The value '' is invalid. for primary key in MVC
I'm practicing MVC with asp.net and building a simple todo app. I have my Model:
and MVC controller:
and my view _Form.cshtml:
When I try to run the program, the data is not written into my database and I also get the error
The value '' is invalid
, when I run the program in debug, the error cause from Id value, from what I found on the internet, people that had this error only cause with other attribute, but not primary key as mine. So I'm frustrated right now, because it's primary key, so I cannot just make it nullable or assign a default value as the normal attribute.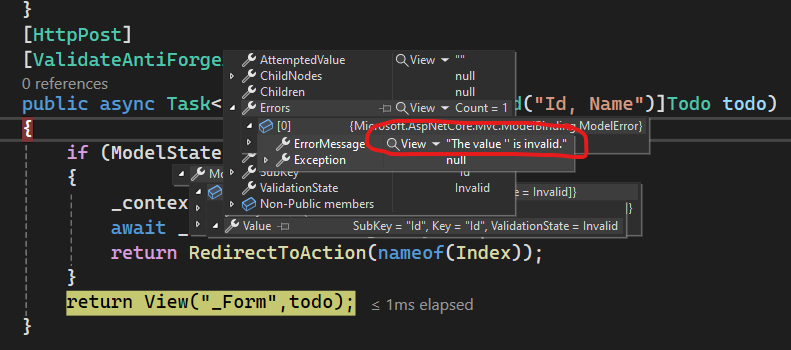
15 Replies
For the primary in my database, I believe it has all the standards of a primary key
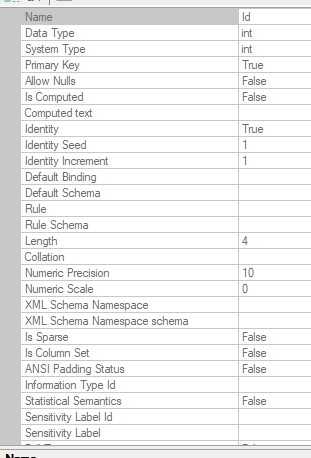
You're adding a new todo with the primary key already defined instead of letting the database generate that key
Perhaps there's some conflict, like trying to insert an item with ID that already exists
not likely, because there's no record ever inserted into my database, but I think it might has something to do with the id value when my method get is 0 when I try to run it ?
Could be
I don't think
0
is a valid PK
Again, it would be best if you just let the database generate themhow could I do that from my method? Excluding the id binding ?
you could create a custom dto for that cause the id isn't useful in your form
First of all, don't pass the database model around and use a DTO
could you give me more detail ? I dont think I understand you entirely 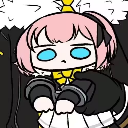
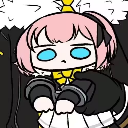
I'm not even sure you need the FromForm these day 🤔
we dont need [HttpPost] and [ValidateAntiForgeryToken] anymore ?
I didn't remove them
oh wait, mb I was
code actually works, ty a lot, I'll try to see the different and learn 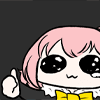
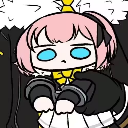
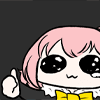