Setting up oauth API
my dashboard is hosted on https://cardinal.oreotm.xyz
and my backend is hosted on a vps on [ipaddress]
Backend Setup:
Frontend Code:
When i host the frontend on my local machine the login data is returned, but when i host the site on the domain it returns 'Bad request'. How do i properly set the api up?
Solution:Jump to solution
something like
http://207.xxx.xxx.xxx:4000
could it be because im mixing http and https while using credentials: 'include'
...56 Replies
have you checkedo out https://www.sapphirejs.dev/docs/Guide/plugins/API/using-oauth2-backend-route and the accompying frontend page? You also seem to have copied code from @Skyra seeing as you also have the
transformOauthGuildsAndUsers
, keep in mind that Skyra is still using @sapphire/framework v2. Furthermore, that bad request ultimately gets returned from your own host after all so you should be able to view a reason why, i.e. by using debugging breakpoints.Sapphire Framework
Using the built in OAUTH2 route (backend) | Sapphire
If you are using localhost as your test environment, make sure you use http//localhost in
yup i first copied that when i was only running it on localhost and everything worked fine but the cookie didnt set, and later i tried to host it on the domain and i got the bad request error. So i decided to search for solutions in this server and i saw someone having the same problem so i copied their config, but it still didnt work.
Nothing gets logged on the backend. I remember it used to log stuff like
invalid_grant
and invalid_redirect_uri
but now it doesnt log the error at all. The most weirdest thing is that it returns the login data when in on localhost but not when im on cardinal.oreotm.xyz
okay so i made some changes
Now i get this error in the backend
when i make the following request from the front end
but when i make the same request with the redirectUri set to https://cardinal.oreotm.xyz/oauth/discord/callback
on the front end
it doesnt log anything in the console and login data is returned as bad request
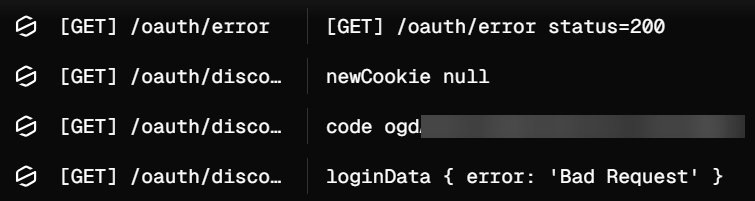
alright so i looked at https://discord.com/channels/737141877803057244/1111812366296948736/1111812366296948736
and the only thing i can see different is that his api is hosted on a subdomain of his bots dashboard domain, will that be an issue in my case? since im just making an api request to the IP:PORT of the vps im using my bot on, i dont really now how to make
api.oreotm.xyz
be pointed to my bot so yeah
Ok i have an update
Im using this to make the request
on both localhost and the actual website hosted on vercel
and i can assure that the redirect uri is correct
on prod: https://oreotm.xyz/oauth/discord/callback
on dev: http://localhost:5173/oauth/discord/callback
But when i click the login button on the vercel website i get
But on localhost i get the full login data with the transformed guilds and everything
And on the backend (bot) i have hardcoded the api config as so
And i have changed the production website url to be https://oreotm.xyz
since i thought the subdomain might be messing up the oauth
So why is it that on localhost it returns the logindata but not on the actual production site?
You can ignore the previous messages and start reading from hereDid you setup the redirect URL on the discord bot dashboard?
Discord Developer Portal
Discord Developer Portal — API Docs for Bots and Developers
Integrate your service with Discord — whether it's a bot or a game or whatever your wildest imagination can come up with.
like this
also if you're using the built in oauth callback endpoint from the api plugin then it's
/oauth/callback
, not /oauth/discord/callback
yup
even on the frontend?
because im calling the built in oauth api correctly
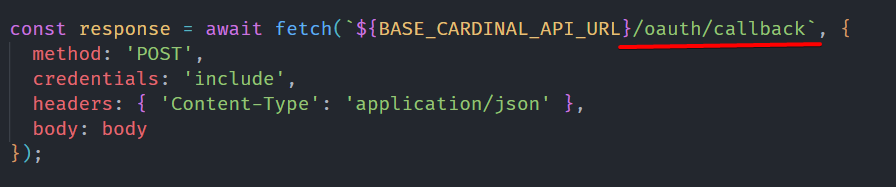
unless u mean on the
redirectUri
in the body that it should also be /oauth/callback
anyhow i changed the frontend oauth link from /oauth/discord/callback
to /oauth/callback
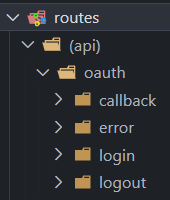
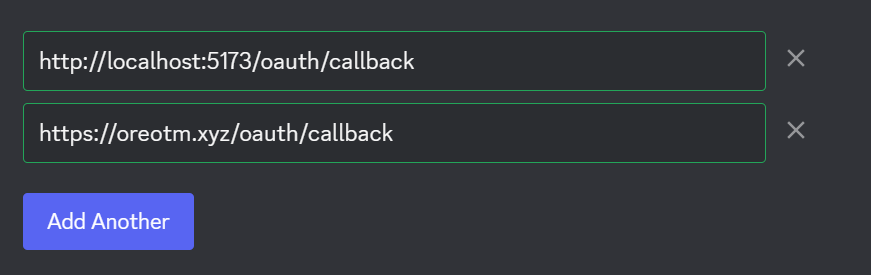
this yes
okay i did that, and i made sure it worked by console logging the redirect uri here

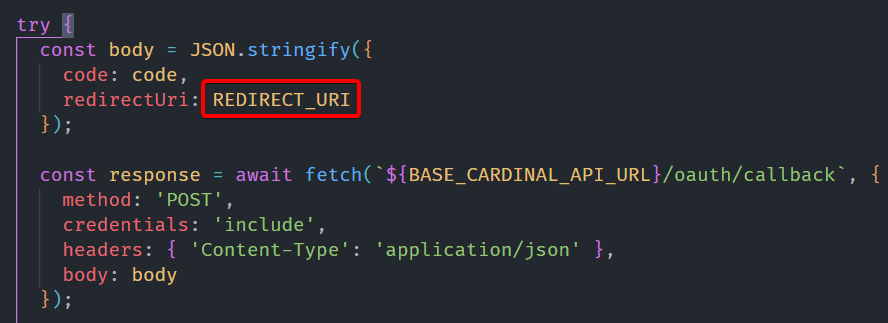
still i get this error
{ error: 'Bad Request' }
i checked the sapphire/plugin-api src code and it returns
Bad Request
when the code isnt a string, i think
but i checked the code and its there
I have no idea then. You could leverage yarn patches /
patch-package
(for npm/pnpm) to add console logging.. or use VSCode Remote Server to make it so you can set a breakpoint on your prod run.
problem with this kinda stuff is that it's so heavily environment dependant that I cannot do much for you eithermaybe the bots config?
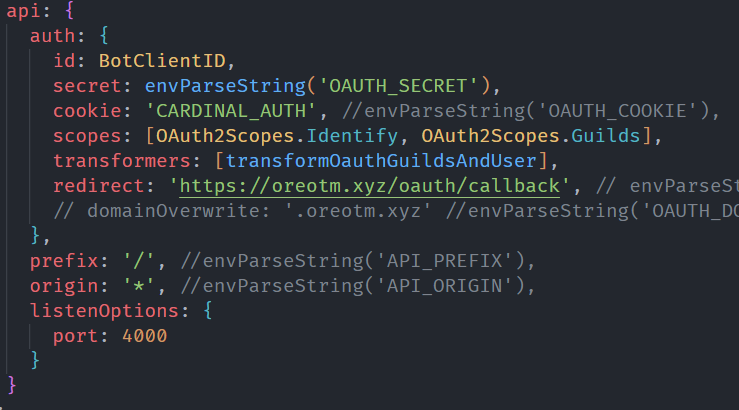
if not ill go back to the oauth system i had made before, i just wanted to use sapphires one bc of the
LoginData
objectwhat kind of frontend do you use? next? react? vue?
svelte
is that the problem? but i saw someone else use svelte with sapphires/plugin-api too
ah I dont have experienc with that but if you say the code is definitely being sent then you're probably right
nah it shouldnt be
you logged the body after stringifying it and before sending it?
yup
localhost:
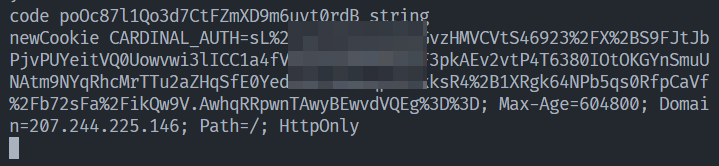
for @Skyra the config is as follows
Frontend
Step 1
- User clicks the button to login. That button has the URL:
https://discord.com/oauth2/authorize?redirect_uri=https%3A%2F%2Fskyra.pw%2Foauth%2Fcallback&response_type=code&scope=identify+guilds&client_id=266624760782258186
- This URL gets build up here: https://github.com/skyra-project/skyra.pw/blob/main/src/utils/constants.ts#L9-L14
Step 2
- https://github.com/skyra-project/skyra.pw/blob/main/src/pages/oauth/callback.tsx
- The user gets sent back to this page by Discord after they authorise. We receive the code from Discord and then send the request to the api plugin route. We provide the SAME url as the current page as the redirect uri before so React doesn't navigate us away thus losing our state, instead we just end up receiving the data and processing it.
- redirectUri
: ${BASE_WEB_URL}/oauth/callback
==> redirectUri: https://skyra.pw/oauth/callback
(this is a FRONTEND route! Not a backend one which would be https://api.skyra.pw
)
- clientId
is @Skyra 's client id. A variable here because we use dev bots for dev. You could hard code it to your bot's client id.
- code
as you know, parsed from the querystring.
Backend
- https://github.com/skyra-project/skyra/blob/main/src/config.ts#L46-L69
- Also see image below.
- The domain overwrite is because we have the backend on api.skyra.pw
and the default for the domain would be skyra.pw
which then has the cookie not match. If you host your backend on for example https://oreotm.xyz/api
through routing then you don't need the domain overwritehope that helps
ok so since im hosting the api on a vps that doesnt actually have a domain but instead i just connect to it using its ip i dont need
domainOverwrite
and what does API_ORIGIN actually do?
and my setup is similar to skyras setupso you're saying that BASE_CARDINAL_API_URL is an IP address?
yeah..
with the port
that the api is configed to listen to
as for API_ORIGIN, that sets the
Access-Control-Allow-Origin
which defaults to *
Solution
something like
http://207.xxx.xxx.xxx:4000
could it be because im mixing http and https while using credentials: 'include'
uh yeah you should definitely only use https
You can use cloudflare are your dns even when using Vercel (that's literally what https://sapphirejs.dev does too) and then you can get free SSL
Sapphire Framework
Home | Sapphire
Sapphire is a next-gen Discord bot framework for developers of all skill levels to make the best JavaScript/TypeScript based bots possible.
as well as a free subdomain that you just point to a different IP address
different A records
yeah the domain is using cloudflare dns
but i couldnt get the api.oreotm.xyz to be pointed to this IP bc of ports and stuff
wait ill try something
api.oreotm.xyz
point it to 207.xxx.xxx.xxx
, dont add a port on cloudflare.and it will get requests from 207.xxx.xxx.xxx:4000 ??
I mean you'll have to use nginx on the VPS to expose the localhost and port
yeah ill try that
on nginx you configure
localhost:4000
to go to api.oreotm.xyz
and then on CF you configure the same to go to the IP address
btw just my 2 cents but if you're using CF anyway then why use Vercel?
I mean if you want GH integration you can use CF pagesidk how else to host my sveltekit site
im new to this frontend stuff
Deploy a Svelte site · Cloudflare Pages docs
Svelte is an increasingly popular, open-source framework for building user interfaces and web applications. Unlike most frameworks, Svelte is …
I've never used Svelte/Sveltekit before mind you so I can't be much help other than giving that doc site
but I know it should be possible
FWIW this wouldn't necessarily alleviate issues with all the OAUTH stuff
Just that it's a bit wacky to use 2 platforms when 1 suffices
true
ill look into that
thanks for ur help
@Oreo ™ Here's a SvelteKit implementation you can check if it helps, though I haven't touched it in a while: https://github.com/KBot-discord/KBot/blob/main/apps/web/src/routes/(api)/oauth/discord/callback/%2Bserver.ts
funnily enough i was following your github
https://discord.com/channels/737141877803057244/1183099327816671242/1183372987953582111
Oh I didn't notice lol
Also i know this is not related to sapphire but are you able to link me a resource to link
api.oreotm.xyz
to 207.xxx.xxx.xxx:4000
with nginx? i searched around and found like 3 videos telling me to do 3 different things. One tells me to create a file called api.oreotm.xyz in conf.d/ and another tell me to edit a prexisting file, and none of them seems to work.
right now im using cloudflare tunnels
i mean it works
¯\_(ツ)_/¯
this goes in
sites-available
and then you symlink it to sites-enabled
thanks so much
so ultimately it was just exposing it through a subdomain instead of ip?
yup