WPF and variables that I need access to in a Button_Click event
Trying to figure out how to create a random number for a guessing game. Obviously I need to know where to put it so it's accessible to the program and generates a number when the program loads and before I can use the button_Click event on a button. I've attached the code for the random number. I found Window_Loaded but that doesn't seem to be working. I also tried putting it in the Main Window area and without luck. Sorry, still new. Where would it go so it could be accessible to the different events I can setup?
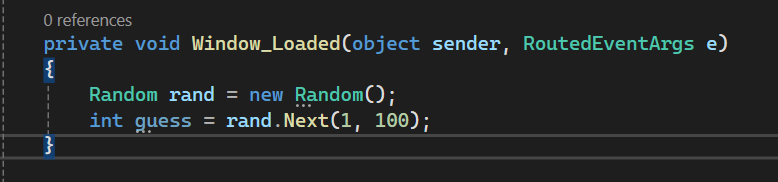
11 Replies
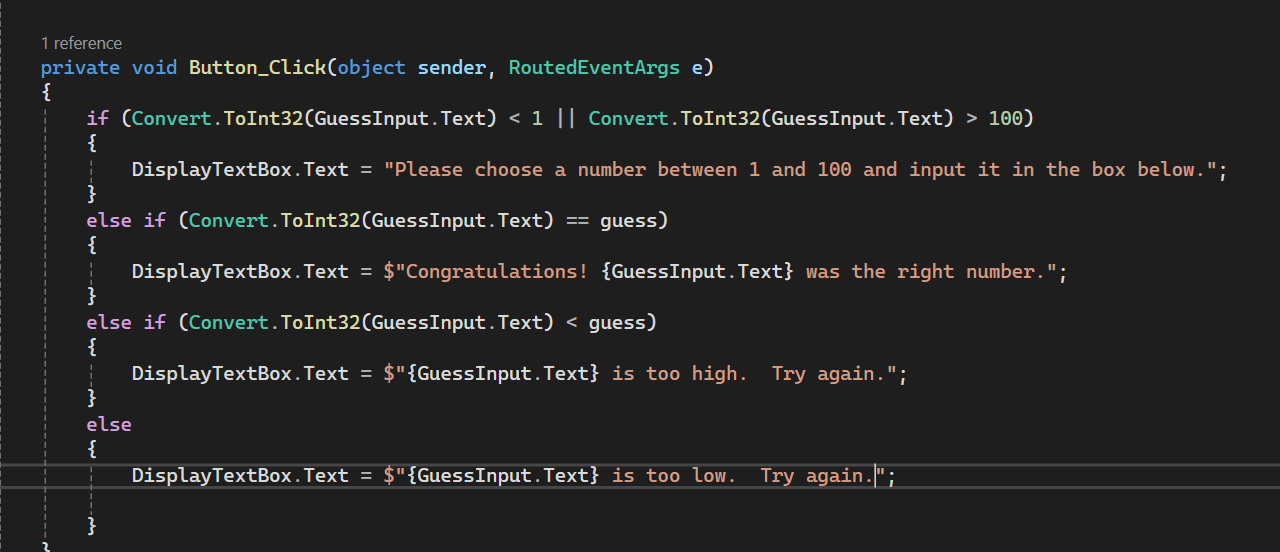
Also tried this
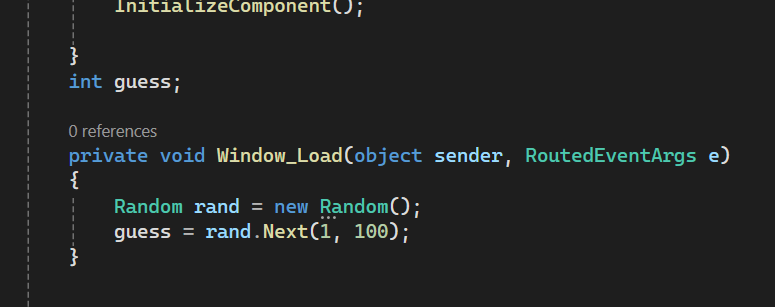
Okay first, this isn't the usual wpf way; this is winforms way; wpf the preferred way is mvvm. That being said, something to research later for you.
That being said
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/This is what I had...
so what I would end up with most likely is something like....
NB: This isn't the WPF way
but I said this earlier
and Convert.ToInt32 is really dated.
$tryparse
When you don't know if a string is actually a number when handling user input, use
int.TryParse
(or variants, e.g. double.TryParse
)
TryParse
returns a bool
, where true
indicates successful parsing.
Remarks:
- Avoid int.Parse
if you do not know if the value parsed is definitely a number.
- Avoid Convert.ToInt32
entirely, this is an older method and Parse
should be preferred where you know the string can be parsed.
Read more hereOk thank you. I will continue to work on it. I did end up using Int32.Prase and followed someone'se tutorial to try it out but I guess it's still nott he WPF way. I'll have to learn more about it. As of right now I have this working:
@drewhosick when I say it's not the WPF way, most folks using WPF use an idea calld MVVM
$mvvm
guess no tag
so mvvm is a different programming paradigm, still c# of course.
Yeah, I started reading about it but I am not very familiar with it yet. I will read more and continue to learn. Thanks for your time
It's not easy to start with, but once it makes sense it's good to know