Issues trying to create new error types
Hello everyone.
I'm trying to create my own extensible error type, based on the RFC 7807.
The idea being, I could override it with sane defaults, and only having to deal with a subset of the arguments
The issue is that, my new
unknownCapabilityException
raises an CS9035, Required member ‘RFC8807Error.title’ must be set in the object initializer or attribute constructor.
(and same for type), even tho it's set in the constructor of unknownCapabilityException
... Any pointer on how I could fix this issue ?9 Replies
using the property initializer doesn't call your ctor
even when doing
I get the same error message. Even worse, now even
detail
is undefined.just don't use required keyword for things not setup in the constructor
I'll give this a try when my Visual Studio update is finished, thanks 🙂
They are required per the spec, I would have prefered if it was forced by the compiler to the consumers of the API, but I'm not going to fret too much if it's not possible
I have the latest rider version and it doesn't compile even removing required on title and type
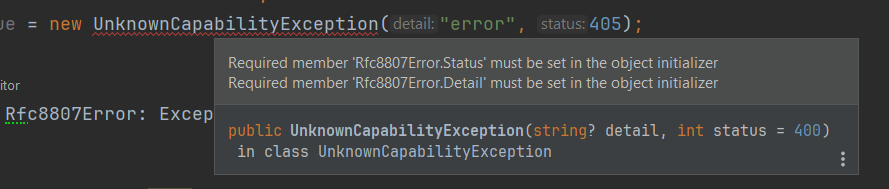
the error is not logic
Removing the
required
did the trick. I have warninng about property being maybe unset, but that's someone else problem
Thanks for the help 🙂I think the inherithance fucked up the required keyword
@COLAMAroro you have to add
[SetsRequiredMembers] on your children constructor
https://discord.com/channels/143867839282020352/598678594750775301/1175826056607969380