Is it possible to remove predicate by condition?
I have following:
LastNotificationId
can be null or 0 and this case I wanna remove .Where(model => model.Id <= arguments.LastNotificationId)
and return just limited number of items13 Replies
u can split the method chaining up:
oh
You can also make a nice extension method like
it is good idea but can we just remove predicate from linq query?
No, you cannot
the
predicate
needs to be Expression<Func<T, bool>>
, i thinkur right
Yep yep, right
thanks for helping me!
I'm sorry but
how to use predicate in my case? 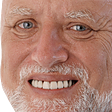
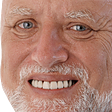
the
predicate
is the same as in the normal Where
call, just afterwards u pass a boolean value that if true actually adds the checknever tried it with
IQueryable
and Expression
but i guess theoretically u could do something like
so it would simply add your perdicate or an "always true" predicate.
but in normal linq its a horrible idea because this will check the "always true" predicate on each element as well (even if not needed)
and i guess the generated sql would look quite weird as well (and maybe the dbms would do the check for every row as well)ah I see
thanks!