I really need to complete the simulated annealing method for the traveling salesman problem
Deadlines are running out, there is a program that should work, but it has errors that I cannot fix due to poor knowledge of the code, please help. I don't even know what part of the code is wrong
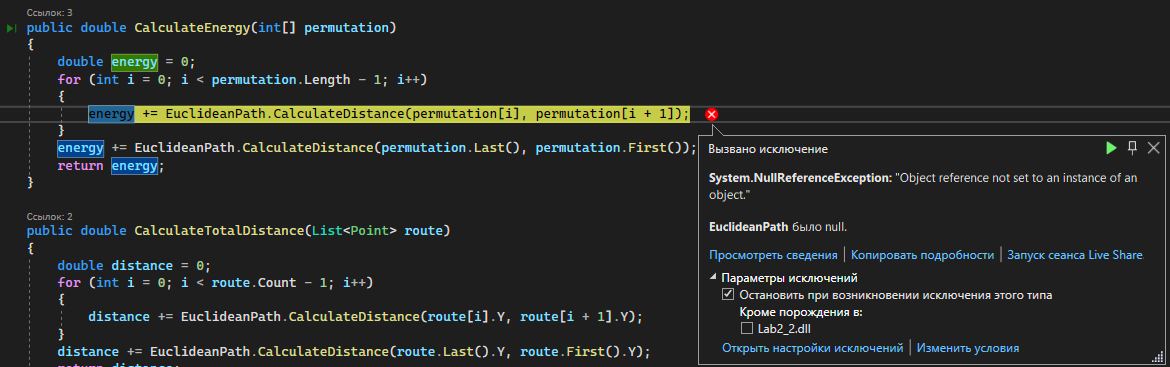
10 Replies
When I enter all the values I only get 1 error(screenshot)
I understand there is an error somewhere here
Do you create a new instance of the
EuclideanPath
class anywhere? The error is weird though because it seems like you're calling a non-static method on a class name. That shouldn't even compile.Now I have created an EuclideanPath class object in the CalculateEnergy function. The class object looks like this: EuclideanPath euclideanPath = new EuclideanPath(cities);
But it says that the name Ńities does not exist in the current context
Well,
EuclideanPath
constructor takes cities as a parameter, it has to come from somewhere. I presume that cities should be an input to the program?
So you probably have to construct the liset of the cities by reading some data from somewhere.Cities are created through this function
ok, then:
should work
I donāt quite understand where to write this part of the code, does it replace my GenerateCities method?
probably in the "CalculateEnergy" method
also, I updated the code above
it was wrong
and then replace EuclideanPath with euclideanPath. Class with an instance
The problem is that the number of cities is taken from the textbox
Do you have a debugger available?