Basic Router
Hey there I want to have a simple Router component that I can use. Problem I'm curently facing is that it doesn't rerender on path change.
8 Replies
maybe the switch can be inside the jsx
your
switch
isn't inside a reactive context, so it's only being ran once. put it in a createMemo or inside a jsx fragment@CC8 , your code would be fine in react, which reruns the entire component, and thus can have multiple return statements. In solid, the top part of the component (the setup) is only executed once, so it's only the first return statement that will be hit (the render function). That's why as bendonovich says, your control flow has to be either inside a reactive context made in the setup part (like memo), or inside the render function itself.
so i should so something likethis ?
either that, or something like this
alright thanks
now do you know a way I can export the navigate function because I want to use it in other components without passing it through props
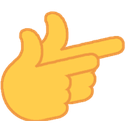
I believe you can just move
const [path, setPath] = createSignal(window.location.pathname)
and
outside the component, and write export
in front of them
or in case the window.location.pathname is troublesome so it's only the navigate that's moved out, simply add the path and setpath signals to the argument list of navigate
export const navigate = (to, path, setPath) => {...}
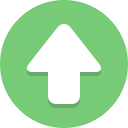