Capturing multiple displays scaling information
I have managed to code a function that semi works in terms of capturing all displays scaling. The only issue is, it captures the default monitors scaling only. The function I found and changed slightly is below:
With this, it returns 1.25 for 125% scaling. If I change my default monitor to 150%, it will return 1.5. So it does return the scaling.
The only issue I have now is passing the monitor index through as a parameter. And then using it in the following way
This will throw an error once monitor index is not 0. The error shows the following:
According to my understanding of the program. The value of 'monitorHwnd ' will be something like when it is 0 and when it is 1 and so on.
This is how I call my function:
The overall reason for me needing the scaling is for a feature I am making that will take a screenshot. This is one of those functions to make the app work on multiple displays.
16 Replies
ok ill take a look
Thank you!!!
I've been working on it for the past 3 days. This is the closest I have gotten.
When we pass 0 into the pointer it does give 125% for all screens which as you said may be due to the null hwnd
i think you could use https://learn.microsoft.com/en-us/windows/win32/api/winuser/nf-winuser-enumdisplaymonitors
and
https://learn.microsoft.com/en-us/windows/win32/api/shellscalingapi/nf-shellscalingapi-getdpiformonitor
EnumDisplayMonitors function (winuser.h) - Win32 apps
The EnumDisplayMonitors function enumerates display monitors (including invisible pseudo-monitors associated with the mirroring drivers) that intersect a region formed by the intersection of a specified clipping rectangle and the visible region of a device context. EnumDisplayMonitors calls an application-defined MonitorEnumProc callback functio...
GetDpiForMonitor function (shellscalingapi.h) - Win32 apps
Queries the dots per inch (dpi) of a display.
I was able to fetch the screens
Monitor \.\DISPLAY1: HWND = 65539
Monitor \.\DISPLAY2: HWND = 65541
Monitor \.\DISPLAY3: HWND = 65537
EnumDisplayMonitors(null, null, your delegate, 0)
in your delegate GetDpiForMonitor for each handle it gets
Ok, will give this a try in about 5 min
Wanna get coffee real quick
Can I ask, do you mind trying to assist me with the existing fuction.
I changed it to look like this now. It now uses the hWnd. So it handles that window
you should dispose the graphics and hdc at the end
Ok
I still get the issue This is so weird
I get it here
Graphics g = Graphics.FromHwnd(monitorHwnd);

Pointers are new to me so it's something I don't understand
its also a really old windows api so it is weird
idk how this happened
It may be the invalid window type...in terms of hwnd. But It fetches this hwnd so not sure...
After evaluating the variables, this is the variable value of
monitorHwnd
= 0x00010003
Inside graphics gim also busy doing other stuff now so i cant help you too much rn
Ok thats fine. You provided a lot of help already. I will keep trying and will let you know of my findings as I make progress
Managed to solve this. Thank you very much @297 лаyс ынтил hаллоwээн 👻
A problem that took 4 days...my word 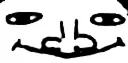
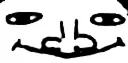
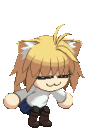

Yeah it does, I can agree