Filtering by a related table column changes the table id
Hi, I'm following the Owners, Pets, Treatments demo and I'm having issues creating a simple toggle filter by owner's email for the Patients resource.
My problem is that after applying filter, the Patients table now uses the owner's id instead of the patients id. What I'm doing wrong?
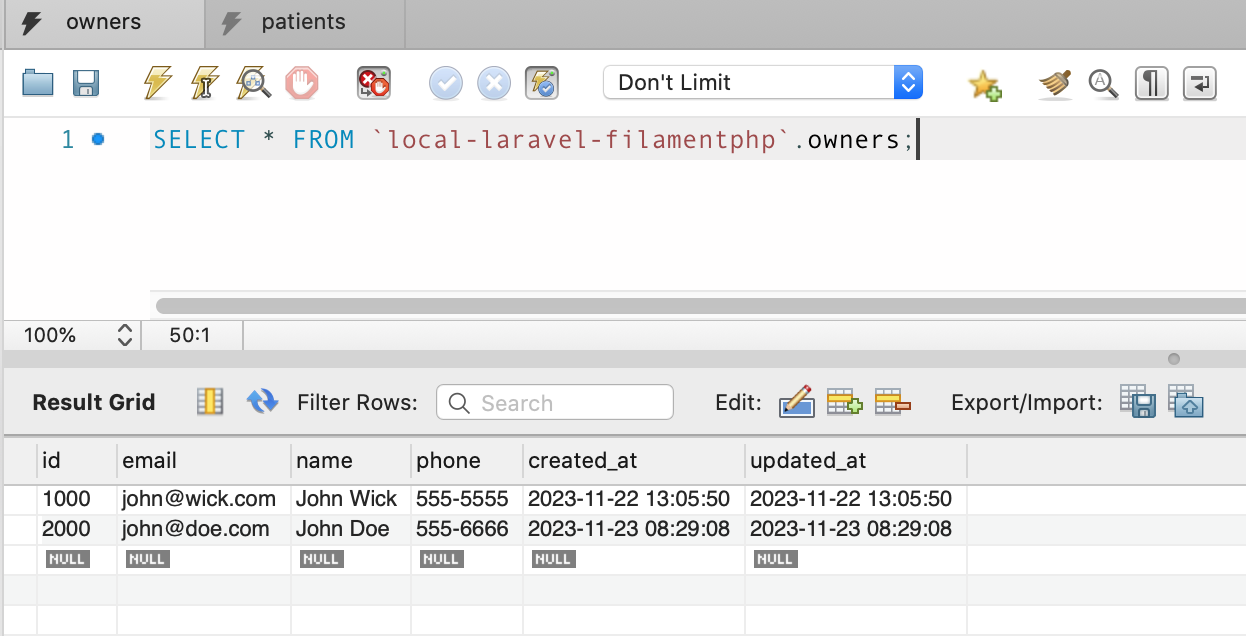
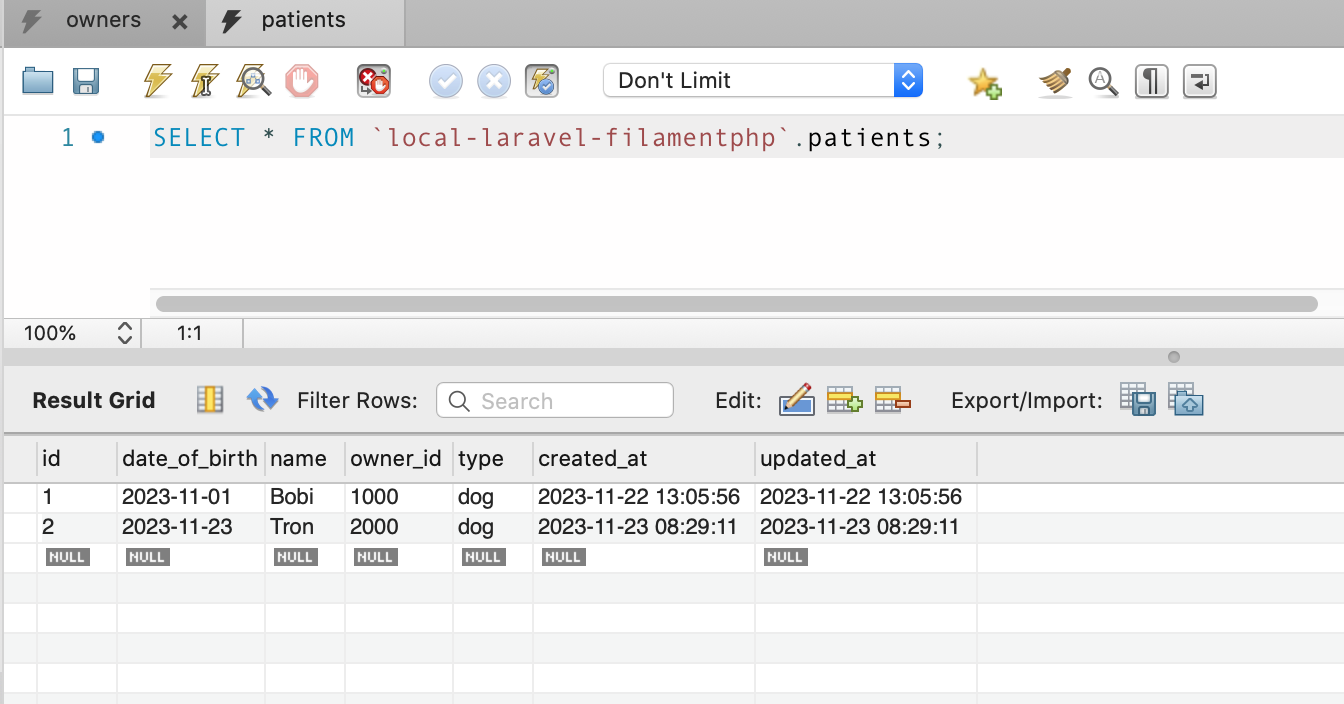
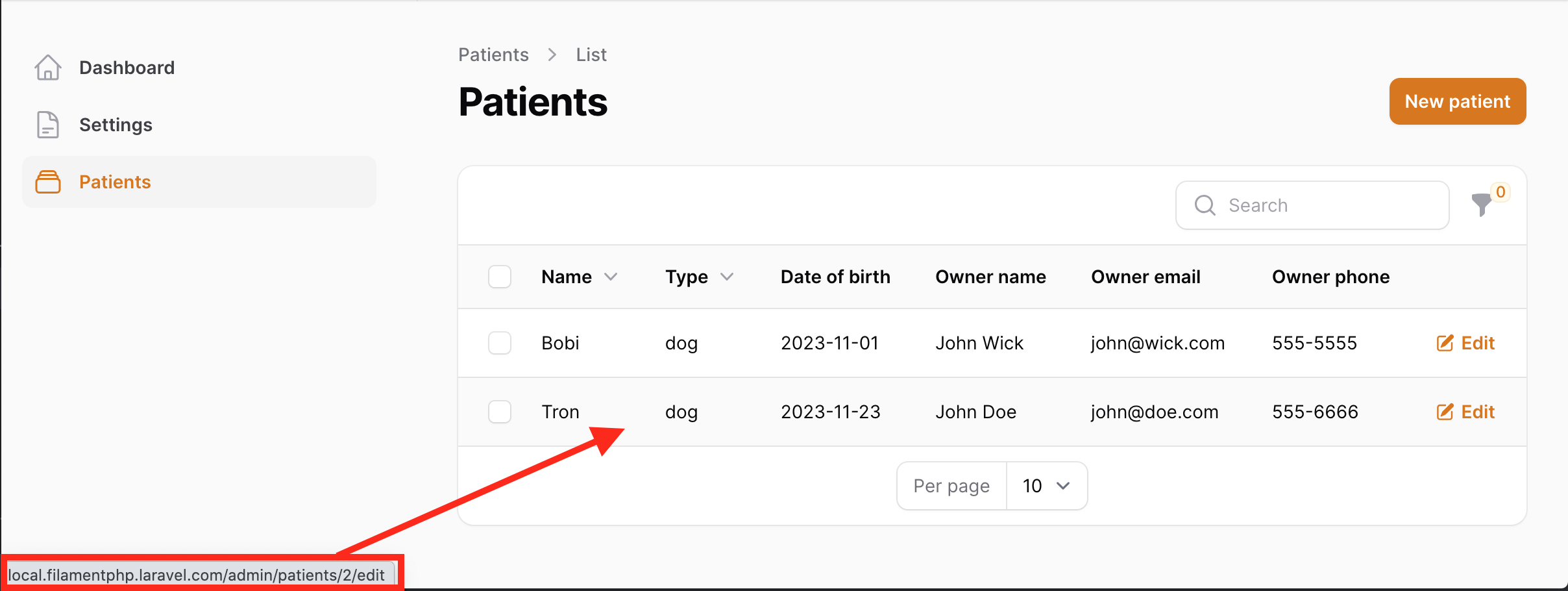
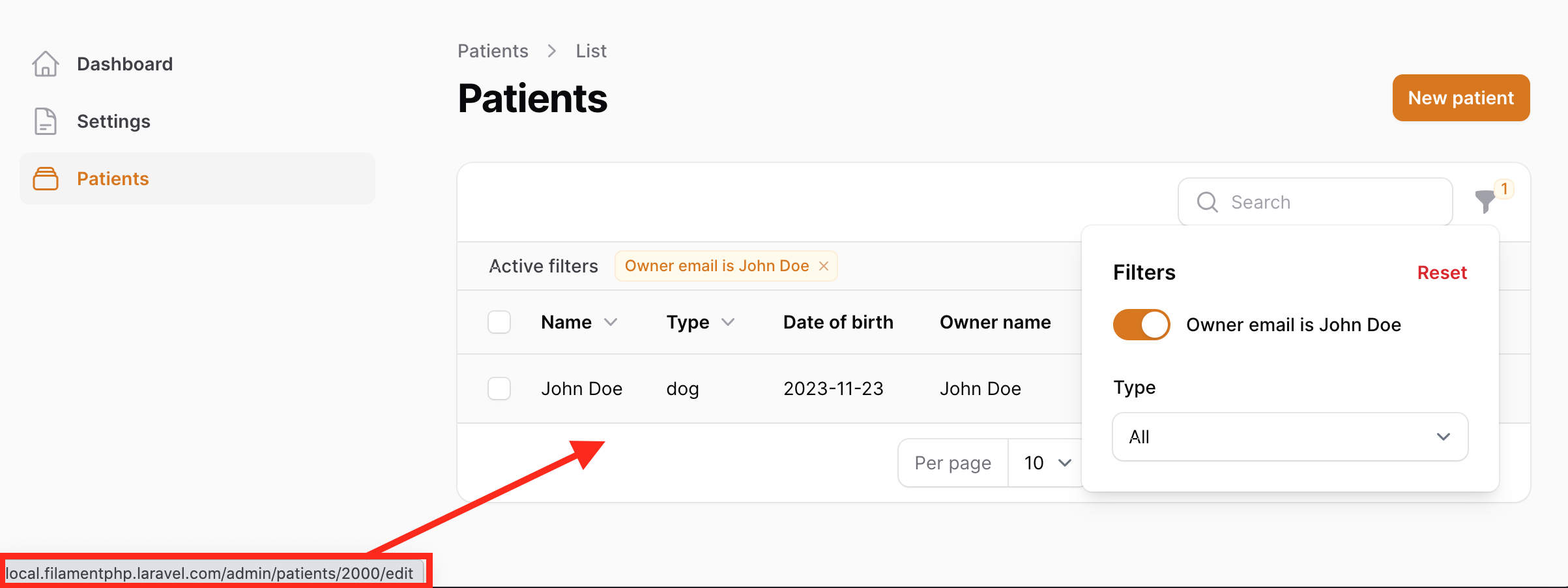
Solution:Jump to solution
You are adding a join with a table that also has an ID. Try adding a
select('your_table.*')
via the table query6 Replies
Solution
You are adding a join with a table that also has an ID. Try adding a
select('your_table.*')
via the table querylike this?
Thanks @Dennis Koch , btw great export plugin
Thanks ☺️
Ouch, ... not that fast. It turns out that now the ids are showing as expected but when the filter is active and I try to use the search box (individual or not) a new error raises because the name is ambiguous. Any advice on how to fix it?
Is this not a relationship? Why do you need the join? I think a SelectFilter with a relationship would handle this for you.
Ok, ok now I think I'm getting it. Modifying the base query is a bad idea, it would be better to use Eloquent's with and whereHas. sorry for the dumb question, the beginning is always the hardest.