Custom Field does not submit data
Created a custom field as in the docs, using
artisan make:form-field ProductPrices
. The component looks like this and receives an array of Currency
models:
And I use it in my form as:
The view of my custom component is:
And an input field is indeed generated for every currency: <div><input wire:model="data.pricesTest.1"></div>
In EditProduct extends EditRecord
, where I use the ProductResource
with said form I do a dd()
:
Other values of 'default' Filament form fields are there but I cannot figure out why my custom field PricesTest
isn't submitted into the form data. Can someone please point me where I'm going wrong?Solution:Jump to solution
Thanks again for your help @Lara Zeus . It seems that the
setup()
function in the ProductPrices
does the trick. I think it needs to be setup as an array. And it works when setting it up in the same way that CheckboxList()
does. Final working code of the custom field:
```php
class ProductPrices extends Field {
...6 Replies
this can be obvious questions but 🙂
- the
pricesTest
is exist in db
- pricesTest
is fillable in your model
- pricesTest
is cast to array
also after saving, check the database directly to sees if it stored or notThank you for taking the time to reply.
pricesTest
isn't a real property on my Product
model but for the sake of trying to work it out this doesn't make any difference when added:
I would expect the dd()
data in mutateFormDataBeforeSave()
to show the data from the form and not necessarily the model attributes. But it still comes up as null
in mutateFormDataBeforeSave()
.
(also tried adding a column pricesTest
to the db)ok
do you get a console error?
ok
I tested you code, and I remember my class extending
CheckboxList
not
Field
not sure which trait you need (didnt deg deepr), but if it dosnt make any diff to you
you can also extend CheckboxList
and all will work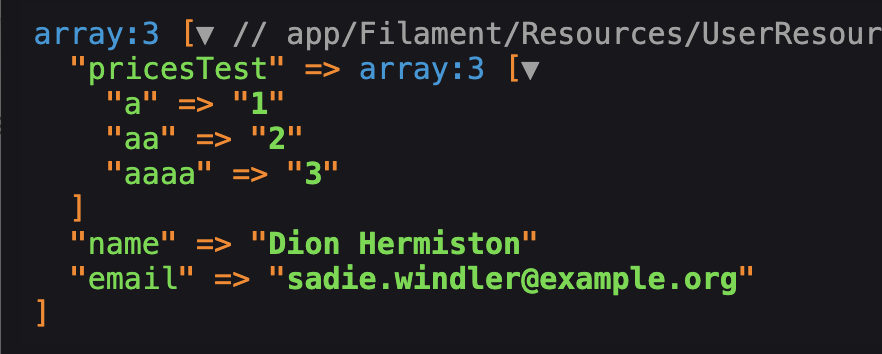
Thanks, I will look into this and report back if I get it working. If anybody knows which trait is needed then please let me know!
Solution
Thanks again for your help @Lara Zeus . It seems that the
setup()
function in the ProductPrices
does the trick. I think it needs to be setup as an array. And it works when setting it up in the same way that CheckboxList()
does. Final working code of the custom field: