Error while using useNavigate() or <Navigate />
PrivateRoute.tsx
import { Route, useNavigate } from '@solidjs/router'
import Index from '../pages'
const PrivateRoute = () => {
const isLoggedIn = !!localStorage.getItem('token')
const navigate = useNavigate()
if (!isLoggedIn) {
navigate('/', { replace: true })
}
return <Route path='/' component={Index} />
}
export default PrivateRoute
App.tsx
import { MetaProvider } from '@solidjs/meta'
import { Route, Router } from '@solidjs/router'
import { Component, lazy } from 'solid-js'
import PrivateRoute from './routes'
const Login = lazy(() => import('./pages/Login'))
const App: Component = () => {
return (
<MetaProvider>
<Router>
<Route children={<PrivateRoute />} />
<Route path='/login' component={Login} />
</Router>
</MetaProvider>
)
}
export default App
The error in browser is Uncaught Error: Make sure your app is wrapped in a <Router />
Any Solution21 Replies
"@solidjs/meta": "^0.29.3",
"@solidjs/router": "^0.10.5",
"solid-js": "^1.8.8",
The children syntax here is strange.
Also the route with children prop has no path.
change to this
const App: Component = () => {
return (
<AuthProvider>
<MetaProvider>
<Router>
<Route path='/'>
<PrivateRoute />
</Route>
</Router>
</MetaProvider>
</AuthProvider>
)
}
but still same problemWhat settings do you use? Bare solid-start?
It's not a solid start it's solid with vite
By this cli npm create vite@latest my-app -- --template solid-ts
1. update your solid to the latest version (just tried it out w the template and was on 1.7.6 and then it's missing a function the new router needs)
2. use the component-prop to inject it into the router, do not use children
p.ex
But now warning popup in browser i.e Unrecognized value. Skipped inserting {children: undefined, path: '/', component: ƒ}
Any reason why inside PrivateRoute you have another Route-component?
the problem is solved from above code but what will be props type ? const PrivateRoute = props =>
GitHub
GitHub - solidjs/solid-router: A universal router for Solid inspire...
A universal router for Solid inspired by Ember and React Router - GitHub - solidjs/solid-router: A universal router for Solid inspired by Ember and React Router
so in the new router
props.children
in a route-component is a slot.
that also explains why
does not produce anything, because there is no component in the component-prop that uses the props.children.
on the other hand does use the props.children-slot.
Besides {children: JSX.Element}
afaik there are no props on a route-component.now see the difference with your code and my code
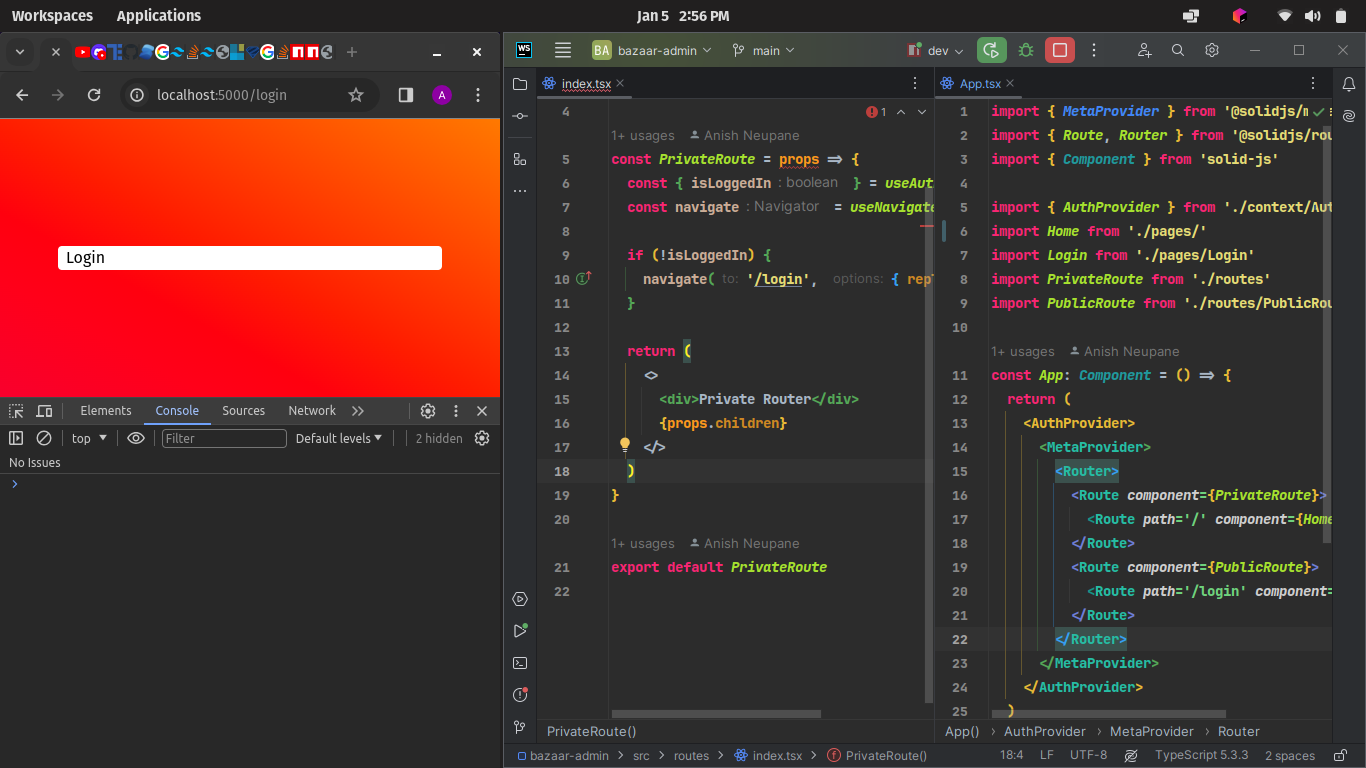
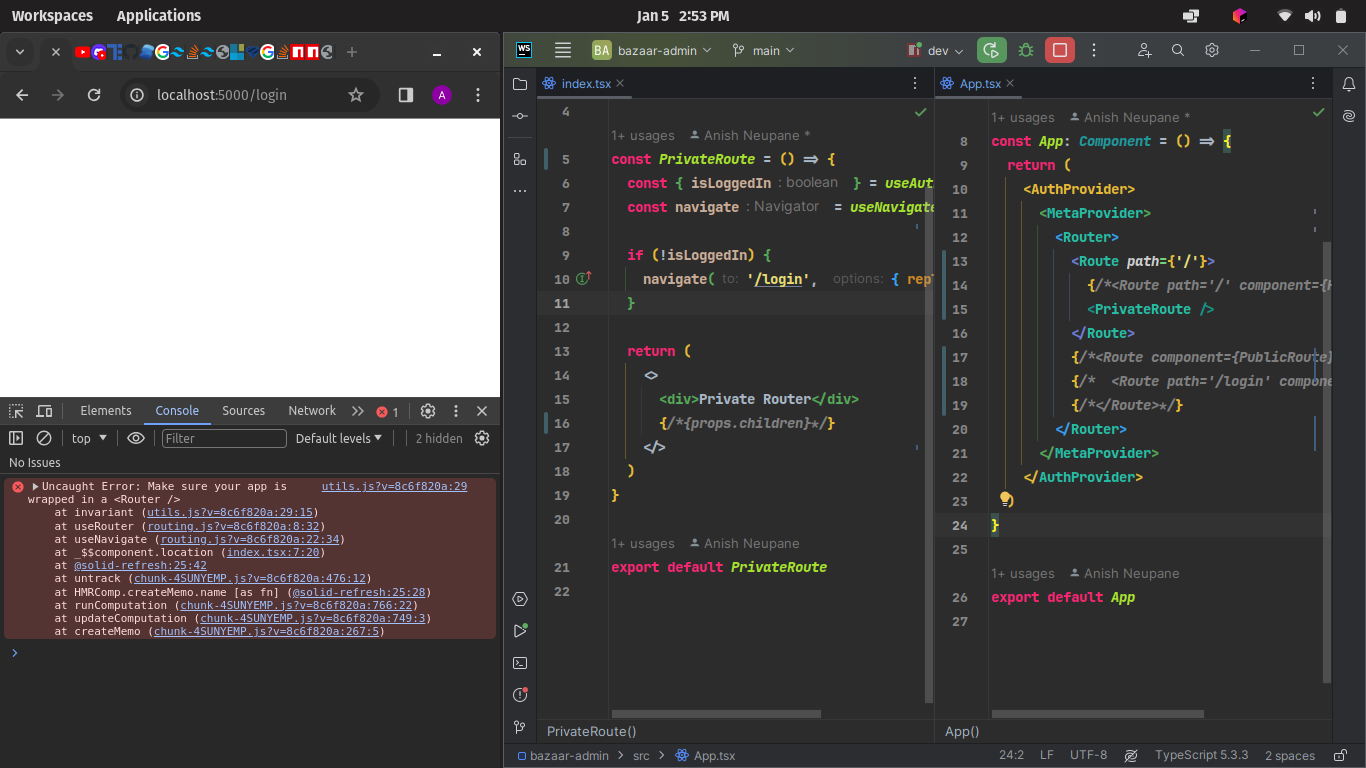
please check this also
https://codesandbox.io/p/devbox/laughing-mcnulty-zz5ct3?file=%2Fsrc%2FApp.tsx%3A23%2C20
what is the question?
you said
but while using this syntax error occur and the error is provided through image above with your syntax and other is my syntax and also provide the codesandbox with my example
Besides {children: JSX.Element} afaik there are no props on a route-component.that was incorrect assumption btw. there is
RouteSectionProps
a sorry for the confusion, I tried to say that that's the incorrect way of doing it, since Route needs a component that uses the props.children.So please review my code is this correct or not
https://codesandbox.io/p/devbox/laughing-mcnulty-zz5ct3?file=%2Fsrc%2FApp.tsx%3A23%2C20
does it do what u want it to do?
Thank you for your support
ur welcome!
i m still figuring out the new router too 🙂