Registration Page with custom Field
I added new Register Page to my Panel, and need a new Field for REFERENCE CODE INVITE.
But causing this error when send submit.
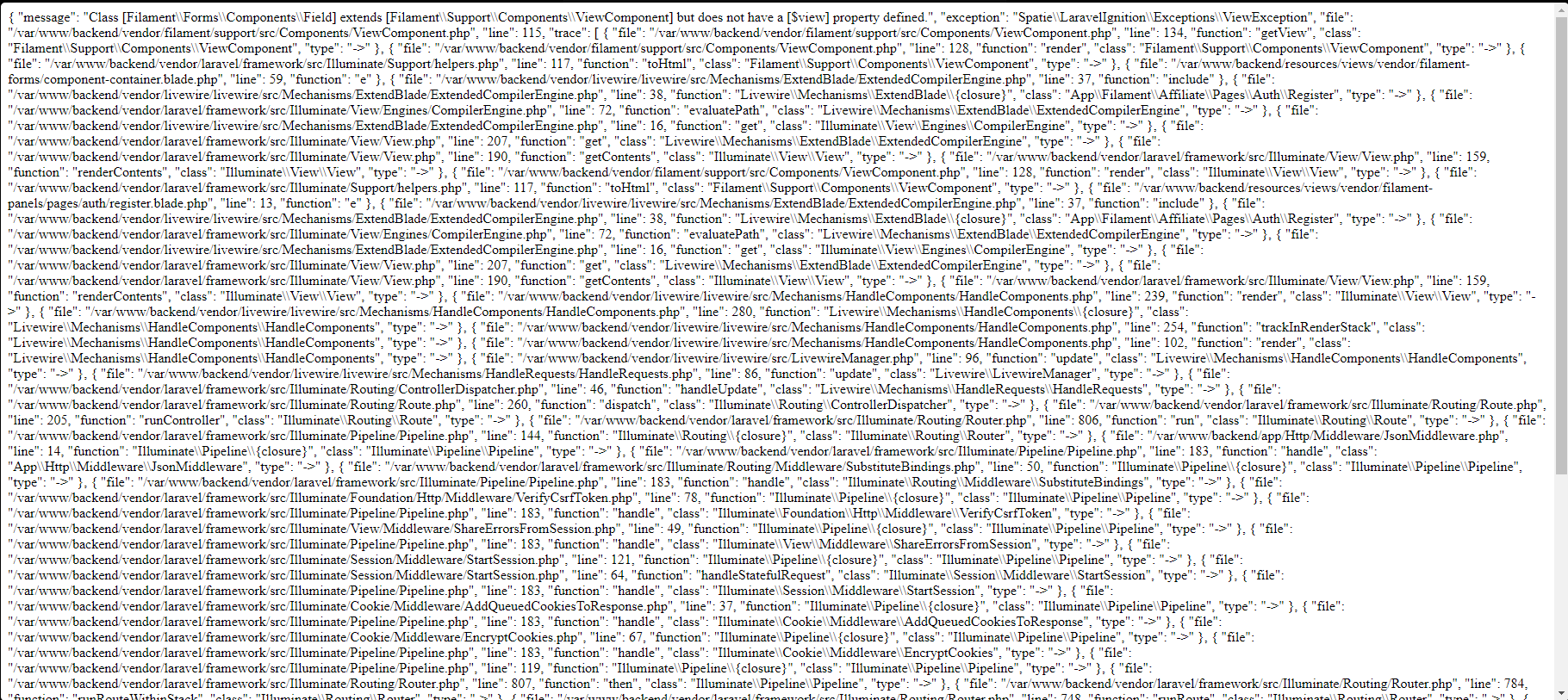
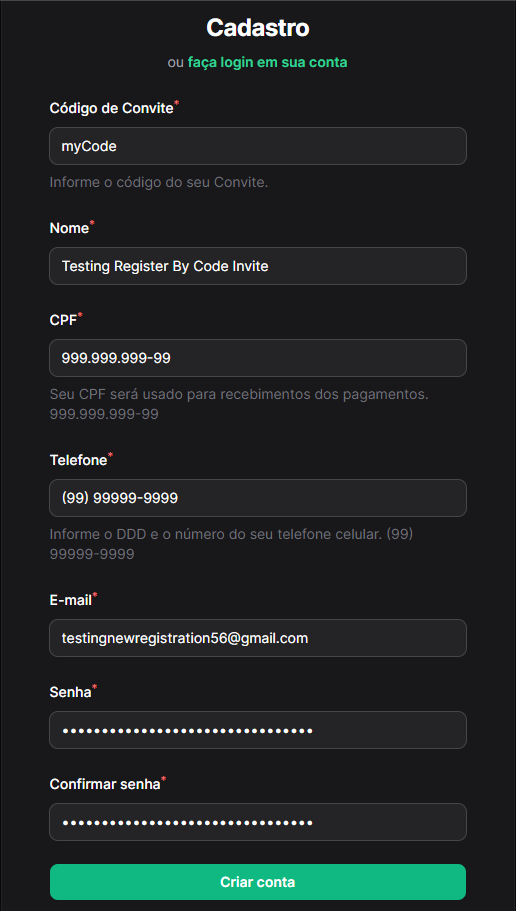
7 Replies
Can you post the FlareApp share URL from the error page? (Your screenshot of a bunch of error text is really hard to read; seeing the stack trace in Flare would be much easier to trace through)

And it would be helpful to see the code for your Register page and User model.
How to capture the share link if error is from Filament Error in Modal Popup?
Does the same problem happen if you "only clone the Registration" class? ie: if you back out all the things you've added, does the problem continue? Researching that will help find out which field or code-change is triggering it.
I didn't quite understand what said.
My problem is that I want to enter another user's guest code and then associate the code owner's ID with this new record. understand?
The registration system works, if I remove the "invite code" input required.
however, I need this field for my project concept to work. and this is causing me problems because I can't find any way to make it work.
You said the errors appear when you click Submit ... which is when Validation runs.
If you remove the
->exists(....)
logic from that InviteCode field, does it all work correctly? (obviously, except that it could allow a a fake code to be used). So: is the error in your custom validation rule?