Change function behavior depending on class' generic type.
Hello! I was wondering if there was a way to change the behavior of a function in a class with a generic type. I was originally looking into overloading but didn't have any luck. Is what I'm trying to do possible?
10 Replies
you'd have to compare
typeof(T)
to the types you're interested in
what's the end goal? if you're only interested in numbers you can use the INumber<T>
interface as a constraint(
Color
is from Microsoft.Xna.Framework
)
Can't, since if i have it inside of the function, the compiler won't be able to convert T
into Color
.yeah that's about all you can do then, though you could also pattern match on
_value1
or _value2
since you have those available
e.g. if (_value1 is Color color1)
Still having a conversion issue, mainly with setting
color1
to _value1
.sounds like a job for extensions.
Color.Lerp()
is already a member of the class.
...I don't understand this answer.you might have to do some sketchy unsafe things to force this pattern to work 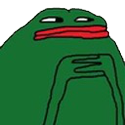
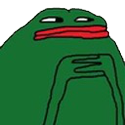
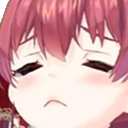
like
Unsafe.As
which is a type cast that ignores any safety checksI'll just do it the original way I had planned, making inherited classes like 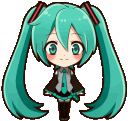
LerpableColor : Lerpable<Color>
.
Thank you for the help though. 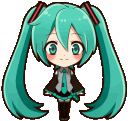