✅ Fluent API Relationship Help
Hey there,
I am trying to write out the relationship between the Route and OrderRoute table as shown in the picture, but I am lost on actually how to do it. This is my first time working with EF in general. Any advice would be appreciated.
What I have so far:
Thanks
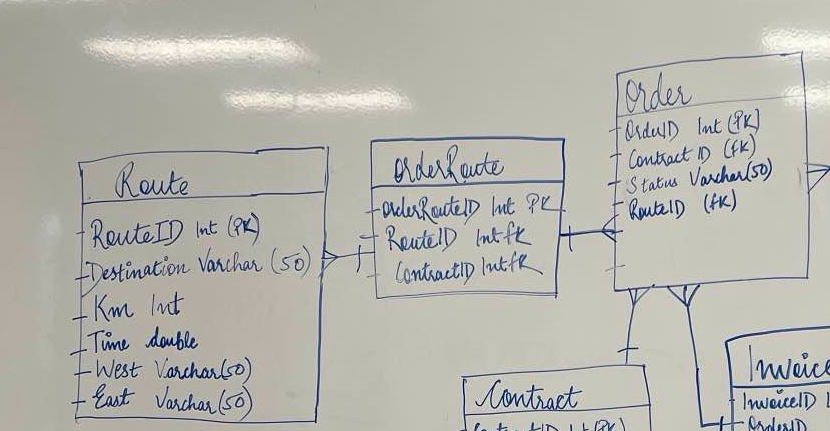
16 Replies
You only really need to configure one side of the relationship
yeah you can get rid of most of that configuration if you follow EF conventions
Like non-nullable properties automatically being required
Do you mean attributes in the class themselves instead of using the modelBuilder?
Thank you!
no, i mean nothing at all
EF can determine things like primary keys, navigations, required or not, etc just by how you define the properties
Oh.. I didn't know that
Conventions for relationship discovery - EF Core
How navigations, foreign keys, and other aspects of relationships are discovered by EF Core model building conventions
i only use the fluent API to configure things that can't be detected by convention
For example
That's neat but looks very confusing to me tbh. We're required to write it all out for this group project, so it's easier for all my team members to understand
Gotcha that's actually such a great feature
Thanks a bunch
school and teaching a less optimal way to do things
name a better duo
Lol yup.. still teaching .NET Framework
you shouldn't mix annotations and fluent imo
between that and EF conventions your model is like triple redundant 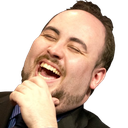
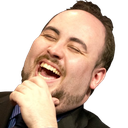
Fair
But relationship wise with the image I provided, would that be accurate?
i don't think that's how you're supposed to use the ForeignKey attribute
but if you're using some old EF version maybe it is, i've only used Core
Gotcha, thanks a bunch