Custom Column Filament
#Model: OrderItem.php
#Model: Order.php
#File: OrderResource.php
How to display total price from
order_item
table? so weight
* unit_price
12 Replies
The above presuming you are storing cents?
You can also adjust the eloquent query to return a sum at the MySQL level
I got an error message:
orders table
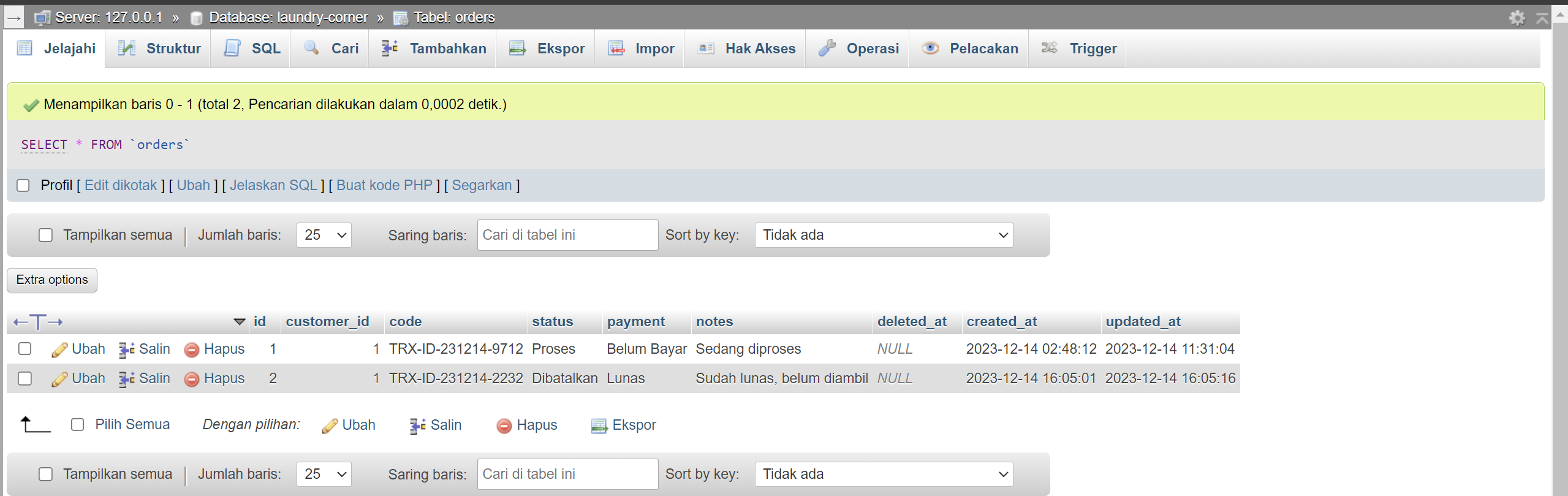
order_items table
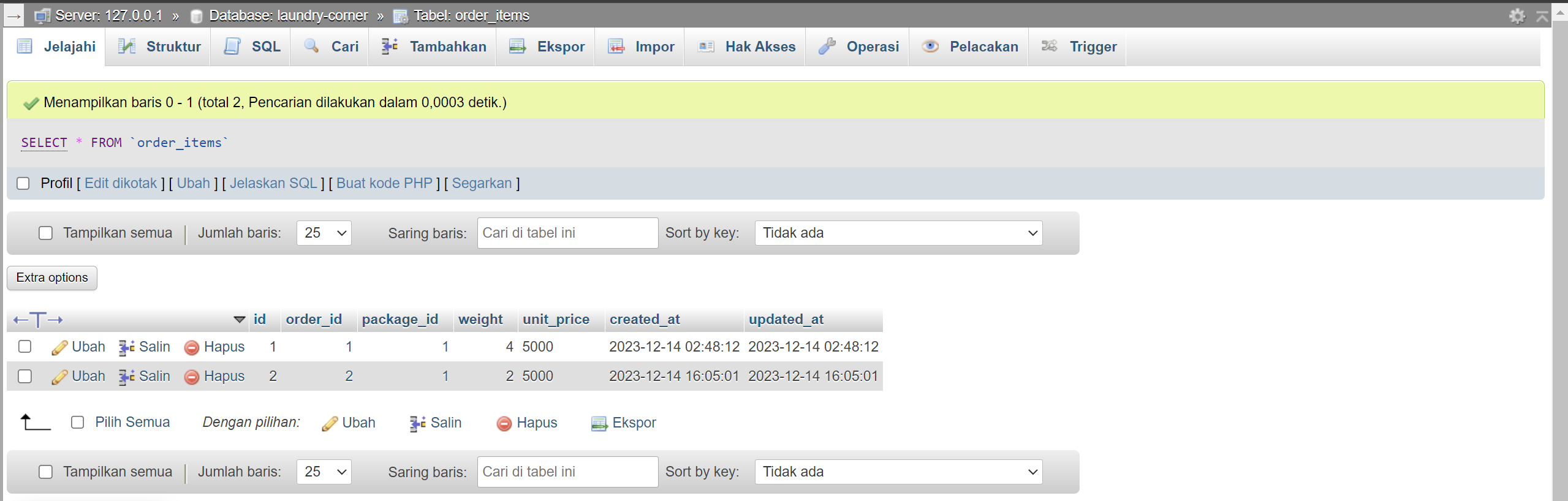
It's because you are in the order resource. So you'll have to change OrderItem to Order and then loop through all order items to calculate the sum
Is there no other way? so that the OrderResource table can retrieve an OrderItem?
Above is kinda right, you want to go through the items
so instead of $record, as you have a relationship of items you want to look through them items. Why not just have a sum relationship on the model in that case and just return that instance which handles it for it
But you have a hasmany relationship. So you cannot fetch just 1 item for the order
I'm just learning to use filament, so I don't really understand it
It's not really filament related, more Laravel.
You can also try the sum method on your column https://filamentphp.com/docs/3.x/tables/columns/relationships#aggregating-relationships
It's been resolved, thanks to the help you gave. This is the code I fixed
Greate