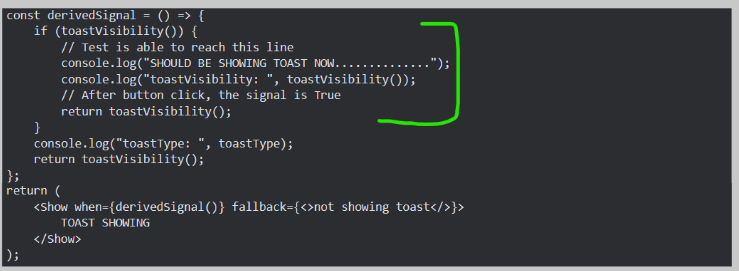
Try await find instead of query
Replaced the expect with this:
Still fails...
I'm currently abroad, and will need to wait until I get to my desk before I can test this myself.
No worries, do you know if there is some kind of playground environment where I can try to mimic this setup for you?
If you have a minimal reconstruction in a single test file, that would help