12 Replies
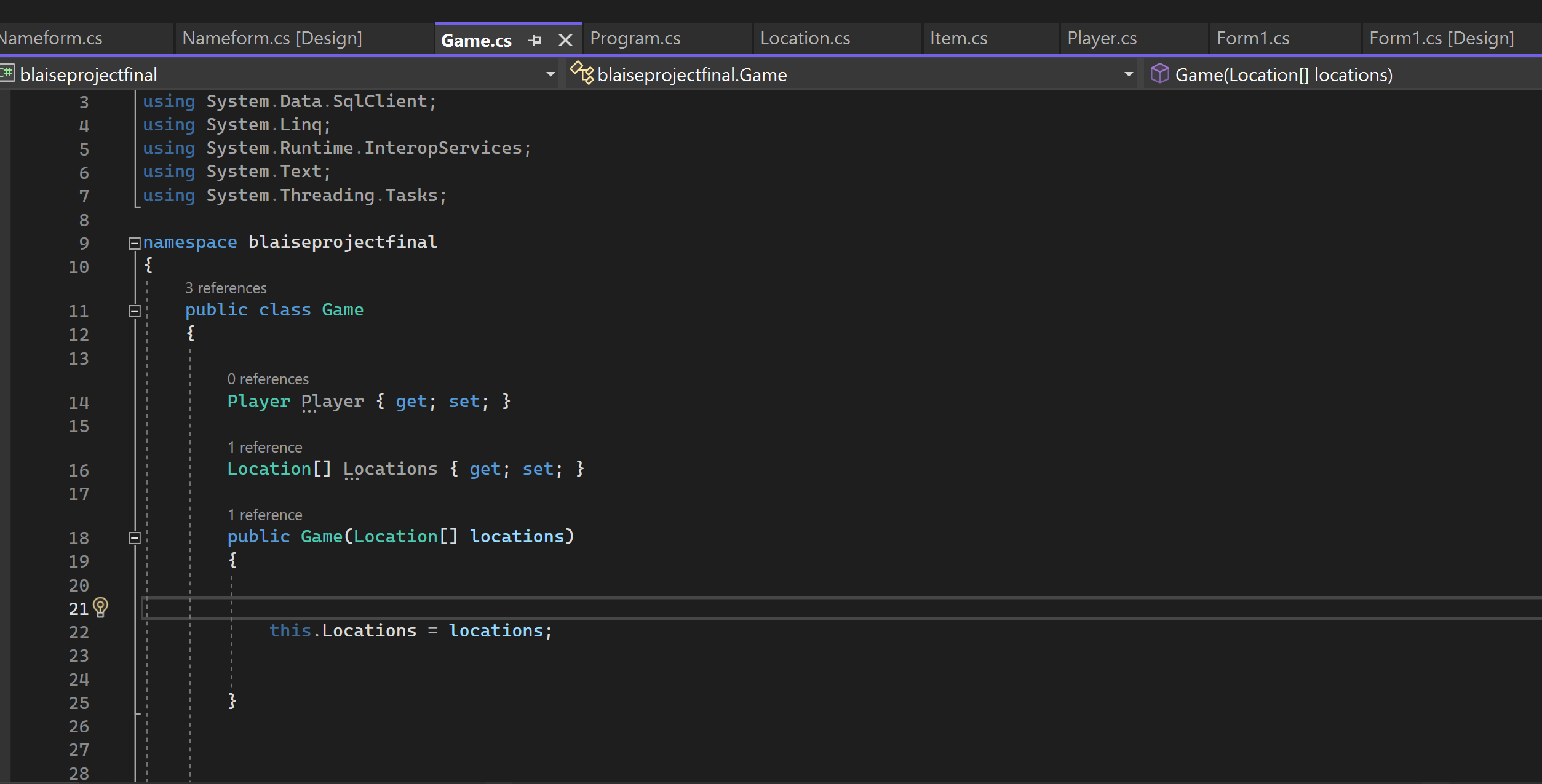
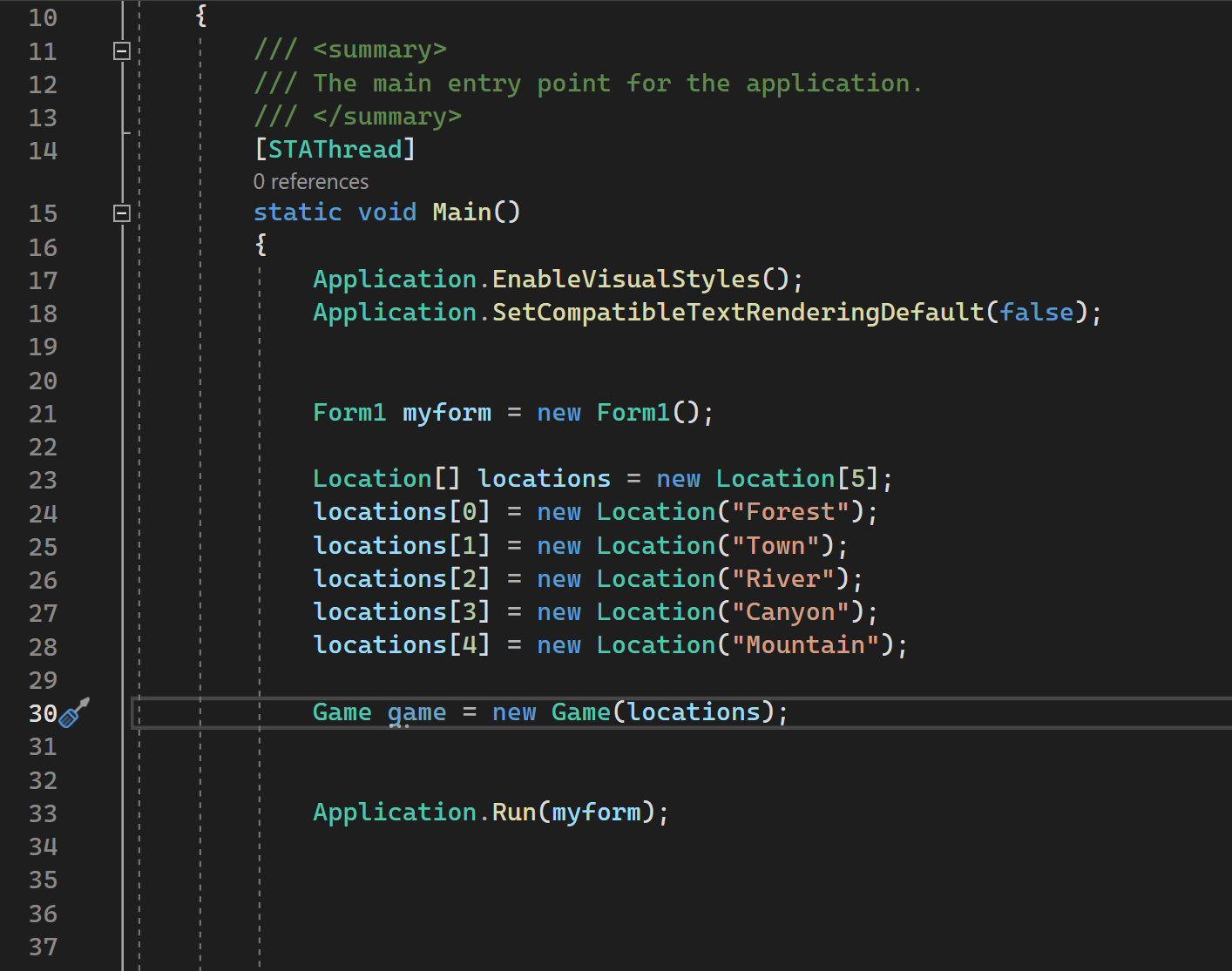
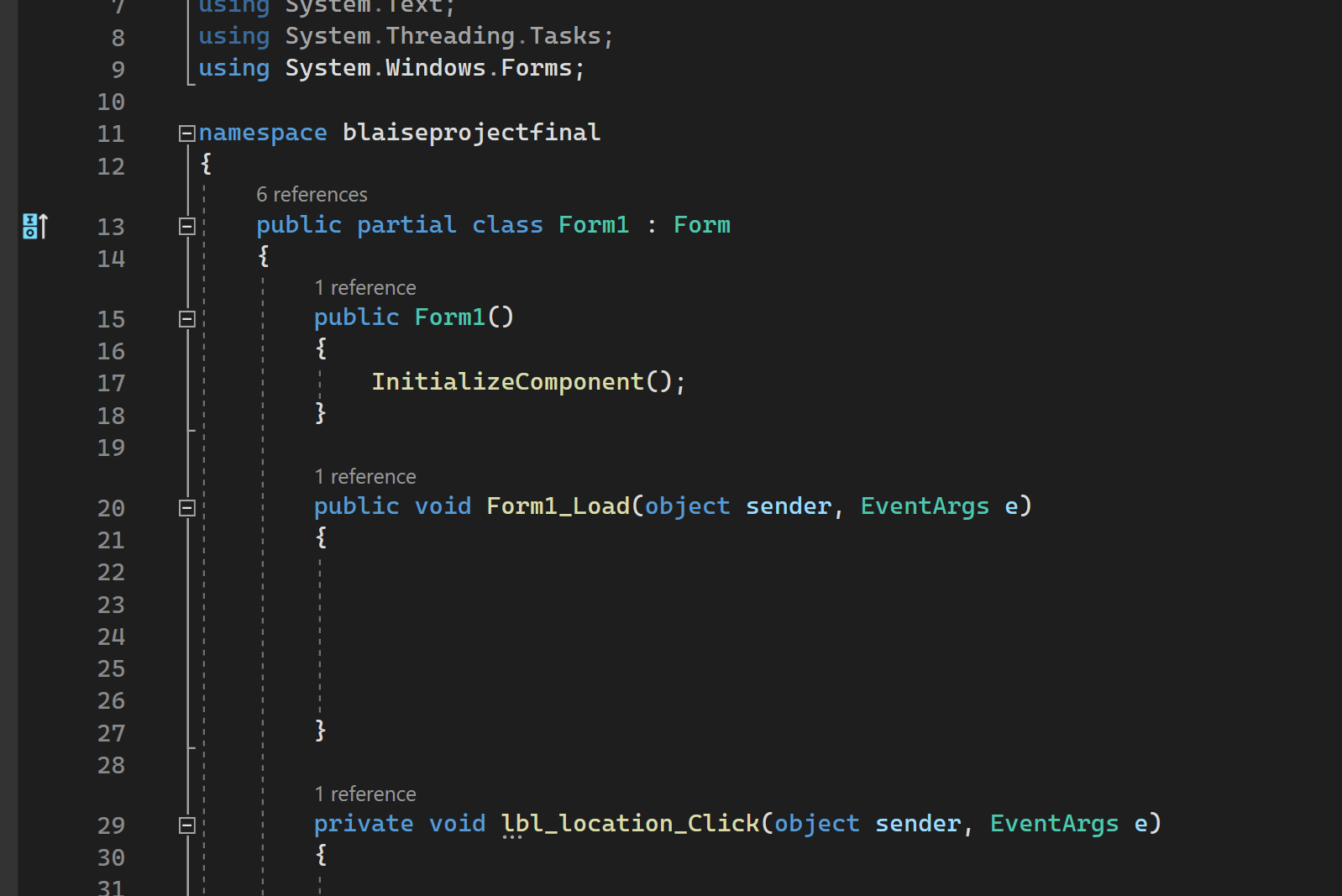
i want to be able to access the game obj from anywhere in my program
how would i be able to do this?
Best way? Dependency injection.
Easiest way? Create an instance of
Game
at the start, and keep it in a static variable
Another easy and slightly better way? Instantiate Game
at the start and pass it around.will try, thank you!
I think there's no out of the box tooling for di in winforms. I imagine it's fairly trivial to build up though.
Could prolly hook up Autofac or Ms.DependencyInjection fairly easily
i dunno what this means specifically but i could look it up : p
I got some quick and easy traction, but things definitely fall apart.
There's probably a "winforms way" to solve the disposed object issue on a singleton form
So I ended up with this nasty bit of code on the first try; it "works" in a naive sample
Here is my naive example : @chronos @ZZZZZZZZZZZZZZZZZZZZZZZZZ https://github.com/devdevdeveau/winforms-di-sample
thank you!
You're welcome to steal all the ideas there.
Which it's a public repo; should be a given