How to use toggle column to update pivot table?
I have a many-to-many relationship with product, user, and product_user_fav models, and I want to create a favorite toggle column in the products table to update the product_user_fav table (pivot).
That is when a user switches the favorite toggle column in the products table if there are no user_id and product_id rows in the Product_user_fav table, a new row is registered in the pivot table, and if there are user_id and product_id, the existing row is removed.
How can I do this? Thank you!
#user model
#product model
#product_user_fav model
#produts table
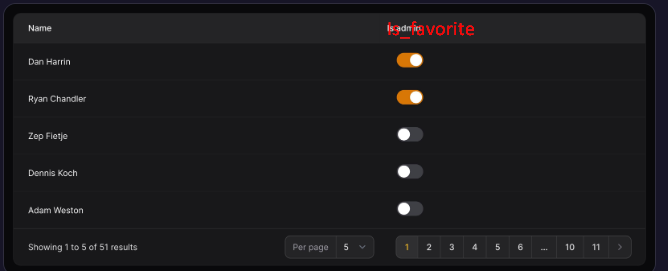
42 Replies
Same request
you can use the
->updatStateUsing()
method to alter the behaviour
for example I have the email_verified
on my Users
table toggle like that
You can do something similar for your case maybe?this work on same table, my date are on pivot table like user.posts.0.email_verified
🤗 🙏
I will try it.
no i want say it didn't work ahah sorry
Can you share the whole table? What record is this on?
NVM the table is there
I don't understand what you're trying to do here. Is this table inside the Product view? So when you're looking at a procuct, you can also select the users that have favorited it?
Ok, I will share all things, currently, the pivot field is not working in table toggle column.
This is Product model
This is user model
This is Product resoruce.php to show the products table.
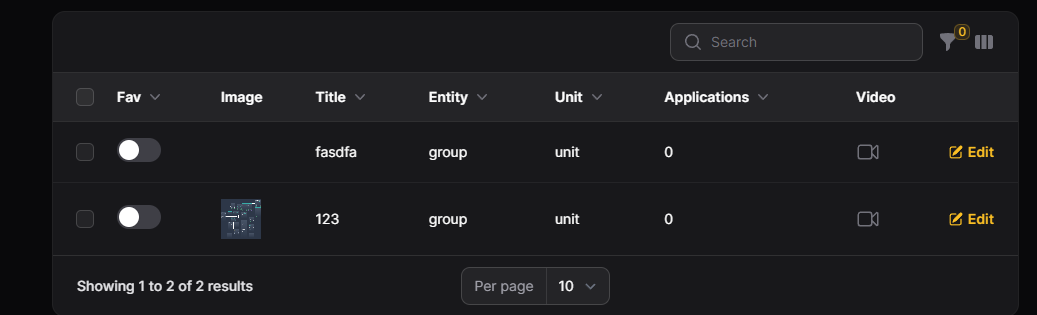
When toggling, I got below issue:
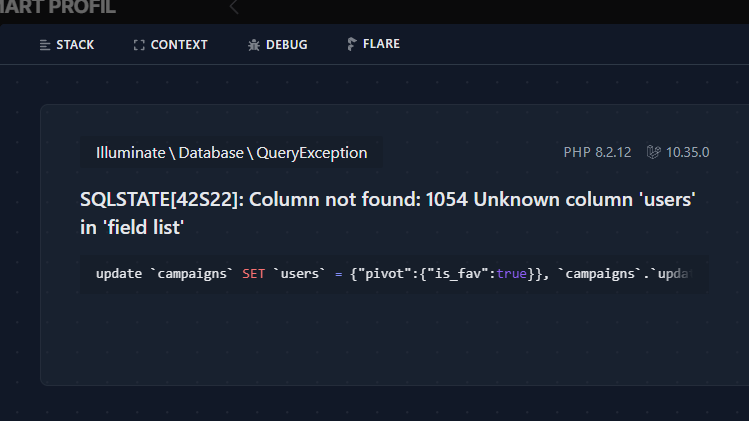
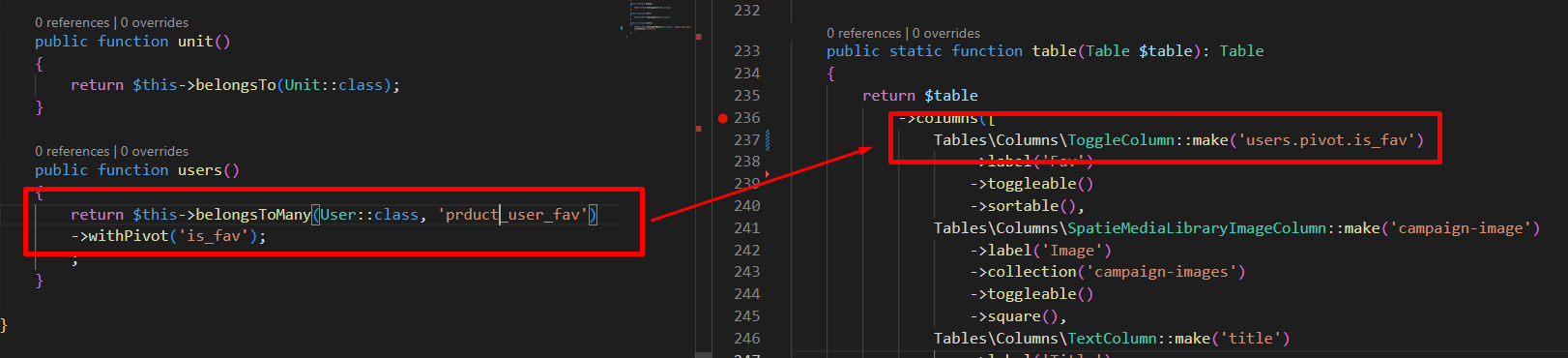
How to use is_fav field of pivot table for toggle column?
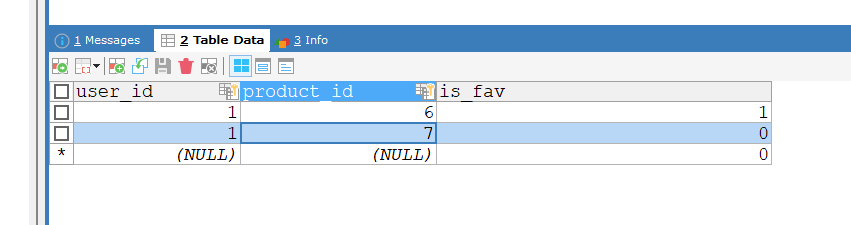
Ah, so you want the current user to be able to favorite it
Yes, I want to update the pivot table when toggling
Ok, I don't know if there's a better/official way of doing it (using pivot models https://laravel.com/docs/10.x/eloquent-relationships#defining-custom-intermediate-table-models)
So there are two problems:
- how to use the is_fav field for the toggle column.
- how to update pivot table when toggling.
Give this a try
also, make sure the syntax for the field is correct. I'm not sure how Filament handles pivot values
the
ToggleColumn::make('users.pivot.is_fav')
might not be enough. You might need to use a getStateUsing()
to get what you needHere is the filament pivot guide, but it is not working. I will report this packaging issue to the filament team and to all developers.
https://filamentphp.com/docs/3.x/panels/resources/relation-managers#listing-with-pivot-attributes
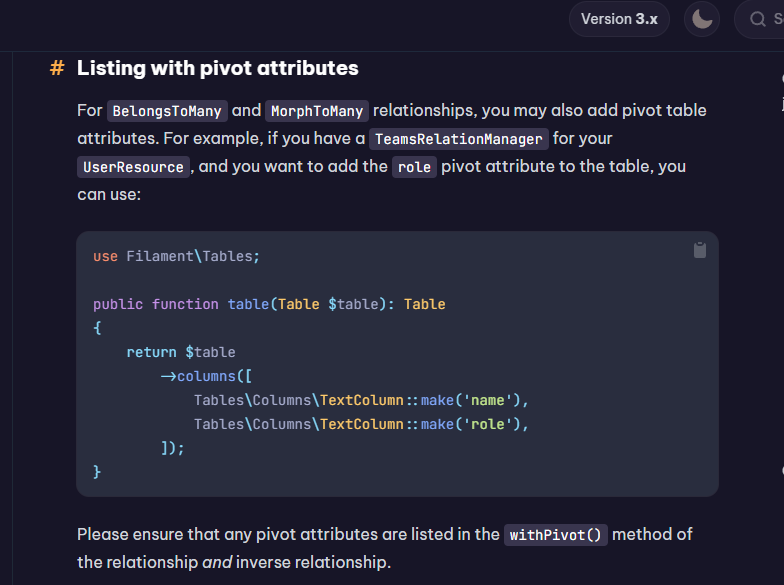
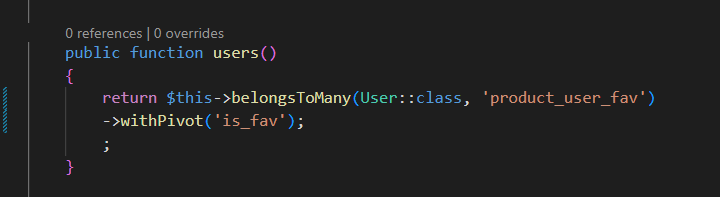
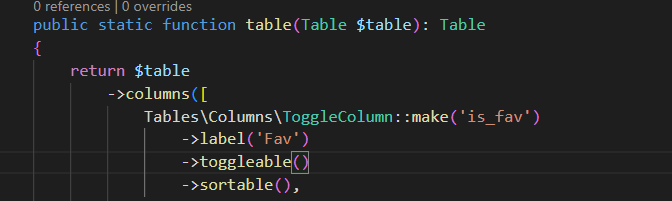
actually sorry my bad
the
toggle
will toggle the relationship. You need to update the pivot
valueYou are welcome 🙂
Can you introduce a developer to solve this problem?
or how to report this issue to Filament team?
there are no issues, it can be done
you just need to change the code
give me a sec
give this a try. The code is ugly but it should work
Ok got it, thanks.
The problem is that each product can have multiple users. You can simplify a lot of this by using some member functions or Attributes on the models.
Be careful to not end up with a lot of queries when using relationships (n+1 issue).
Awesome!
It worked!
You are a senior developer!!!
Haha thanks. Anyway, good luck 👍
👍
PS: do you know mimul.js?
Nope, sorry
Thanks for your support, plz let me know if you need any help, I will help you immediately!
Good luck!
is it possible to get the current column name ?
in my case I want multiple pivot in my table

"users.slot.0.pivot.is_approved_supplier"
I'm not sure how that would work in a relationship table, I've never used them. Either way, you should be able to modify the code above to work for most cases
The hardest part is figuring out how to get to the pivot you need to check/update
based on your structure
"Either way, you should be able to modify the code above to work for most cases" maybe if i can access the current column name
What do you mean "current column name" ?
"users.slot.0.pivot.is_approved_supplier" to get the index "0" in need
@jackleblackdev I think you can refer below code to get name:
him I try
Hi @ChesterS
Could you please help me a thing?
I'm using
SpatieMediaLibraryFileUpload
for file uploading, and it works, but it doesn't work when editing. I think it is related to CORS issue. Thank you.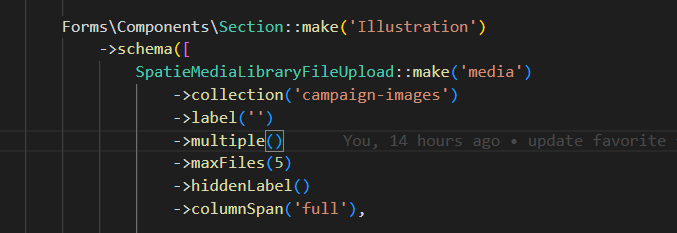
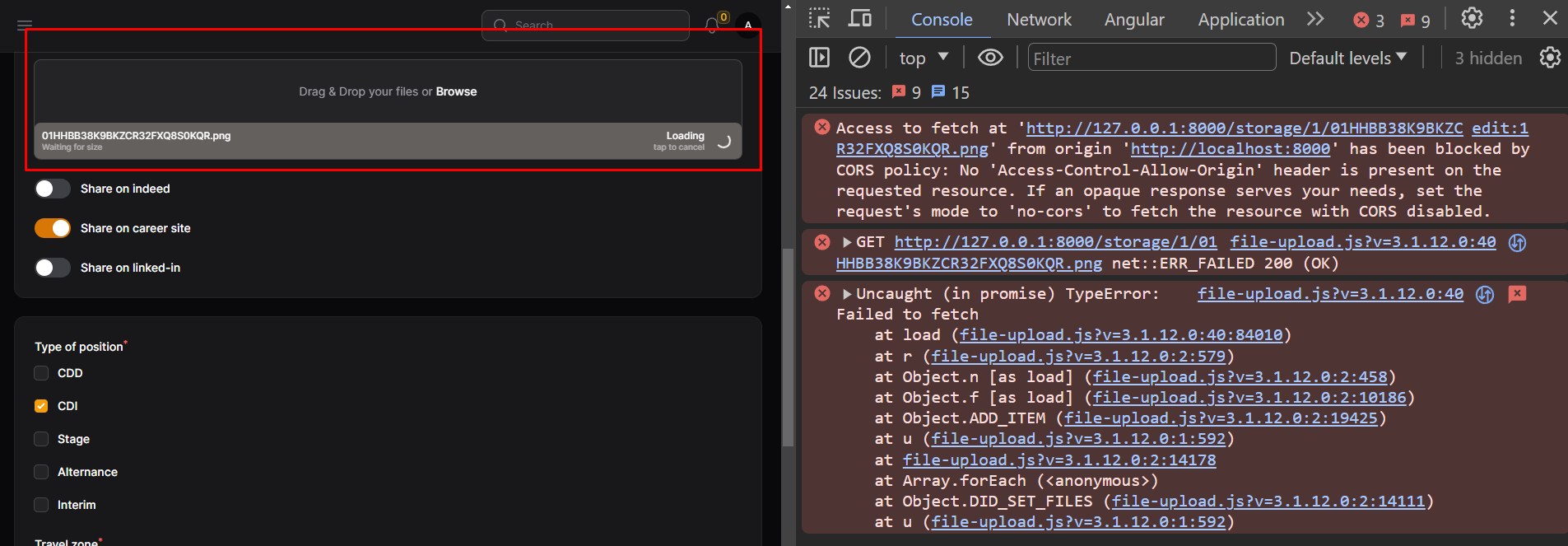
hmm maybe? it should work on localhost though 🤔 If you open that url, can you see the file?