Can someone explain to me what a bool is and how I can implement it in other data structures?
Pretty much title, I'm trying to code a simple game in C# and my teacher suggested I use booleans to make a certain statement come up given if the boolean was true, can someone explain to me how it works?
340 Replies
Thank you so much JP! I've just been learning computer science and I won''t lie it has been a bit tricky to start off @JP
thanks for trying to help me out
@JP Hi, sorry to ping, but can you explain this to me?
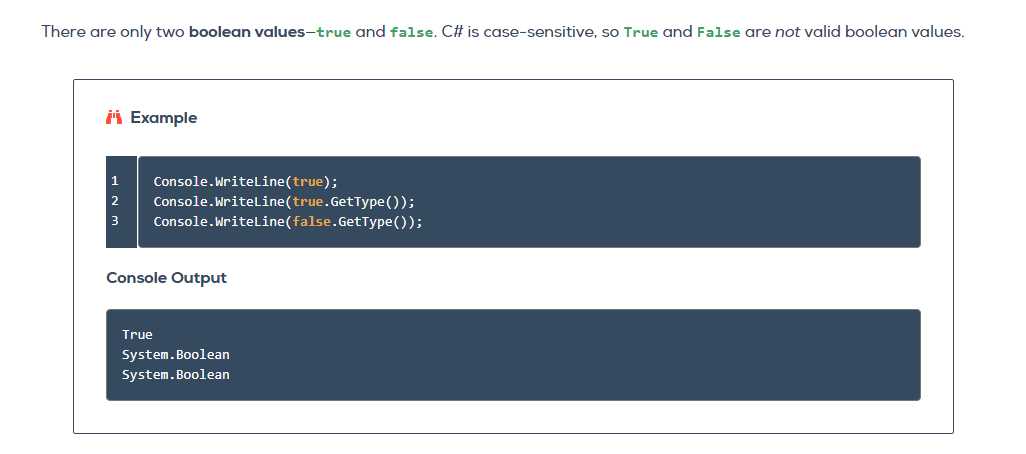
What is GetType()); ?
Is it just saying theyre boolean values?
yes!
Could you use this command for any other data types like int too?
GetType()
is a function available on every type to let you know what it is
yes!
I always have a blank c# console app project open when reading through examples
so I can run their examples and make changes to see how it works
makes learning more interactive, and easier to check these things imoThanks for the suggestion
@JP Sorry to ping again, but is this converting it into a bool data type?
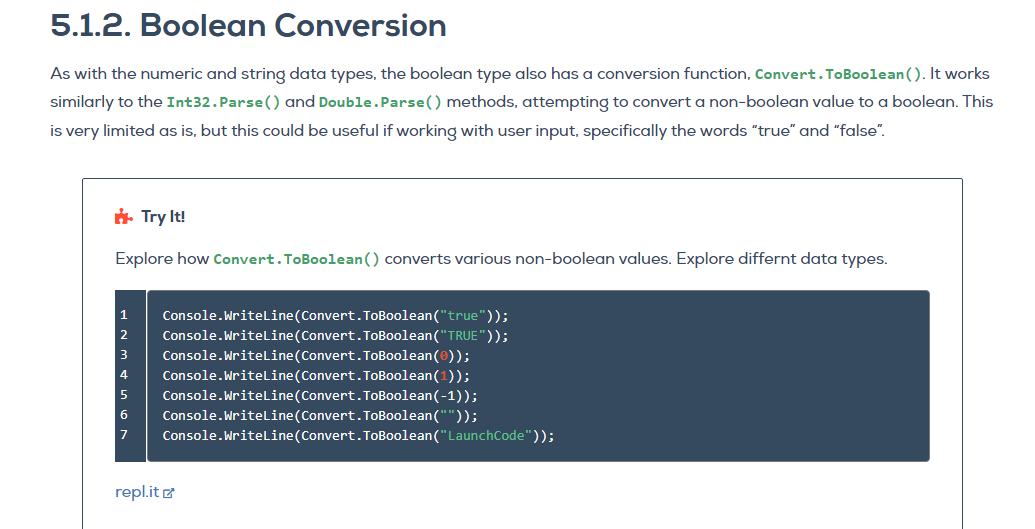
I know Parse converts stuff
Yep, this is another way to do that type of conversion
Convert
is a static class that has a lot of ways to do a variety of types of conversionsGotcha
I was thinking, maybe what he meanto to convert it to a bool was to parse the event I want to occur and then change it to a Bool?
Up until now I have only used Parse commands though
have you ever used
if
statements? (and did you get to the section on boolean expressions
in that article?Sorry if I am being vague I am new to this and have been really struggling for a while
Ya, I am trying to make an if statement to represent the course of action I wanna use
sounds like a good plan
Once again sorry if I sound really dumb or if this is easy I am just figuring it out really sorry
I imagine that is what they meant. Inside an if statement,
if (x > 5)
the x > 5
bit is called a "boolean expression"
you're chillin, dw
so, where are you at right now. Do you have a plan, or still figuring it out?
Feel free to drop your current code here and we can take a lookI can drop my current code
one second
sidenote, this is useful for formatting:
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/https://paste.mod.gg/cmtsumueoivd/0 @JP I inputted here if you wanna see
BlazeBin - cmtsumueoivd
A tool for sharing your source code with the world!
Looks like it would work
looks like ya got cut off:
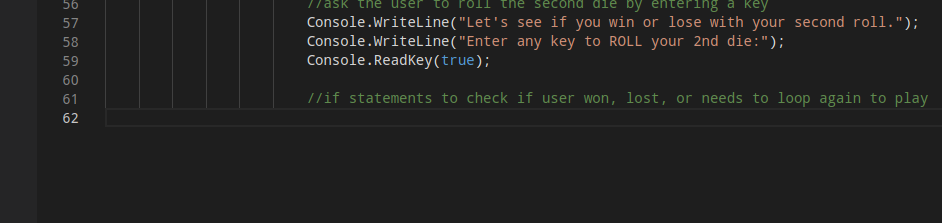
I'm having an issue with my if statements and an actual variable for the events of winning and losing
Pretty much I want the loop to finish or stop if the user wins or loses, but I am not sure how to do that as I thought when a loop end it can only have 1 condition
You can
break;
out of the loopHe suggested I create booleans for those events for the loop
yeah, that's also possible
That's also possible, yeah
Great minds think alike lmao
indeed
I assume that's what you mean
hah, ya beat me. Yeah, this ^
Ya, so for the game it would be loop again if they lost, and the loop would end if they won or lost, and I'd have to writeline a message
then
something
is your win conditionYa that makes sense, what is throwing me off is that I have 2 conditions to end the loop (sorry if I sound confusing I was really struggling)
you can combine boolean expressions with things called logical operators
Ya, I was thinking maybe I ccould make booleans to show that if your dices roll to 7 or 11 you win, or if you role a double, loss
Oh yeah!
these let you say things like
if hasWon AND isFirstTurn
(do something)
in c# AND is &&
and or is ||
And yeah, you can use boolean operators
This makes sense
thanks so much for the help guys i was very lost
i am new to computer science but i really like math so i thought i could try it out
right now its hard for me but its fun and im learning
slowlu
I have a question about this technique
If I have a loop and have a conditional operator for both when the loop ends how would I specify if they won or lost?
The loop would end when the condition is false
It runs as long as the condition is true
store it in a bool?
What would the condition be in this case? If they got neither? The loop would only run if they neither won or lost
The condition can be simply named
run
If they won, set it to false
If they lost, set it to false
If they neither won nor lost, keep it as true
, don't change itSo here, you have a true or false value named hasWon set to false, I'm a little confused though, the loop runs if hasWon is true, shouldn't it be flipped since I want the loop to end when they win
The loop runs as long as the condition inside is
true
You can use boolean operators to your hear's content
You can use !hasWon
to negate it, so the loop runs as long as the player has not wonthe while condition there is a comment, I didn't fill that in for the example
here, let's try this
Sorry if this is another dumb question, but how does the condition run occupy both winning and losing if they are different events?
You can set
run
to false
no matter if the player won or lostthis is how
sorry if this is dumb again, but was it setting run to false really doing in the code?
It makes the loop stop
Because it runs as long as
run
is true
If run
becomes false, the loop stopsAngius
REPL Result: Success
Console Output
Compile: 665.310ms | Execution: 51.054ms | React with ❌ to remove this embed.
Well that was a fast loss lmao
Angius
REPL Result: Success
Console Output
Compile: 575.298ms | Execution: 90.075ms | React with ❌ to remove this embed.
hah
Angius
REPL Result: Success
Console Output
Compile: 601.550ms | Execution: 89.028ms | React with ❌ to remove this embed.
And a fast win lol
Angius
REPL Result: Success
Console Output
Compile: 660.657ms | Execution: 92.428ms | React with ❌ to remove this embed.
Finally it ran more than once 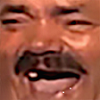
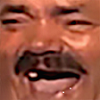
LOL
Okay give me a sec to read and try to understand
sorry guys if im asking repitive questions im just really trying to understand since it is hard for me
i really appreciate you guys taking the time to help \
really tho
It's a help channel, you're supposed to ask questions lol
Starting to make some sense!
so it is like reverse logic
What would a good name for the bool be?
run
seems fine to me
runGame
maybeI have another question, within my if statement, what would be the correct way to represent what happens in a win or lose
For a win in this game, you either get a 7 or 11
I created a variable for the dice
RolledDice
int RolledDice = RandGenerator.Next(1, 7);
Could I say RolledDice = 7 OR 11
using a conditional operator
or hmm
maybe not 11
rolledDice == 7 || rollDuce == 11
it won't roll 11
it has to add to 7 or 11
sorry
Is there a way to track the past 2 dice rolls outcome?
sum == 7 || sum == 11
Sorry again if this is a stupid question, what is sum?
I know what a sum is but is it a special keyword in #
C#
Sum of the two rolls
Angius
REPL Result: Success
Console Output
More...
Compile: 572.403ms | Execution: 89.489ms | React with ❌ to remove this embed.
Oh okay
So I needed to create a variable for sum
Ohhhhh
So I actually needed to create 2 dice variables
oh okay
Dang I didn’t know you could name things DiceOne and DiceTwo
I thought there was a way to track it in one variable
Well, you could just do
directly
Or you could store them in an array
Haha my name is Arrays
I haven’t learned Arrays yet in detail though
Is Sum an int Variable?
Yeah
Here's the same code with explicit types, if that helps
For the double event, would it work to have another variable could double setting them equal?
?
Oh
Sorry
In the game you lose if you roll 2 of the same number
Or a double
rollOne == rollTwo
thenSo like int Double = RollOne =RollTwo?
No, this would be the loss condition
Oh
Would I have to create a variable for that how would that work?
Oh okay
You can, but you don't have to
Thanks for being patient with me I’m learning slowly
One thing I realized is that you used a while loop here and I used a do while loop here, I’m curious on how I could adjust this into still using a do while loop
My teacher had a lab where he made a guess game do the while statement ran the loop only if a condition wasn’t met
Does the while statement of a do while loop always have to have a condition?
The only difference between while and do..while is that the latter will run at least once
Angius
REPL Result: Success
Console Output
Compile: 483.034ms | Execution: 68.235ms | React with ❌ to remove this embed.
Wdym by at least once?
This
Wait, what is happening here?
I see two different loops but only one is outputted
What happened to the while?
The condition was
false
so it did not execute
The condition is checked where you see it, going top to bottom
So while
checks the condition first, then decides whether to execute the code inside or not
In this case, condition was false
from the get-go, so it didn't even execute the loop once
do..while
executes code first, then checks the condition to see if it should proceed to the next execution
So it executed the code, then checked the condition, and since it was false
it decided to not execute the next iterationOh okay
Oh okay
So a do while runs it first
While a while doesn’t
Yep
While checks, then runs
Do...while runs, then checks
Why is it double equals sign btw?
Why do I get an error here?

@JP @ZZZZZZZZZZZZZZZZZZZZZZZZZ any ideas here?
= is assignment
== is equality check
Also I'm a little confused one if I should set my if statement's condition to win or loss or if I should do like, on my do loop for the game
Oh okay
I still get an error

additionally, there is no left-hand side of your second condition
||
can only OR things that evaluate to booleansoh okay
think of it like you currently have this:
(Sum equals 7) OR (___ equals 11)
So Sum on both sides!
exactly!
thank you
I have a question about the Run Game bool i made
should I make it at the end of my while loop
try it!
I'm a bit confused because I worry that if I put it at the end the game will go back to the main menu
Oh okay
see what happens! break things! See how it behaves. then, if stuck, give me a ping
Okay thanks
Is this an okay mindset to have?
works well for me lol
Sometimes I worry if I break things I won't able to fix them or I'm doing it wrong because coding is supposed to be "logical"
I'm 90% learning by trial and error and scattered googling
Ya I have this mindset too i just worry its bad
and reading error messages the compiler spits at me ig lol
the way I see it, if a broke it, you can usually fix it, might just take some time
That's really encouraging to hear lol
unless you're deleting files
righttt
becareful with accidentally deleting things
but that's not what we're doing :P
One thing I realize is that I always get the same two numbers, any reason why? @JP
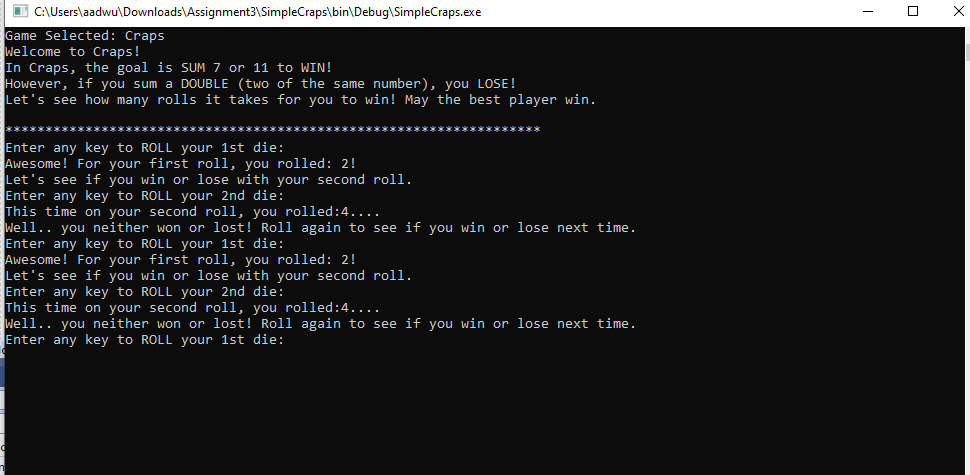
I'd need to see your current code to know
For sure!
One moment
BlazeBin - eehjowfuqzzm
A tool for sharing your source code with the world!
you are doing the rolls before the loop
so you store the values as your first step, then check those same stored values over and over in the loop
you'd want to store those rolled values as the first step inside the loop, not before it
Oh okay!
probably near where you say "Enter Any Key to ROLL your 1st die"
Thanks it worked! I'm really thankful for your help
Have a question but... trying to understand something
fire away
When I get a win or loss, my program just returns to the menu. It doesn't super fast and doesn't show the winning message from the if else statements
e.g you never see "CONGRATS!!! THESE TWO ROLLS SUM TO 11, YOU WON"?
Here’s an example
Yeah!
aha, I understand
so it looks like everything was cleared from the console
do you see anywhere that could be happening?
(sidenote, brb)
No worries!
I see I added a Console.Clear but I’m not 100% how that works yet
Experiment! What happens if you comment it out for now
I realized that the Console.Clear in the if statement after the menu works to clear the menu away, I commented it out, but the menu remains and takes up space while the issue still exists
I have a question about the Console clear, how does it work or on what basis does it clear things? Is it just the ones ahead of it/
that have Console wihin them
note, there is more than one
Console.Clear()
in your app!Ohhh
Yeah
I have one within my menu
Hm it is worth asking why I added that
the other one might be causing your issue 😉
Console.Clear()
will immediately clear anything that was printed before it gets runI have an idea to make the game more fun but I'm not 100% sure how to implement it
I learned in class you can track the number of guesses per round
using the counter
Do you know for loops?
ye
though you don't need a for loop for a counter
at it's core a "counter" is just an
int
variable that you add 1
to every time you want the "count" to go upMy teacher did this
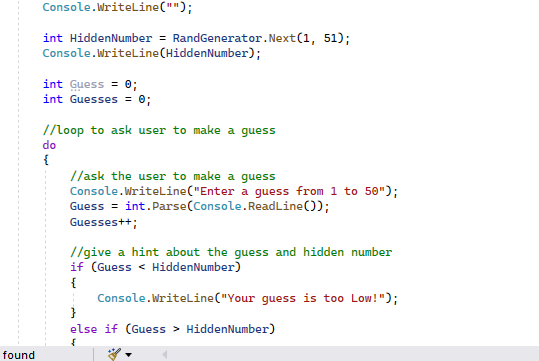
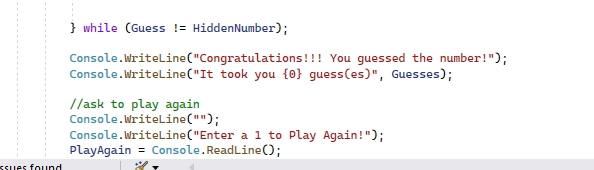
Oh okay
yep, exactly
I see
I want it to count until they get it right or lose
"Guesses" is the
int
, and Guesses++
means "add one to Guesses
"Although my difference is that I don't have an that they check
Maybe I can make a variable for it
Something like Rounds
i GOT THE COUNTER to work
but I have one issue, it doesn;t actually count the rounds
or rather
I don't know where to place it in my code to make it count... maybe after the loop?
When do you want the count to go up?
After the first two dice rolls
put it there!
(the adding to the count bit, not the definition for the count variable)
right
I will put Rounds++;
I ran into this error, where I got the counter not increasing
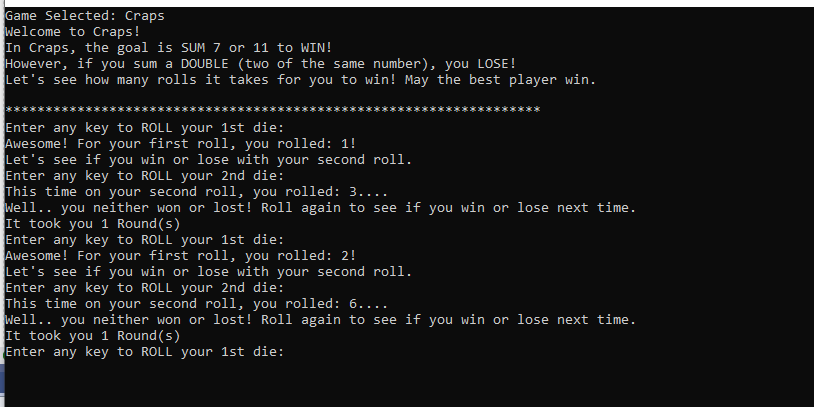
Any ideas?
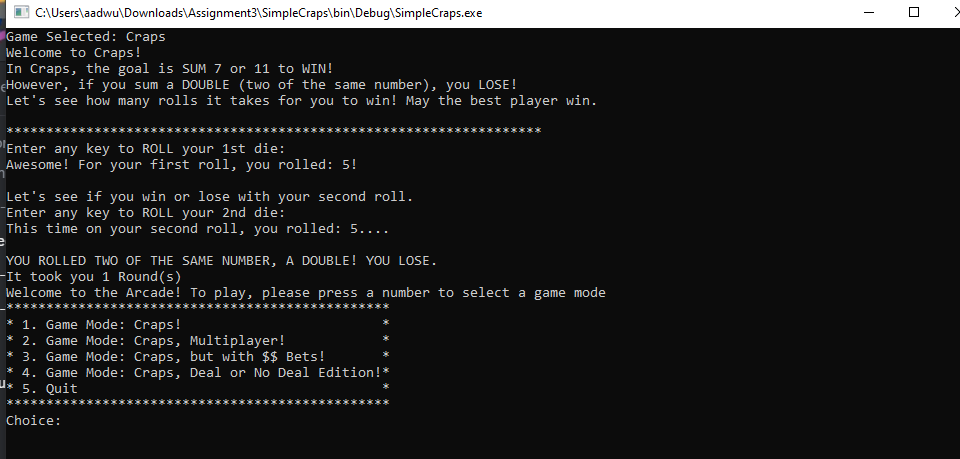
let's see the code!
Sure thing
BlazeBin - bvydpcbtypno
A tool for sharing your source code with the world!
alright, looking
so, what happens in the first four lines of the dice-rolling loop, every time it runs?
what does the computer do
(in broad terms)
I declared it’s variables, so it understands dice 1 and 2, sum and rounds to count
So I gave RolledDiceOne a datatype of integer so it is a number
And gave it to the random generator
Similar for rolled DiceTwo
And for Sum I gave it a combination of both
Rounds I gave 0 so it starts at 0
all true
what happens on the second iteration of the loop?
if unsure, just say, I can explain
The second loop is to ask them to input something to play, and then give them a result
When I say "the second iteration of the loop" I mean this same loop, the second time it runs
Oh
and the question was kind of a trick
Doesn’t it loop again?
the answer is "the same thing that happened the first time!"
exactly, yes!
Yay
so, notably, it would get reset to 0
but there is actually a second part
do you know what "variable scope" is? Fine if not, can explain quickly
Ya that makes sense, would it be possible to track it still even if it loops? My teacher did but I’m not sure how ours is different
Not sure
Did it here
I'll explain, "variable scope" plays a role, and its a good thing to know
Oh ok
Thanks
so, "scope" is a term used to describe where a variable lives, and where it can be accessed from.
"Blocks" (the areas in your code surrounded by curly
{
braces }
are the primary thing that will determine the scope of a variable.What is a variable scope?
example, because a picture is worth a thousand words:
any questions about this ^?
feel free to toss in your IDE to see what errors it gives you
One second let me it through sorry
you're golden, take yer time
Probably a dumb question but wdym by var?
Like datatype?
oh, var is a shortcut telling c# to guess the datatype
in this case, it guesses "string" because the value on the right is a string literal (in other words a value between quotes like that is always a string)
so it would be the same as me writing
string
in this case
I'll change it to that to be more specific!Oh okay
any questions about variable scope or the example above?
What is being done with the myusername variable?
string myusername = john paul e
And “fine”
in what sense?
Are you asking what the code written is attempting to do, or what happens behind the scenes when the scope ends
Attempting to do
that's just a comment I included to note that the next line will not error
Oh ok
Do they mean the same thing?
it's... being logged? Is that what you're asking?
"they"?
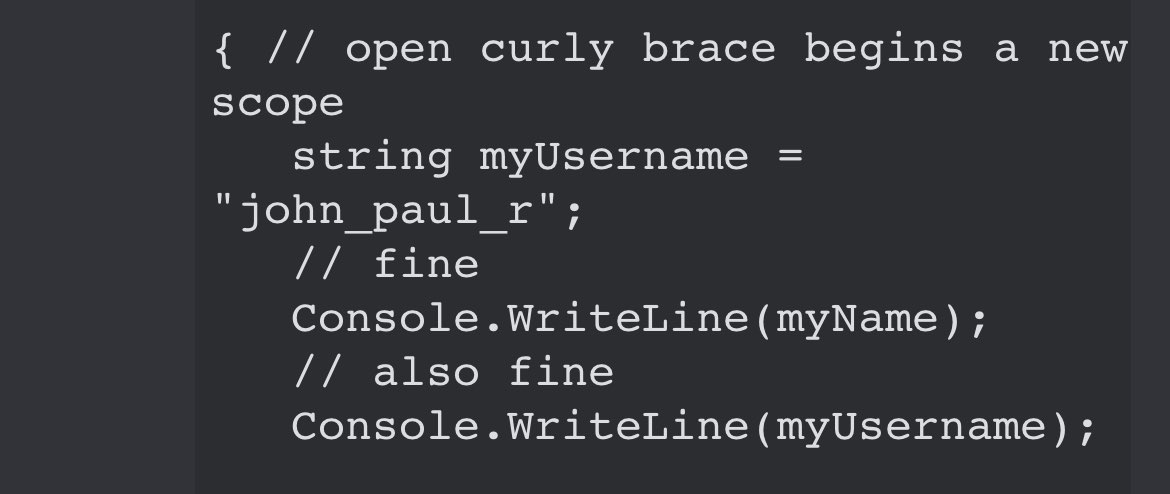
there are 6 lines to choose from there :P
idk which you're referring to
Do all the lines that aren’t header comments relate? Or are you just showing it
I thought writeline needed “
they do all relate, they are all one small program, yes
Console.WriteLine
can write any string
strings can be stored in variables just like ints and bools
and you can pass the variable that contains the string to WriteLine
, and it will get the value contained within
Just like you could do
or
and get the same resultOh okay
here is an example of the output, and it's interactive, so you can fiddle around
if no immediate questions, I can relate this back to your program's count problem
Hey,. i’m sorry I left yesterday, I feel asleep
Ya, this makes sense
It's like different ways to write the same thing as long as within the brackets
I'm thinking my counter has to be within the lines of code containing where I want a round to be quanitied, and make sure it's within the brackets
@ZZZZZZZZZZZZZZZZZZZZZZZZZ you helped me alot yesterday, any chance you can take a look at this too?
Making I should put the counter at the bottom
I tried this but it didn't work, I also added a play again function but I think I implemented it weirdly, I only want it to say that if a user wins or loses, not after the loop, so I'll put it in the statements where the user wins/loses
BlazeBin - szpnjayemhit
A tool for sharing your source code with the world!
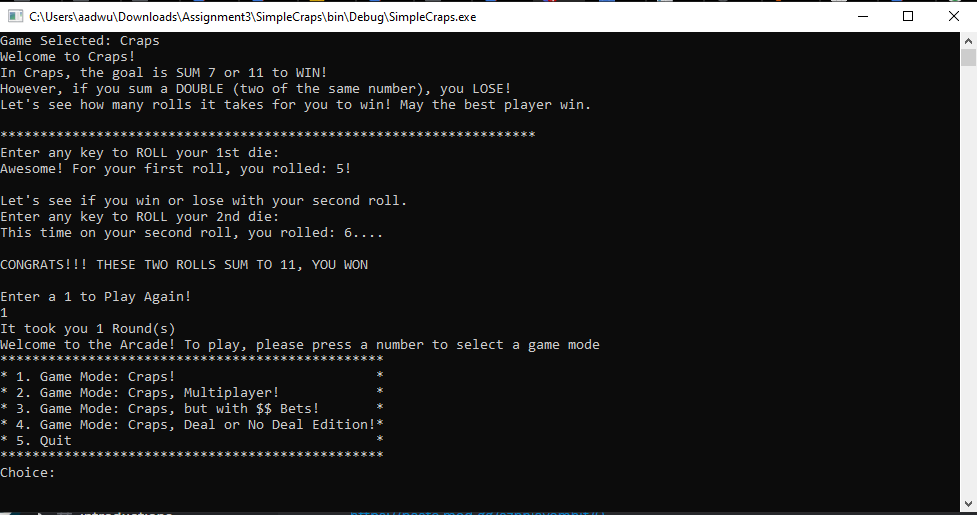
One of my issues is that the play again doesn't recycle the loop, which makes it a bit hard, not sure how to fix that
As you can see here I entered 1 but it just makes the whole thing restart which is wrong
What do you mean "recycle the loop"?
Sorry, I meant loop again
I want it to be that if you press 1 it loops back to the two dice rolls, given you win or lose since the hame ends @SinFluxx
Sorry if my wording is wacky
Also thanks for even trying to help
@ZZZZZZZZZZZZZZZZZZZZZZZZZ any chance you can help me out now if you're back online?
I can try
YAY
thanks so much for responding
I am very grateful
omg
So, if I'm not mistaken, you want to restart this particular game, not the whole application?
Yes!!
Meaning, you don't want to go back to game selection, you want to re-run the game of Craps, Multiplayer for example?
Right!
I want them to play again after they win or lose
If so, the best way would be to enclose it in another loop
Oh okay
And with the code growing more complex like that, you might consider splitting it into separate methods
I haven't learned about methods yet
Ah, all in due time, then
Amen!!
I think it is our next unit
in class
I am very beginner so sorry if I am lost a bit
but the help is thanked
it takes time
So, would I just made another do while loop within the do while loop?
Yep
Ok, I will try this out
I'm gonna place it above the if statement for the first game
Something like that, basically
One overarching game loop, and smaller loops one per individual game
Gotcha!!
@ZZZZZZZZZZZZZZZZZZZZZZZZZ is it okay if I send you a link of my code? im stuck on where to start the do while loop within the other one
i tried to frame it like this but it kinda broke up the brackets
i tried to put it over my if statement but it doesnt really get the code in
ill send you a link
Sure
BlazeBin - vosdoaldryun
A tool for sharing your source code with the world!
I tried to put it above the line where it says "if menu == 1"
Any suggestions on how I should try it
I feel like alot of the time I do get the idea
but I just really struggle implementing it without alot of errors or even like near breaking the whole thing
Looks fine
Ya, but I didn’t implement the do while loop within it
I’m not sure where to put it or which line
Without messing the other one within it
I see you do have the inner loop
What exactly is the issue with it?
Ya but I removed the functionality to Play Again
The press 1 to play again
RunGame && PlayAgain == "1"change this to
RunGame && PlayAgain != "1"
I want to add that
But I want them to press 1 to playagain
oh, apologies, misreaed
Ya
It should just automatically play again unless you set
RunGame
to false
I want to implement it so that if they win or lose they press 1 to play again
So, check the input while inside of the inner loop
before terminating the loop,
Would that be the while statement?
Like the condition of the do while
That'd be a minimal example
try it, I think that also works, yes
Oh okay
That looks like JP’s
Too
the ||` is the meaningful bit
Is it correct to only ask them if they win or lose by putting it under the if and else if statements for winning and losing?
Just making sure Imm doing it right
You can, sure
Also question, why do we set RunGame = to itself, and why is it and OR statement instead of AND?
because, in the case where you neither won nor lost, you never asked them if they want to continue
you presumably just want the game to continue going if
RunGame
is already true
because they've not won/lost yet
Another way of writing this would be:
point being, we only care about PlayAgain
if RunGame
is false
and RunGame
is only false
if they've won or lostWhat does the statement RunGame = RunGame by itself mean?
on its own that just assigns the value currently stored in
RunGame
to RunGame
. So it effectively does nothing
as part of the larger expression, however:
if RunGame
is already true, it'll never be set to false
by this statement, because of the ||
because true || false
-> true
Why does true or False make false turn to true?
read it like this:
are
true OR false
true? if yes, return true
, else return false
that is what true || false
means
similarly, true && false
asks: are true AND false
true?
If yes, return true
, else return false
so true && false
yields false
, since that question is not satisfied.“Are true or false true?” what do you mean by true (the one bolded)?
I mean literally the value
true
true
is true
. false
is not true
.Oh okay
Only true is true and false is not teue
True
Ok i think that makes a little bit more sense
Its checking if its true
Dont these do the same thing then?
?
elaborate
Both of them check if true or false matches the value true, and if they match it returns true, if not it returns false
are green and red green?
No
are green or green green?
Yeah
tada
that's the difference
Oh ok
What happened to red
I realized its and vs or though
ah, gz I goofed my example
restarting:
are green and red green?
noare green or red green?
It can be green, for and it cannot be green only because its both
i have no idea what you just said lmao
Looool
generally, AND needs all to match
Im saying that green and red cant be just green because its both
OR needs ANY (at least one) to match
Green or red can be green sometimes
But technically it can be red too
Oh okay
welp, I declare the example bad :P
That makes sense then
Well i get what you’re saying
i'll have to get a better one next time lol
It just needs one to match
si
U have no idea how much i appreciate this though 😭😭
At least i am starting to understand the logic a bit
Gonna try running it but I implemented this
This happens
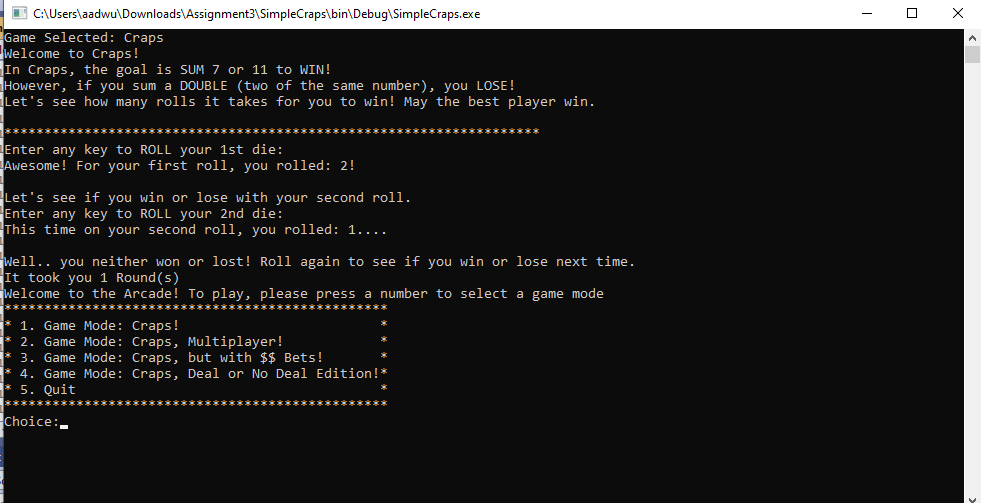
So this is kinda bad because instead of looping again to roll, it brings the menu
Let me try again to see what happens if you win
@JP any ideas why this may happen?
send code!
Sure thing
BlazeBin - gmgphwfrdich
A tool for sharing your source code with the world!
Anything jump out?
no.. this is odd
experimenting
Gotcha
I have a suspicion
move
PlayAgain
right before the while
condition
er, actually, right after the innermost do
, forgot you read that in earlier in the loop
point is, it needs to fall out of scope when the inner loop restartsBy PlayAgain, which line of code are you referring to specifically? Is it String PlayAgain = string.Empty?
I'm thinkin something like this
though I'm suddenly not 100% sure that's the problem, checking..
you are also not doing
in the
Sum == 7
branchOops
Thank
Fixed this
That may have fixed it, will try it out
IT WORKED
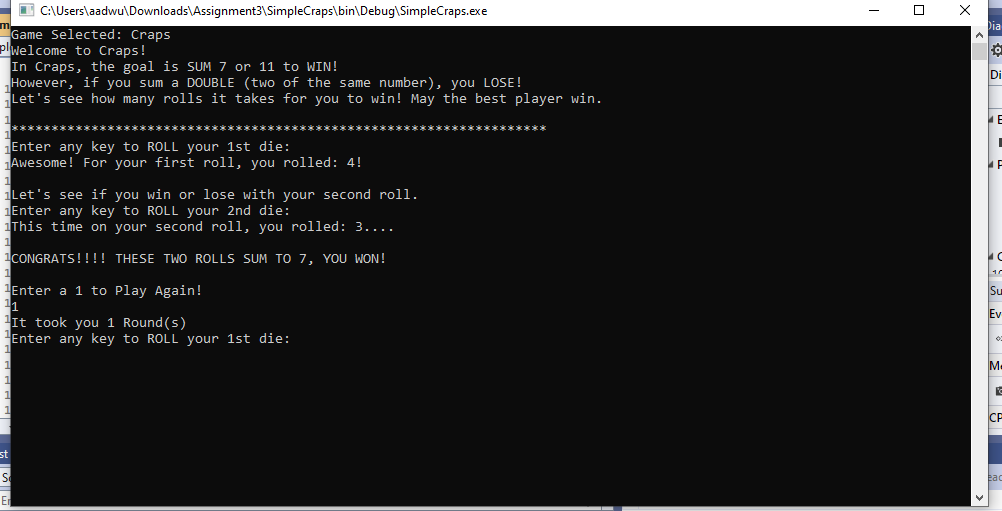
wow
well
let me try again
I think that fixed it lol
I think the only thing left to fix is the counter and the formating
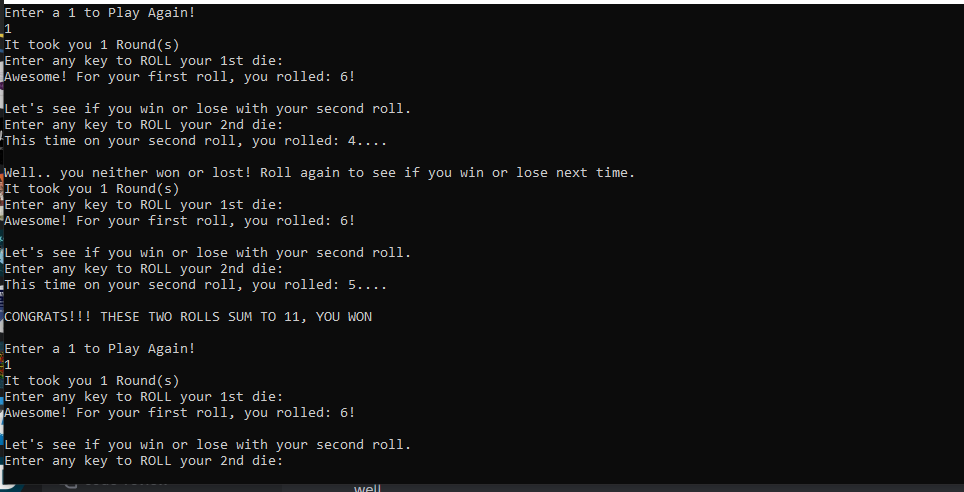
I think a good idea is to clear the prior results
As in like if you get neither win or loss, after a round is counted, it clears it off the screen
thank you so much jp
ill be back in a bit i need a break
Alright I'm back
Thank you so much for helping me out guys @JP @ZZZZZZZZZZZZZZZZZZZZZZZZZ
I just want to do something I think will be a bit more minor but yeah
I want to fix the counter, it's kinda buggy, it doesn't increment by 1 like I want it to
it seems like it's always stuck at 1
Anytime
Thanks so much
any idea on how to make the counter fixed?
I remember yesterday JP was talking about scopes
maybe I should put it within the bracketed if statements?
I want it to increase by 1 every time 2 rolls happen
or at least output that at the end, for some reason it doesn't work
it just goes up by 1
it just stays at 1*
any ideas on how i can fix this?
@ZZZZZZZZZZZZZZZZZZZZZZZZZ dont mean to double ping but you were jus here
$scopes
thing a
is available in scope A
and scope B
thing b
is available only in scope B
If you want something to persist outside of some given scope, it needs to be defined outside of that scope
BlazeBin - oitamnrieqew
A tool for sharing your source code with the world!
I put the counter after the if statements and made the int for it above it
both of them are in the loop though
im confused why its not increasing
If it’s okay, can you check if everything is placed correctly? I assumed the do while loop is the “scope” here
Declare the rounds outside of the loop
With every loop, you create a new variable
rounds
And set it to 0
When you say declare the variable, how do I declare it without setting it to 0?
Angius
REPL Result: Success
Console Output
Compile: 641.111ms | Execution: 53.360ms | React with ❌ to remove this embed.
Declare it and set it to 0
But it has to be before the loop
I tried that,got this error
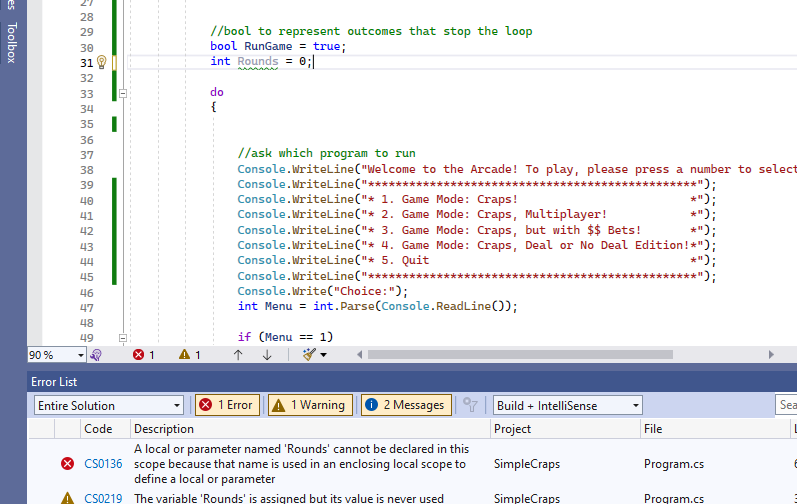
Means you have a
Rounds
variable somewhere already
Somewhere in the outer scope, specificallyI see
Look at this for exmaple
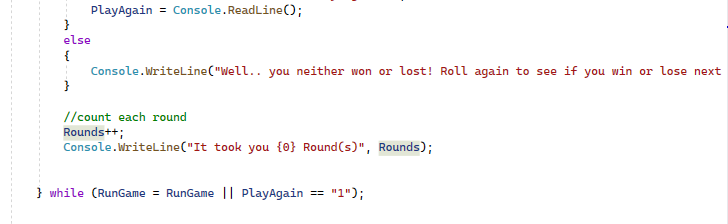
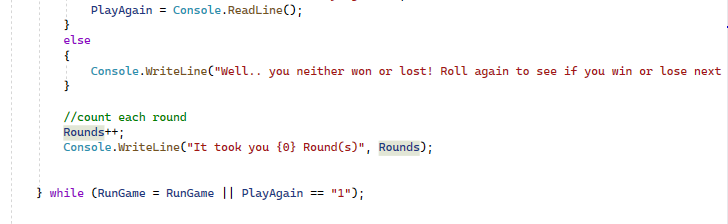
I tried to mimmick what you did here
Ooosp
Seems fine
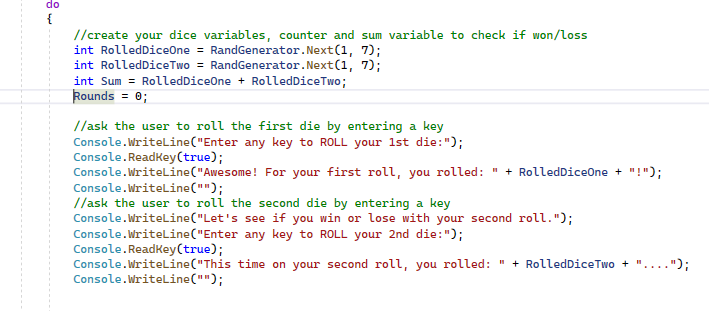
So I decleared it outside the loop, within the loop set it to 0, then made it count by 1 each time
I'll try running it to see if it counts
Angius
REPL Result: Success
Console Output
Compile: 630.047ms | Execution: 93.792ms | React with ❌ to remove this embed.
Oh it seems I made an error
I have to put my counter inside the loop?
the Rounds++?
ok, ill try that
well actually im confused
@ZZZZZZZZZZZZZZZZZZZZZZZZZ I'm a bit confused where I should put my counter
like its still within my loop and i ran it it doesnt increment by 1
Look at my example
The counter is outside of the loop
And it gets incremented inside of the loop
I’m a bit confused because I don’t have a for loop. I just have an Rounds++ variable
Maybe I can send you my code again?
@ZZZZZZZZZZZZZZZZZZZZZZZZZ @JP https://paste.mod.gg/dpmedmelnbfo/0 Can you guys check out where I messed up ?
BlazeBin - dpmedmelnbfo
A tool for sharing your source code with the world!
I put it outside and it still didn't function sadly
You have a loop though
Place the
rounds
variable nearest to the loop whose iterations you want to count
No need to place it this far out
Unless you want to count total rounds of all the games the player played, then sureI'm a bit confused though, is it not in the do while loop ?
the Rounds++: counter
Like it is within the do while loop
is it supposed to be in the if else statements you mean?
You have the rounds variable declared in front of your big loop
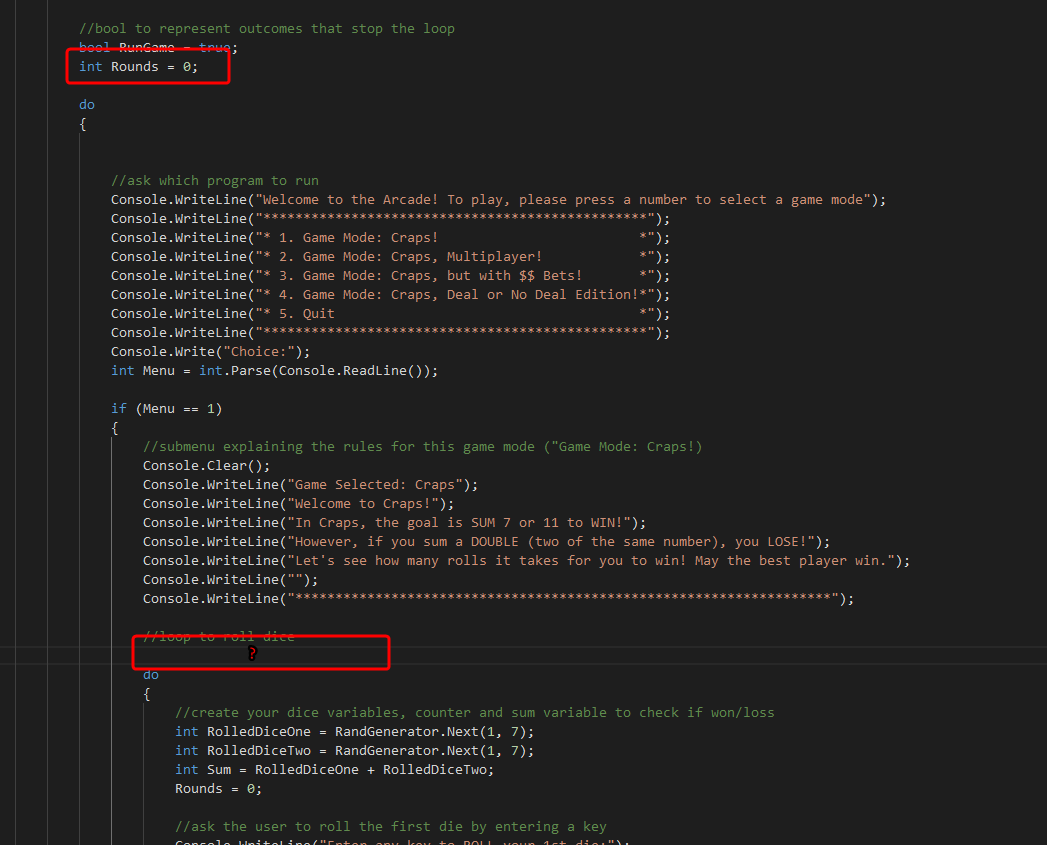
Is it supposed to be there?
Instead of in front of the small loop?
So that each game can count rounds separately?
Or do you actually want to count all rounds of all the games played?
If so, carry on
But then don't reset the rounds count to zero with every inner loop iteration
I want to count how many rounds it took in total til they won or lost, yea
Ohhh I get what you're saying
my bad
I want it to be seperate
Wondering, why does it matter where I declare the variable?
Here's another thing
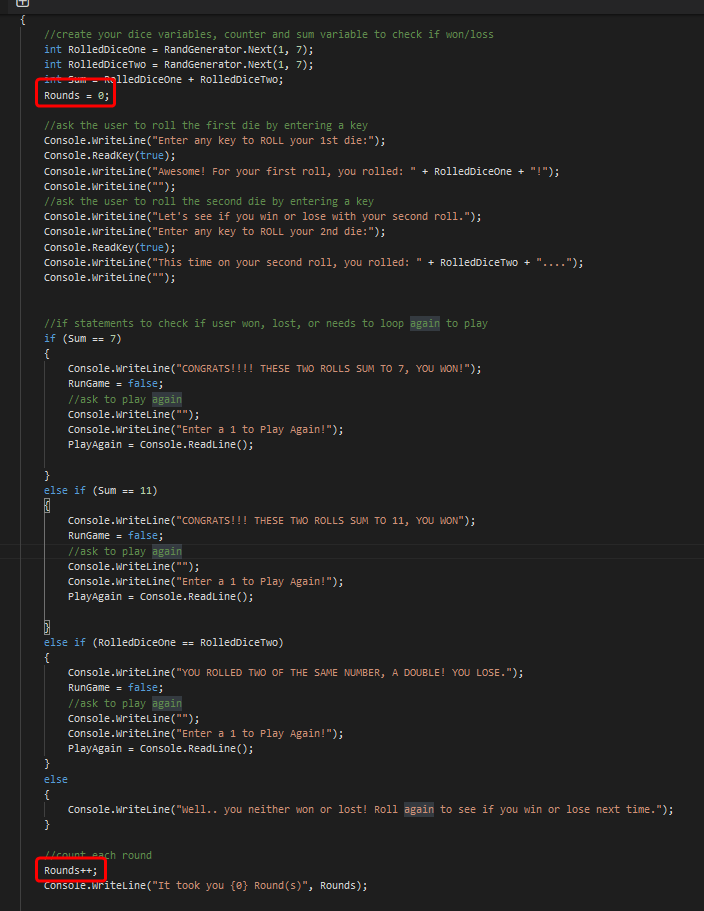
You increment the
Rounds
at the end of the loop
But at the start you reset it to 0 again
It can never increase
Because you reset it each timeHow should I fix this?
Move the variable outside of the loop and don't reset it
wdym by dont reset it ?
You're doing
Can you see how with every loop iteration the value of
rounds
will be reset to zero?ya
because when it ends it resets to 0
Do this:
So the rounds++; counter is supposed to be within my if else statements, right?
Within the loop
You're counting the iterations of the loop
Your increment is where it should be
But you also reset the
rounds
value with each iteration
Don'ttest
```cs
//if statements to check if user won, lost, or needs to loop again to play
if (Sum == 7)
{
Console.WriteLine("CONGRATS!!!! THESE TWO ROLLS SUM TO 7, YOU WON!");
RunGame = false;
//ask to play again
Console.WriteLine("");
Console.WriteLine("Enter a 1 to Play Again!");
PlayAgain = Console.ReadLine();
Rounds = 0;
}
else if (Sum == 11)
{
Console.WriteLine("CONGRATS!!! THESE TWO ROLLS SUM TO 11, YOU WON");
RunGame = false;
//ask to play again
Console.WriteLine("");
Console.WriteLine("Enter a 1 to Play Again!");
PlayAgain = Console.ReadLine();
Rounds++;
}
else if (RolledDiceOne == RolledDiceTwo)
{
Console.WriteLine("YOU ROLLED TWO OF THE SAME NUMBER, A DOUBLE! YOU LOSE.");
RunGame = false;
//ask to play again
Console.WriteLine("");
Console.WriteLine("Enter a 1 to Play Again!");
PlayAgain = Console.ReadLine();
Rounds++;
}
else
{
Console.WriteLine("Well.. you neither won or lost! Roll again to see if you win or lose next time.");
Rounds++;
}
oops
@ZZZZZZZZZZZZZZZZZZZZZZZZZ is this how it is supposed to be?
You forgot the closing backticks
thanks
Is this supposed to reset the counter when the player gets 7?
@ZZZZZZZZZZZZZZZZZZZZZZZZZ I fixed the 7 thing
that was an error
well discord still kinda messed it up
You still reset the
Rounds
back to zero at the start of the loopbut i want to increment after every 2 rolls
What should I set it to?
?
It should not be set at all
oh
Declare the variable with the value of 0 BEFORE the loop
Increment the variable INSIDE the loop
Do not change the variable IN ANY OTHER WAY inside of the loop
that's all
Right so I set it to int Rounds = 0; before the loop
Okay I'll try running it
@ZZZZZZZZZZZZZZZZZZZZZZZZZ thanks for your paitence with me
I noticed it says 2 rounds, which is nearly right, but I'd want it to say 1 round since the user won immeditaely
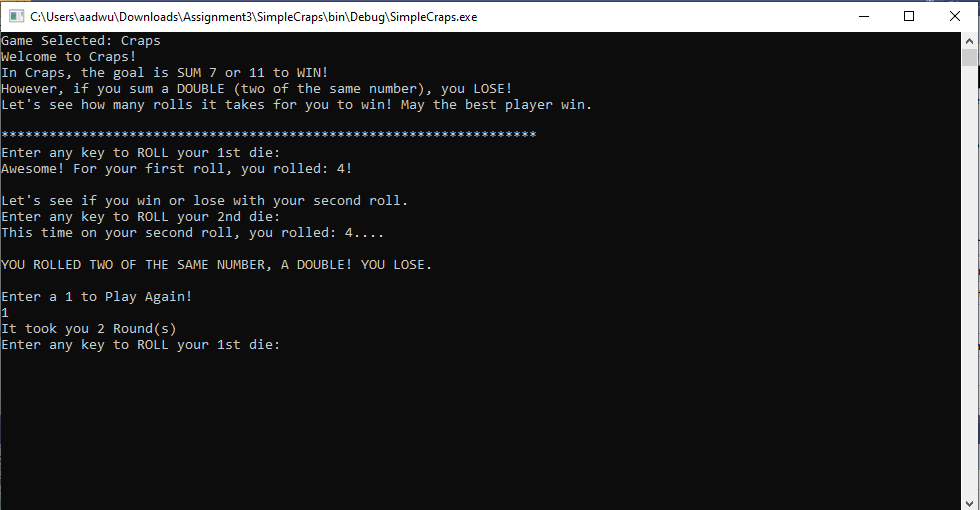
I should also probably remove the counter on losses, only keep it for wins