ClientWebSocket hangs on ReceiveAsync, never continues
I will concede I am brand new to working with websockets. I'm following this Establishing a connection guide which uses websockets for connection. One of the recommended ways for initiating a connection is to connect normally, and then send an Authenticate event to the server upon which you will receive either an Error or Authenticated event.
In an obviously rough draft attempt to accomplish this feat:
my
_ws
is a class which wraps ClientWebSocket, and I can confirm my data is being sent (at least, no exception is thrown), but then when I try to receive, it never advances. Even stepping into it and breakpointing the actual ClientWebSocket#ReceiveAsync
method, it never advances beyond this point. Is there a trick I'm missing?Establishing a connection | Revolt
To get started, you should have:
15 Replies
this code is all client-side?
are you quite sure the server-side is good?
is it definitely sending something for you to read?
cause that's what ReceiveAsync() is waiting for
either some data to read, or the socket to close
I'm just following the docs on how it's supposed to be working. Maybe I'm sending my data incorrectly? I'm just writing raw JSON to the buffer and then sending that, I guess I should check what's actually being sent
I can confirm the server is actually functioning as the Revolt client exists and is working
let's see it
Well, I guess I will first fix the fact my event type is being serialized as an
int
instead of string
.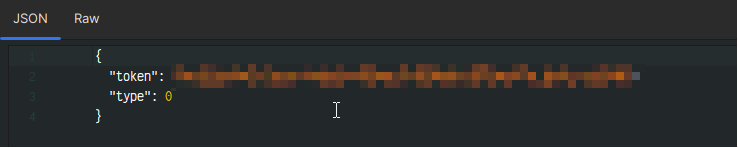
A little sidetracked - is there a way to define a
StringEnumConverter
for ALL enum fields in my models? it's a little annoying to have to put them on each individual model
oh nvm, figured it outyes, through the serializer options
I don't remember specifically, but I know I've encountered this before
(plus my other stuff obviously)
yeah
NICE, that was the issue all along

after like 40+ models written and I didn't realize it was never serializing enums correctly lol
ah, so it was throwing an exception deserializing, and you weren't seeing that?
actually the other way around. My
Authenticate
data was malformed since it was not serialized correctly, so it (the server) never sent anything in response
So then after that....just loop ReceiveAsync in the background to dispatch events?well, that's what I mean
an exception was happening when the server tried to deserialize the data
it sounds like you actually do need some hardening on the server
it should not just silently do nothing, it should return an error response or close the connection or both
I'm not the one who wrote the server, so I guess I can forward your complaints along lol
ah
well, that's significant info