I am new to c# and need help
Need Help ( I am new to c#)
This is my task :
Write a C# program that prompts the user for input in the following format
enter the console: "word-word-number". “Word” stands for words and “number” stands for one
numerical number. Each part is separated from each other by the separator "-". The program should
Make sure the input consists of exactly two words and a number. If the input is the
If the criterion is not met, the program should return with the error message "Error: The input must be from e»
2 words and 1 number”. Note that you do not need to do this task
Using the split method should be used and that the str. dealt with in school
Methods are sufficient to solve the task.
Valid entries:
* Hello World 20th
* I-Am-500
Invalid entries
Hello World 20-20
I-20 world
Hello
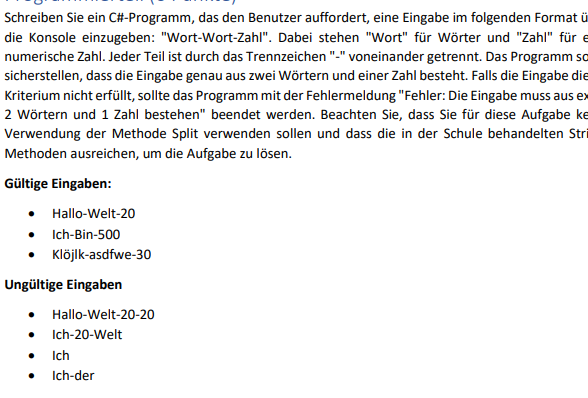
25 Replies
What have you got so far?
using System;
public class Program
{
static void Main(string[] args)
{
Console.WriteLine("Bitte geben sie bitte ein Wort-Wort-Zahl ein");
string nutzereingabe1 = Console.ReadLine();
string nutzereingabe2 = Console.ReadLine();
string nutzereingabe3 = Console.ReadLine();
string nutzereingabezusammen = nutzereingabe1 + "-" + nutzereingabe2 + "-" + nutzereingabe3;
Console.WriteLine(nutzereingabezusammen);
int integerumwandlung = 0;
if (!Int32.TryParse(nutzereingabe1, out integerumwandlung) && (!Int32.TryParse(nutzereingabe2, out integerumwandlung) && (Int32.TryParse(nutzereingabe3, out integerumwandlung))))
{
} else { Console.WriteLine("errror"); } } } idk if you can understand this bc its german
} else { Console.WriteLine("errror"); } } } idk if you can understand this bc its german
you're asking for each input separately, but the instructions to me sound like it should be asking the user for only one input then you have to check that it matches the format "word-word-number"
how can i chceck word-word-number that it matches the format
your assignment mentions using the Split method, so that would be how you'd separate the input, and in your code that you sent above you're already using
int.TryParse
, which is how you'd check that the relevant part is actually an intin the assignsment is that we are not allowed to use the split method because i have not leanred that and we should use the string methods instead
i dont know how i can check the whole format part like word-word-nummber i dont know how to check that with if
oh sorry, the translation is a bit weird round that part so I assumed the wrong thing
I don't know what you've used in school but there's IndexOf and Substring that can be used on strings, if you're not allowed to use Split
There you can look up all the string-methods (in german) for the task: https://learn.microsoft.com/de-de/dotnet/api/system.string?view=net-7.0#methods
I would consider Compare() as a possible candidate, but a few properties are quite interesting too. You can also loop through the string to check whether the character "-" is included twice and whether the last string can be parsed into an integer.
String Klasse (System)
Stellt Text als Sequenz von UTF-16-Codeeinheiten dar.
I should be able to do the part with string functions easly,but the difficult part for me is the part with if where I have to check whether the word-word-number format is correct. I know how it works but I can't practically implement it in C#
A bit off topic but aren't english naming the norm? Rather than country specific language?
Doing non english naming in programming school shouldn't be allowed (if i was a teacher). It's not a good habit to take i guess.
I can understand what you mean, because when programming everything is in English, but for us the teacher doesn't care and we can choose for ourselves whether English or German, since I'm still a beginner it's easier for me with German
I see, you will get to it eventually. But it's even better that the code is in english for entreprises or for online help <:KuuramaNICE_Edit:862500207395602442>
And i bet the best programmers in your class barely write their code in german, it' pretty much always the one writting up full english straight up for some reason 🤣
The easiest way would be to check each letter of the string within a loop to see if it isn't a "-" and append it to a new string. Do the same with a 2nd new string to the 2nd “-” and a 3rd new string to the end of the whole string. Now all you have to do is check which of the three strings you can parse into an integer and finally output the corresponding message.
Well, it's not checking which of the three strings can be parsed to int, it has to be the third one or the format is wrong
Well, in my opinion, it can be very difficult for a beginner to come up with good English names for the identifiers in addition to learning a new programming language, especially if he is not a native English speaker.
Clearly, but since they can already ask for help in english here, i don't expect 3 variable name to be a limiting factor
I haven't asked for english
never programmed in french, it's something that took some getting used to but it's worth the effort.
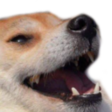
But when the first or second String could also parsed into a Integer the Error Message has to be displayed too.
Your point.
Right, yeah sorry I misinterpreted your message
i still dont know how to do it
If your teacher's saying you can do it with what you've already used in school, what have you used in school so far?
string functions, if function, loop. convert
Until this it's okay:
using System;
public class Program
{
static void Main(string[] args)
{
Console.WriteLine("Bitte geben sie bitte ein Wort-Wort-Zahl ein");
After that define one variable for the user-string-input like: string userInput = Console.ReadLine();
I would suggest just iterating on over the characters, appending each one to an array while also deleting it from the original string. Have arr1, arr2, and arr3 (one for each segment of the string). If the character is
or -
, start appending to the next array. Then convert arr1 and arr2 to strings, an TryParse arr3. Those are the first steps I'd take, but moving to the next array would probably needs methods and such.
However, there's certainly a better way of doing that.You can also do user Input.IndexOf('-') or user Input.LastIndexOf('-') to see if and where there is a '-' inside the user-input-string. But the easiest way would be to iterate over the user-input-string with a for-loop to check if the actual character is a "-".
excample: for( int i = 0; i < userInput.Length; i++) { ... }
before the for-loop declare a variable which counts the hyphen inside the userInput: int hyphenCounter = 0;
inside the for-loop comes a if statement which ask if there is a "-" inside the userInput like: if(userInput[i] != "-"){ ... }
using System;
using System.Reflection;
public class Program
{
static void Main(string[] args)
{
Console.WriteLine("Bitte geben sie Wort-Wort-Zahl ein");
string nutzereingabe = Console.ReadLine();
int posbindestrich1 = nutzereingabe.IndexOf("-"); int posbindestrich2 = nutzereingabe.IndexOf("-",posbindestrich1 + 1); Console.WriteLine(posbindestrich1); Console.WriteLine(posbindestrich2); string wort1 = nutzereingabe.Substring(0, posbindestrich1); string wort2 = nutzereingabe.Substring(posbindestrich1 + 1, posbindestrich2 - posbindestrich1 - 1); string wort3 = nutzereingabe.Substring(posbindestrich2 + 1); Console.WriteLine("Das erste Wort ist:" + wort1); Console.WriteLine("Das zweite Wort ist:" + wort2); Console.WriteLine("Das dritte Wort ist:" + wort3); Console.WriteLine(wort1); Console.WriteLine(wort2); Console.WriteLine(wort3); int überprüfung1 = 0; int überprüfung2 = 0; int überprüfung3 = 0; if(!int.TryParse(wort1, out überprüfung1 ) && !int.TryParse(wort2,out überprüfung2) && int.TryParse(wort3, out überprüfung3)) { Console.WriteLine("Eingabe ist richtig"); } else { Console.WriteLine("Eingabe ist nicht richtig"); } } } is this right?
int posbindestrich1 = nutzereingabe.IndexOf("-"); int posbindestrich2 = nutzereingabe.IndexOf("-",posbindestrich1 + 1); Console.WriteLine(posbindestrich1); Console.WriteLine(posbindestrich2); string wort1 = nutzereingabe.Substring(0, posbindestrich1); string wort2 = nutzereingabe.Substring(posbindestrich1 + 1, posbindestrich2 - posbindestrich1 - 1); string wort3 = nutzereingabe.Substring(posbindestrich2 + 1); Console.WriteLine("Das erste Wort ist:" + wort1); Console.WriteLine("Das zweite Wort ist:" + wort2); Console.WriteLine("Das dritte Wort ist:" + wort3); Console.WriteLine(wort1); Console.WriteLine(wort2); Console.WriteLine(wort3); int überprüfung1 = 0; int überprüfung2 = 0; int überprüfung3 = 0; if(!int.TryParse(wort1, out überprüfung1 ) && !int.TryParse(wort2,out überprüfung2) && int.TryParse(wort3, out überprüfung3)) { Console.WriteLine("Eingabe ist richtig"); } else { Console.WriteLine("Eingabe ist nicht richtig"); } } } is this right?