How to "$set" Repeater itemLabel()
Hi... I manage to set repeater number base on the
Select::make('quantity')
but how can I set itemLabel
in afterStateUpdated
?
Let say if the selected quantity
is 3, the repeater will generate 3 Repeater TextInput
with itemlabel
value "Student" to all 3. How can I dynamically make it "Student 1", "Student 2", "Student 3"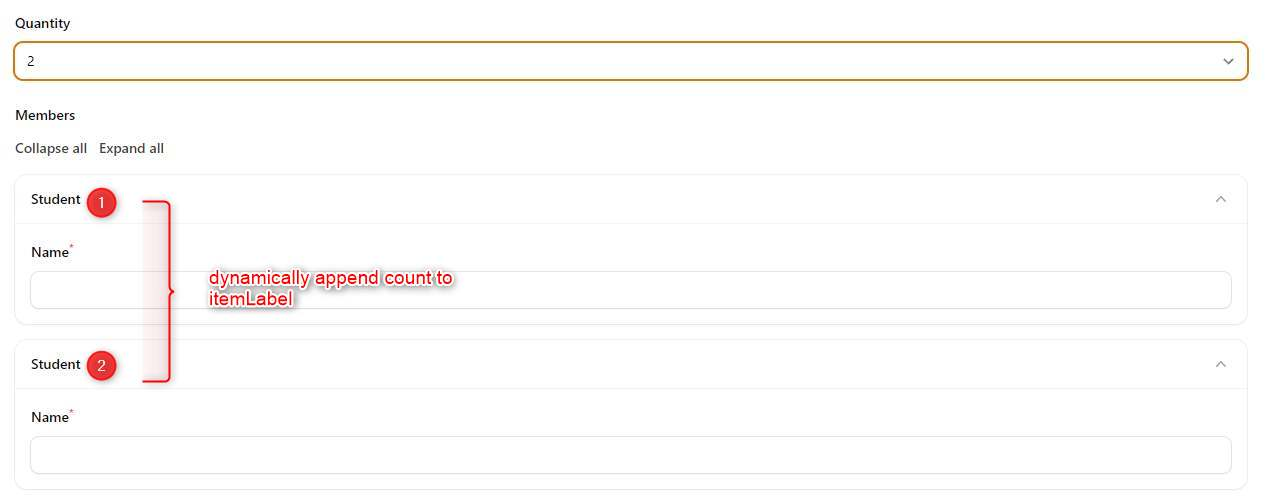
8 Replies
ItemLabel should accept a callback where you can return whatever you need as the label.
If use callback in
ItemLabel
, the return value still will be same to all the generated Repeater. I just want to append numbering ,maybe by using some sort of array index value but I have no idea where to startNot a copy paste solution but explore this.
Basically, you just need a way to check the value in the repeater array to return the key of the state.
Sudo code:
Foreach(state as key => value)
If value === blah
return ‘Student ’ . Key
I’m sure I’m not explaining it well, but the itemLabel has access to the state which for a repeater will be an array so you can use any php conventions to determine the index of the array that works for your use case.
alright, I will take a look into it... thanks @awcodes
Solution
I did this but the
foreach
inside itemLabel
will repeat entire array. Let say I select the quantity 2, then the loop log (Log::info($get('members'))
) will look something like this
Lastly, what I did is I add another id
array in afterStateUpdated
and add another TextInput::make('id')->hidden()
in the repeater schema then add ->itemLabel(fn (array $state): ?string => 'Student '.$state['id'] ?? null)
. Seem like confusing but here is the full codeand here is the output
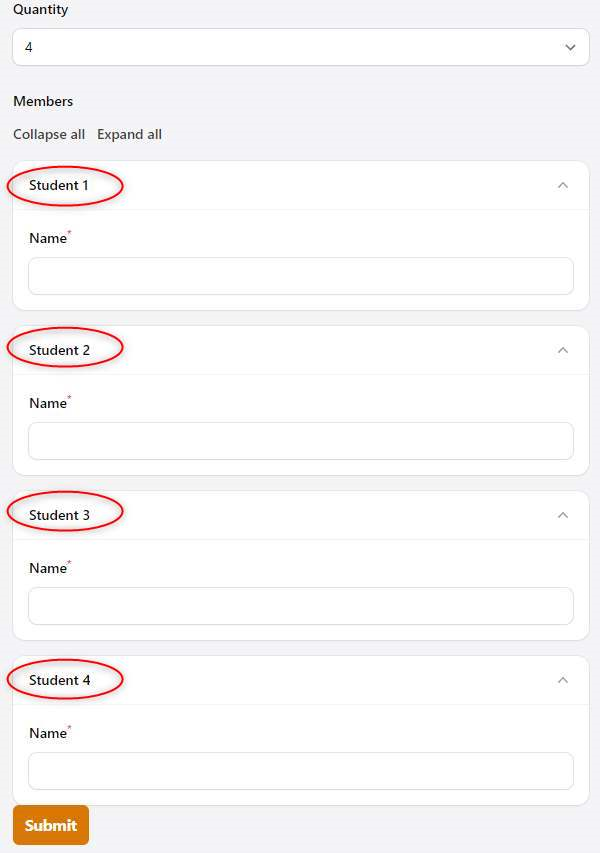
The idea is I want add numbering to each repeater. The list could be large so the numbering will help user to identify how many list are there
I believe there could be better workaround on this and please share here if you do so.