My first solid component
Just wrote my first component. I only wrote React before so I wanted to know if there are better ways to do things than what I figured out so far :)
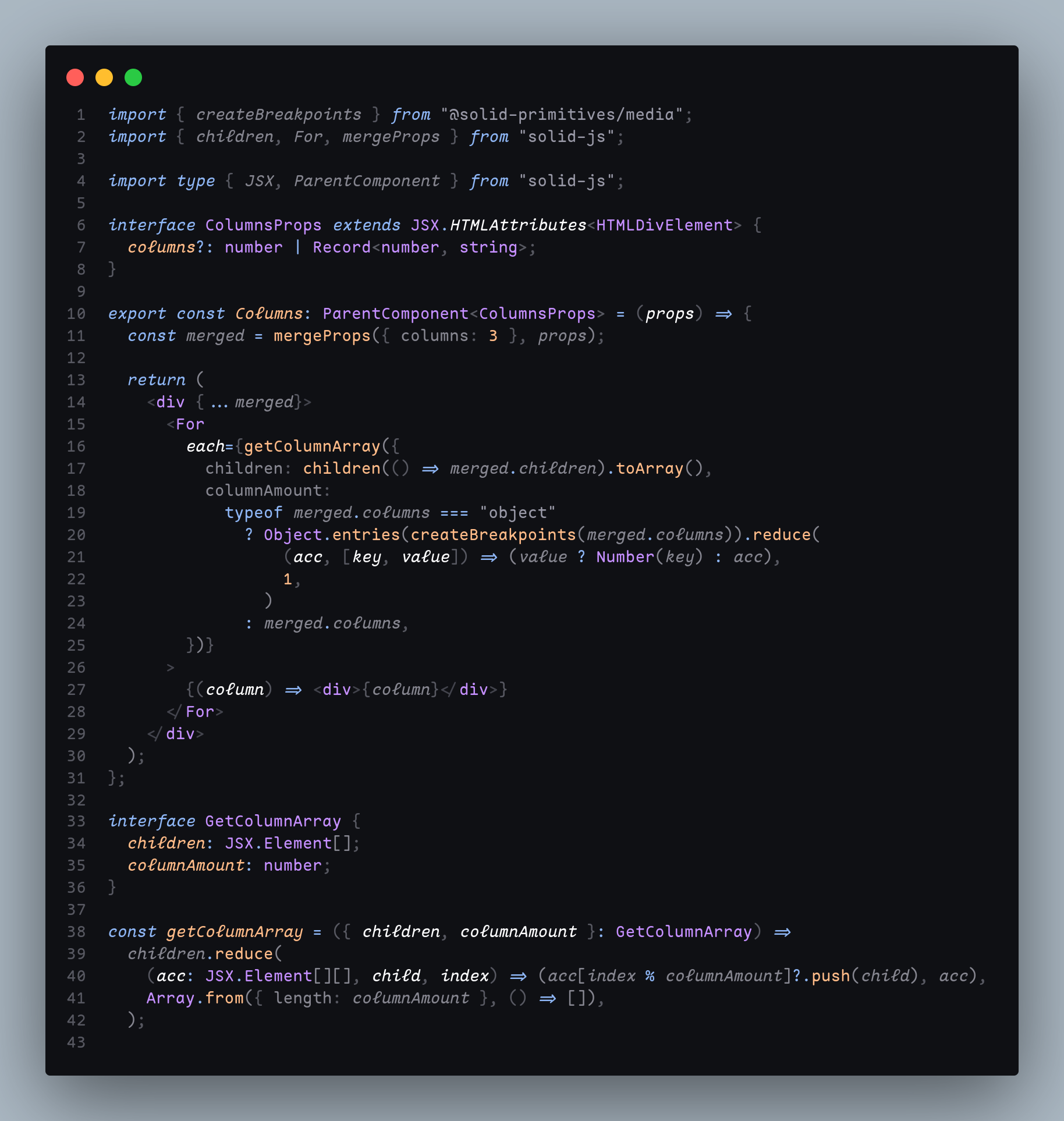
22 Replies
Code and image are the same, I attached it because I find Discord's codeblocks hard to read
1. What is the use of the
.toArray()
with the children?
2. children(...)
returns a signal containing the resolved children. To get the actual children you would need to call it.
3. Something to look out for: props.children
can either be a single element or an array of elements.1. that's the solution I found for iterating over children
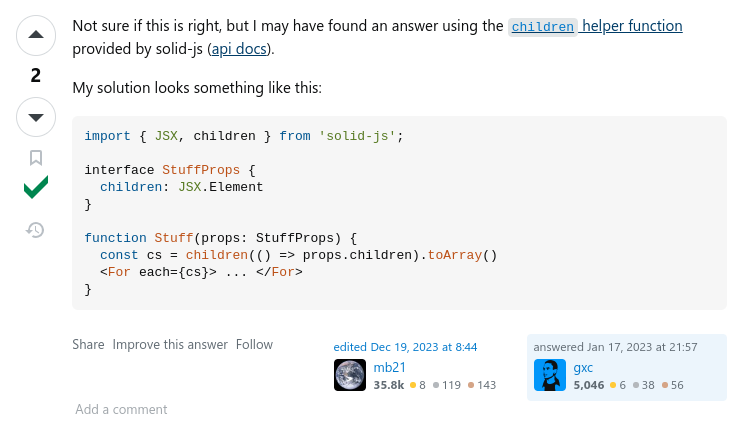

merged.children is of type JSX.Element | undefined so I can't iterate over it
props.children is of the same type so I think 2. is wrong
interesting!
that stackoverflow example will not be reactive
but in your case it will work fine, since you are calling
children()
inside the JSXdid not know about
toArray
!
but this would be reactive:
cool!
calling
children
in jsx will be reactive
but it will also wastefully recreate memos each time it reruns
so better to go with what @bigmistqke wrote
also it currently has an issue that if collumns
prop changes, props.children
will be executed again, recreating elementsI think it's reactive already?
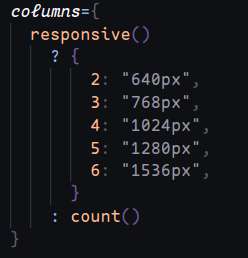
At least this works
Unless I'm misunderstanding
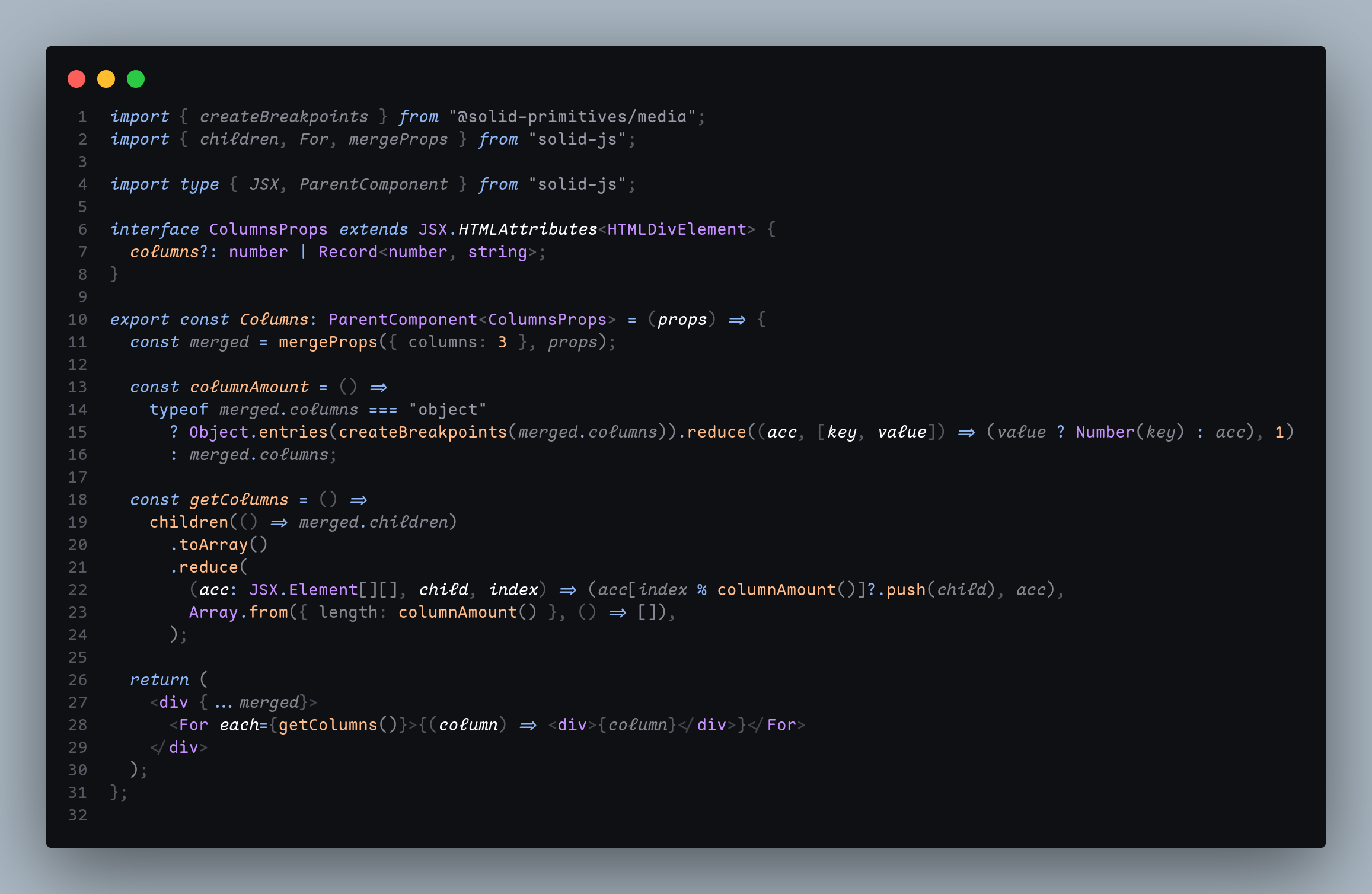
This seems to be working fine too even though I'm getting a warning @ line 22
This function should be passed to a tracked scope (like createEffect) or an event handler because it contains reactivity, or else changes will be ignored.
I can't tell whether this is a false positive or something is actually wrong and I don't understand it
@joshwilsonvu can you help?but it will also wastefully recreate memos each time it rerunsyou are still creating a memo each time. better to bring
children()
outside of getColumns
and into ColumnsProps
body like
This function should be passed to a tracked scope (like createEffect) or an event handler because it contains reactivity, or else changes will be ignored.
this might be solved by renaming getColumns
to createColumns
see https://github.com/solidjs-community/eslint-plugin-solid/issues/112#issuecomment-1844195070GitHub
Enable customizing reactive rule to treat certain custom functions ...
Describe the need I am making a library for my team which handles data fetching with our custom protocol. The library will take in the arguments to the API call and return a signal, very similar to...
generally if something starts with
create
or use
or returns an accessor instead of the value, you probably want to use it at high as possible (eg in component body, parent component or global store), instead of inlining it in jsx, memos, or functions like getColumns
.
also even if stuff is updating correctly on the screen
you might want to add random console logs to various places to see if something isn't updating more then it should
in solid functions should rerun only when needed, no more no less
eg elements are good to check if they are not recreated for some reason, because that might be expensive and cause weird behaviors later, even though it seems ok because "it's reactive"
<div ref={console.log} />
<- will log every time an element is createdChilds isn't getting called here so you can't reduce it
That didn't work
I don't think I understand how memos work
How do I check if one is being created
oops typo, u right. should be
it's mentioned in the docs https://www.solidjs.com/docs/latest/api#children
The return value is a memo evaluating to the resolved children, which updates whenever the children change.with a memo we mean
createMemo
: https://www.solidjs.com/docs/latest/api#creatememo . you can think of it as a combination of a createEffect
and createSignal
: a signal that only updates whenever one of its dependencies change.Oh that makes sense
As for this I think I'll just disable eslint for that line
ye the linter is quite aggressive.. i never use it in my own code tbh
can u post the code? wanna check it in the playground
thanks 🙏
i feel that the linter should be able to recognise these situations.
gives the same linter-error