Dictionary cant find via ContainsKey but it does exist
Am i dumb or are dictionaries broken
How could this even happen?
I see via the debugger that the key i want is in the dictionary but when i use ContainsKey it doesnt find it, huh? Im real confused
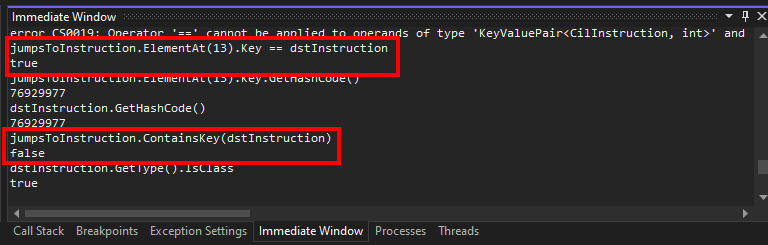
7 Replies
This is the dictionary in question:
the
CilInstruction
type is from the library AsmResolver
which does have its own Equals
and GetHashCode
which when i manually check produces the correct results and the dict should find it based on thatHello, can you share a bit more about your code? Remember that the ContainsKey will use Object.Equals to find you key in the dictionary.
sure, what else is there to share? I think i shared everything
Just to clarify if i do:
They will both return true, so from what i understand this means that the methods are correctly overridden
What I want to see if how you hidratate your Dictionary and where are you trying to do the ContainsKey so I can see if your are doing something wrong
So far what I understood is:
ah yea, well sadly its not 5 lines but here it is:
this is where the lookup gets populated
and this is where it is being read
The call of the 2nd function is quite complex so i cant really put it here, but at the core its basically itterating a List with CilInstruction items
Without debbugging will be hard to find. This is what ContainsKey will execute:
Looking at you code and so far seems to be that the issue is on runtime so I can't go any further without debugging. I will sugest write a test and reproduce it
If the Tkey in your case CilInstruction has an implemented IEqualityComparer, I understood you toldme they have a custom one, it will use the customComparer (which I understand it works properly)
i guess ill just use the wanky workaround, its not performance critical so its ok