✅ Complicated error with C# SignalR client
Hi, I'm trying to receive base class in signalr using
connection.on
function. My base class has inherits and they all may be sent to the client.
Problem is in connection.on<T>
method.
It converts whatever JSON serialized data comes to it straight to the T
type, ignoring T
's dervied types.
I have tested it by wrapping my Foo
class in wrapper class Bar
and passing it to the method:
This way, if I store Baz: Foo
type in Bar
and pass it to client, it will recieve Bar
with foo
field having Baz
type.
Is there a way to make connection.on<T>
convert incoming data not just to T
, but to it's derivative too without using wrapper classes?15 Replies
How to serialize properties of derived classes with System.Text.Jso...
Learn how to serialize polymorphic objects while serializing to and deserializing from JSON in .NET.
What you need is a type discriminator
Alternatively, you could write a custom json converter, but that can get ugly very fast, if the only thing you need it for is type discrimination
I have
JsonDerivedType
attribute on my base class, but connection.on
still refuses to convert data to the derived classes, it just stops converting at the type you passed in genericAnd when serializing, each type inheriting your base has a $type json element?
I've recently faced the same issue and this helped me solve it
Just checked it and looks like problem is in serializer indeed. It doesn't provide $type element if I'm serailizing base class, but if I serialize wrapper class that contains base class it does provide $type element
Consider the following hierarchy:
And the following collection:
Serializing the
pets
as is will produce this JSON:
Decorating the IPet
interface with:
....and serializing again shall produce the following result:
Are you using System.Text.Json
or something else?
I forgot to provide the code for serializing
Yes, I'm using
System.Text.Json
. There is no problem with manual serialization, but when I pass a value to signalr's SendAsync
function it doesn't serialize it's value with $type
property
Here's simple example to reproduce this bug:
Models:
Hub:
When I'm sending foo
, json serializator doesn't include $type
property to json.
When I'm sending wrapper
, json serializator includes $type
property to json.I've checked it via WIreshark
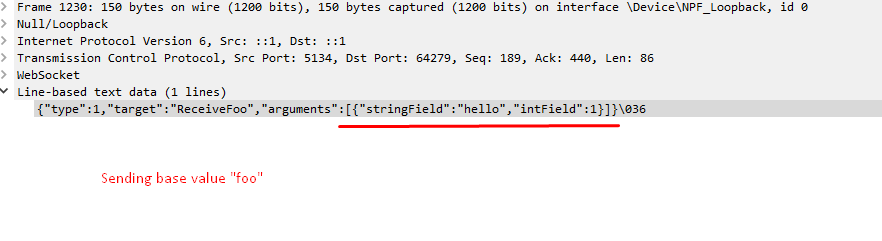
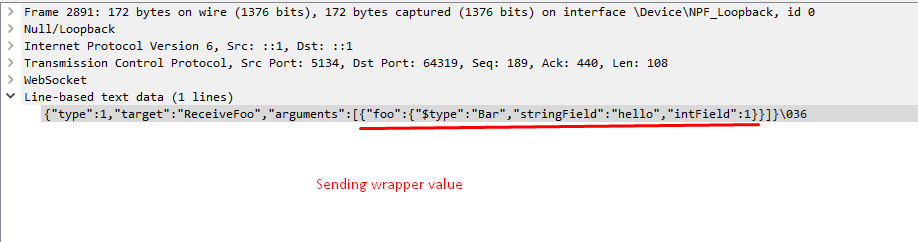
if I serialize and deserialize manually, then derived types are resolved properly
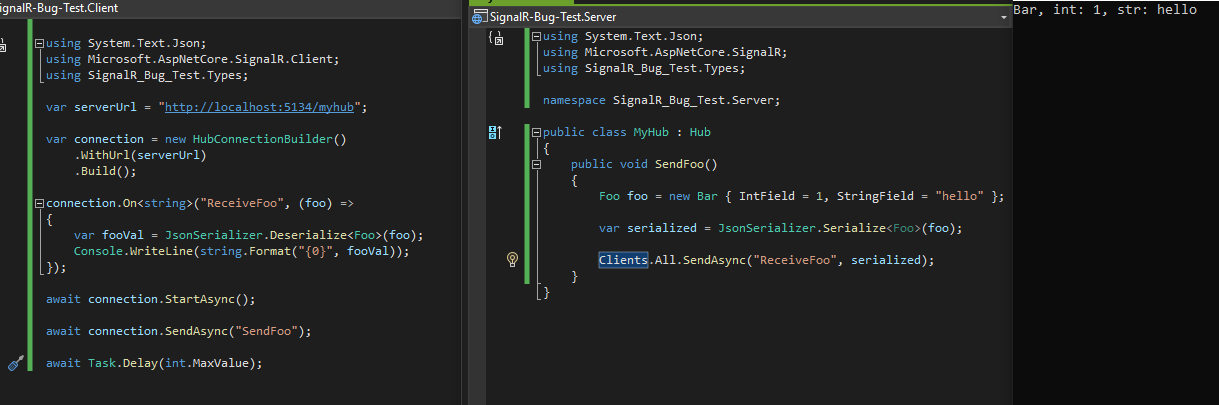
seems like a bug in signalr 💀 https://github.com/dotnet/aspnetcore/issues/52342
GitHub
The JSON returned by SignalR does not contain the $type field · Iss...
Is there an existing issue for this? I have searched the existing issues Describe the bug When serializing descendant types, for methods that take a single (not a collection) parameter of the base ...
Oh, thank you for sending a link, I was thinking about creating an issue myself 😅
this kind of an issue is very unfortunate... Is it possible for you to ser/deser your self and send JSON directly via SignalR?
Yes, I can do that or use wrapper type for now.
Thank you for help, I think this problem is now resolved
$close
Use the /close command to mark a forum thread as answered