Why does `Outlet` not work when using `element` instead of `component` in the configuration?
Wrapper / container
This works:
This doesn't work:
8 Replies
it doesn't work because you're rendering the elements at the moment of declaration (remember, Solid has no VDOM)
You can convert
element
into a getterI don't understand, an
element not rendered
I am confused
element
gets rendered if it's a "parent" route but child routes don't get rendered. Why?
What does it mean to convert an element
into a getter?
When should one choose element
over component
, in a route?
----
The goal I have is to have an component such as: <AllowAuthenticated accept={SomeComponent} />
that returns SomeComponent
if user is authenticated, otherwise <Navigate />
to login.
I was thinking about doing it like:
but that apparently isn't working 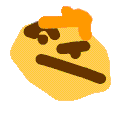
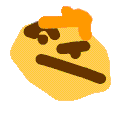
in which case the same thing happens with
element: <Example />
which is why you can solve this by doing:
or element: () => <Example />
also works (if you aren't using TS)Does having them imported through
lazy
not create getter for the elements?lazy
is irrelevant here as I'm talking about how elements in Solid behaveI will try to think about this and see if I can get it to work
thank you for helping <a:pepe_saber:1094297975141978214>
just follow this
🫡 planning to wrap it in a function as you showed, I'll let you know if it
works as expected! 🎉
thank you very much
<a:pepe_saber:1094297975141978214>
was going mad over this
what does
I see now, that way I would replace
thank you Toka

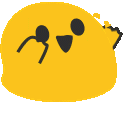


Component<void>
change? 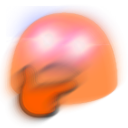
accept({})
with accept()
, is that what you 