Advent of Code Day 1
I'm not sure why my code isn't accepted even though I'm sure it works:
7 Replies
you're adding the numbers together wrong. if your first digit is
5
and your last digit is 7
, you should be adding 57
, not 12
Overall += firstDigit + lastDigit;
is the problme lineRight, that completely went over my head, I need to concatentate them first then parse them
alternatively, you can multiply the first digit by 10
so firstDigit *10 + lastDigit (which is also more efficient)
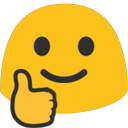
Thanks :)
I'm also a little unsure why this doesn't work :
It's late here now so I'll try again later
you're only checking a single character in the string, so it will never match on the "word" numbers
you'd need to rework the logic that loops from end to beginning to be aware of characters after it