Could someone help me with this task
So I've been struggling with this task for some hours now. Basically I need to add the functionality for borrowing and returning books from a library. Thanks to anyone willing to help.
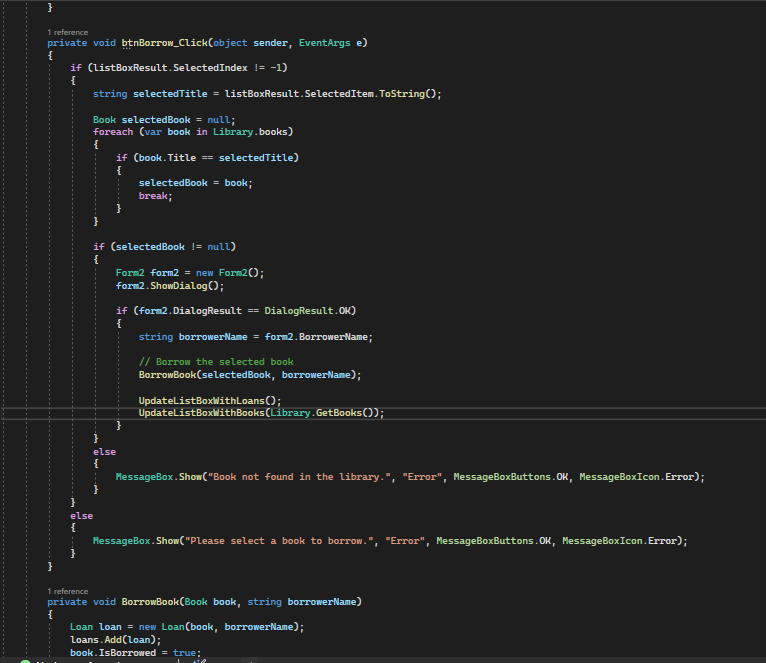
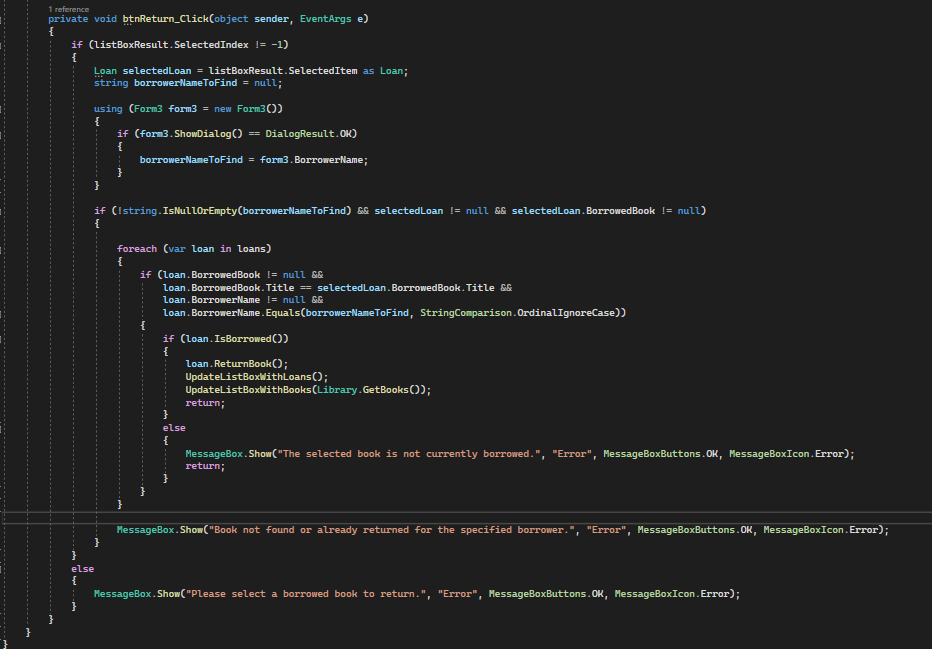
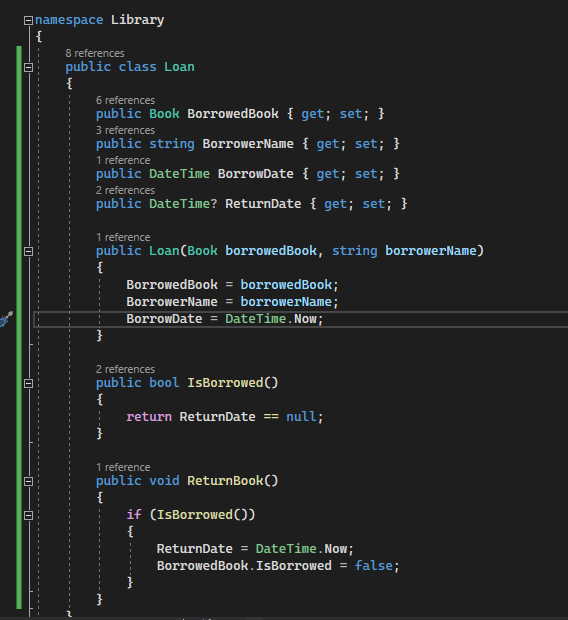
12 Replies
$details
When you ask a question, make sure you include as much detail as possible. Such as code, the issue you are facing, and what you expect the result to be. Upload code here https://paste.mod.gg/ (see $code for more information on how to paste your code)
What exactly doesn't work?
Basically it does not store whether the book is borrowed or not, and due to that, it always returns the error messagebox saying: "Book not found or already returned for the specified borrower."
So basically it should record the borrowerName and the BorrowTime, then check in the loans if there is a borrowedBook with the name that matches the selected item's name in the listBox
need to see more of your code to help
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/cs
cs
using LINQtoCSV;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Library
{
[Serializable]
public enum Genre
{
All,
Fantasy,
Detective,
Fiction,
Thriller,
Biography
}
public class BookClass
{
[CsvColumn(FieldIndex =1)]
public string Title { get; set; }
[CsvColumn(FieldIndex =2)]
public string Author { get; set; }
[CsvColumn(FieldIndex = 3)]
public string Description { get; set; }
[CsvColumn(FieldIndex = 4)]
public Genre Genre { get; set; }
}
}
using LINQtoCSV;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Library
{
[Serializable]
public enum Genre
{
All,
Fantasy,
Detective,
Fiction,
Thriller,
Biography
}
public class BookClass
{
[CsvColumn(FieldIndex =1)]
public string Title { get; set; }
[CsvColumn(FieldIndex =2)]
public string Author { get; set; }
[CsvColumn(FieldIndex = 3)]
public string Description { get; set; }
[CsvColumn(FieldIndex = 4)]
public Genre Genre { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Library
{
public partial class Form2 : Form
{
public string BorrowerName { get; set; }
public Form2()
{
InitializeComponent();
}
private void btnBorrow_Click(object sender, EventArgs e)
{
string borrowerName = textBoxName.Text;
if (!string.IsNullOrEmpty(borrowerName))
{
MessageBox.Show($"The book has been borrowed by {borrowerName} at {DateTime.Now}.", "Borrowed");
this.DialogResult = DialogResult.OK;
}
this.Close();
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Library
{
public partial class Form2 : Form
{
public string BorrowerName { get; set; }
public Form2()
{
InitializeComponent();
}
private void btnBorrow_Click(object sender, EventArgs e)
{
string borrowerName = textBoxName.Text;
if (!string.IsNullOrEmpty(borrowerName))
{
MessageBox.Show($"The book has been borrowed by {borrowerName} at {DateTime.Now}.", "Borrowed");
this.DialogResult = DialogResult.OK;
}
this.Close();
}
}
}
using System.Collections.Generic;
namespace Library
{
public class Library
{
public enum Genre
{
All,
Fantasy,
Detective,
Fiction,
Thriller,
Biography
}
public static List<Book> books = new List<Book>();
public static List<Book> GetBooks()
{
return books;
}
public static void AddBook(Book book)
{
books.Add(book);
}
public static void RemoveBook(Book book)
{
books.Remove(book);
}
}
}
using System.Collections.Generic;
namespace Library
{
public class Library
{
public enum Genre
{
All,
Fantasy,
Detective,
Fiction,
Thriller,
Biography
}
public static List<Book> books = new List<Book>();
public static List<Book> GetBooks()
{
return books;
}
public static void AddBook(Book book)
{
books.Add(book);
}
public static void RemoveBook(Book book)
{
books.Remove(book);
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Library
{
public partial class Form3 : Form
{
public string BorrowerName { get; set; }
public Form3()
{
InitializeComponent();
}
private void btnReturn1_Click_1(object sender, EventArgs e)
{
BorrowerName = textBoxBorrowerName.Text;
if (!string.IsNullOrEmpty(BorrowerName))
{
DialogResult = DialogResult.OK;
}
else
{
MessageBox.Show("Please enter the borrower's name.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Library
{
public partial class Form3 : Form
{
public string BorrowerName { get; set; }
public Form3()
{
InitializeComponent();
}
private void btnReturn1_Click_1(object sender, EventArgs e)
{
BorrowerName = textBoxBorrowerName.Text;
if (!string.IsNullOrEmpty(BorrowerName))
{
DialogResult = DialogResult.OK;
}
else
{
MessageBox.Show("Please enter the borrower's name.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
}
}
using System;
using System.Collections.Generic;
namespace Library
{
public class Book
{
public string Title { get; set; }
public string Author { get; set; }
public string Description { get; set; }
public bool IsBorrowed { get; set; }
public Genre genre;
public Book(string title, string author, string desc, Genre genre)
{
Title = title;
Author = author;
Description = desc;
this.genre = genre;
IsBorrowed = false;
}
}
}
using System;
using System.Collections.Generic;
namespace Library
{
public class Book
{
public string Title { get; set; }
public string Author { get; set; }
public string Description { get; set; }
public bool IsBorrowed { get; set; }
public Genre genre;
public Book(string title, string author, string desc, Genre genre)
{
Title = title;
Author = author;
Description = desc;
this.genre = genre;
IsBorrowed = false;
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Library
{
public class Loan
{
public Book BorrowedBook { get; set; }
public string BorrowerName { get; set; }
public DateTime BorrowDate { get; set; }
public DateTime? ReturnDate { get; set; }
public Loan(Book borrowedBook, string borrowerName)
{
BorrowedBook = borrowedBook;
BorrowerName = borrowerName;
BorrowDate = DateTime.Now;
}
public bool IsBorrowed()
{
return ReturnDate == null;
}
public void ReturnBook()
{
if (IsBorrowed())
{
ReturnDate = DateTime.Now;
BorrowedBook.IsBorrowed = false;
}
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Library
{
public class Loan
{
public Book BorrowedBook { get; set; }
public string BorrowerName { get; set; }
public DateTime BorrowDate { get; set; }
public DateTime? ReturnDate { get; set; }
public Loan(Book borrowedBook, string borrowerName)
{
BorrowedBook = borrowedBook;
BorrowerName = borrowerName;
BorrowDate = DateTime.Now;
}
public bool IsBorrowed()
{
return ReturnDate == null;
}
public void ReturnBook()
{
if (IsBorrowed())
{
ReturnDate = DateTime.Now;
BorrowedBook.IsBorrowed = false;
}
}
}
}
A bit unrelated but one tip for better readability.
Use early returns.
For example this method
Could be refactored to
Now you can read the method from top to bottom and know what is happening and you don't have to skip around the code to look at the else statements.
It also decreases your nesting.
As far as I see this message can only come from
is false for all loans, or if there are no loans to begin with.
You should check if that if case is correct and behaves the way you want it too.
c#
private void btnRemove_Click(object sender, EventArgs e)
{
if (listBoxResult.SelectedIndex != -1)
{
string selectedTitle = listBoxResult.SelectedItem.ToString();
Book selectedBook = null;
foreach (var book in Library.books)
{
if (book.Title == selectedTitle)
{
selectedBook = book;
break;
}
}
if (selectedBook != null)
{
Library.RemoveBook(selectedBook);
UpdateListBoxWithBooks(Library.books);
List<BookClass> booksForTxt = new List<BookClass>();
foreach (var book in Library.books)
{
BookClass bookClass = new BookClass
{
Title = book.Title,
Author = book.Author,
Description = book.Description,
Genre = book.genre
};
booksForTxt.Add(bookClass);
}
WriteTxtFile(booksForTxt);
}
else
{
MessageBox.Show("Book not found in the library.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
else
{
MessageBox.Show("Please select a book to remove.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
c#
private void btnRemove_Click(object sender, EventArgs e)
{
if (listBoxResult.SelectedIndex != -1)
{
string selectedTitle = listBoxResult.SelectedItem.ToString();
Book selectedBook = null;
foreach (var book in Library.books)
{
if (book.Title == selectedTitle)
{
selectedBook = book;
break;
}
}
if (selectedBook != null)
{
Library.RemoveBook(selectedBook);
UpdateListBoxWithBooks(Library.books);
List<BookClass> booksForTxt = new List<BookClass>();
foreach (var book in Library.books)
{
BookClass bookClass = new BookClass
{
Title = book.Title,
Author = book.Author,
Description = book.Description,
Genre = book.genre
};
booksForTxt.Add(bookClass);
}
WriteTxtFile(booksForTxt);
}
else
{
MessageBox.Show("Book not found in the library.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
else
{
MessageBox.Show("Please select a book to remove.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
c#
private void btnBorrow_Click(object sender, EventArgs e)
{
if (listBoxResult.SelectedIndex == -1)
{
MessageBox.Show("Please select a book to borrow.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
string selectedTitle = listBoxResult.SelectedItem.ToString();
Book selectedBook = null;
foreach (var book in Library.books)
{
if (book.Title == selectedTitle)
{
selectedBook = book;
break;
}
}
if (selectedBook is null)
{
MessageBox.Show("Book not found in the library.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
Form2 form2 = new Form2();
form2.ShowDialog();
if (form2.DialogResult == DialogResult.OK)
{
string borrowerName = form2.BorrowerName;
BorrowBook(selectedBook, borrowerName);
UpdateListBoxWithLoans();
UpdateListBoxWithBooks(Library.GetBooks());
}
}
c#
private void btnBorrow_Click(object sender, EventArgs e)
{
if (listBoxResult.SelectedIndex == -1)
{
MessageBox.Show("Please select a book to borrow.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
string selectedTitle = listBoxResult.SelectedItem.ToString();
Book selectedBook = null;
foreach (var book in Library.books)
{
if (book.Title == selectedTitle)
{
selectedBook = book;
break;
}
}
if (selectedBook is null)
{
MessageBox.Show("Book not found in the library.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
Form2 form2 = new Form2();
form2.ShowDialog();
if (form2.DialogResult == DialogResult.OK)
{
string borrowerName = form2.BorrowerName;
BorrowBook(selectedBook, borrowerName);
UpdateListBoxWithLoans();
UpdateListBoxWithBooks(Library.GetBooks());
}
}
btnReturn_Click(object sender, EventArgs e)
.
Did you go in there with a debugger and stepped through the code?
The only possible way to reach those code in this method is if this condition
c#
if (loan.BorrowedBook != null &&
loan.BorrowedBook.Title == selectedLoan.BorrowedBook.Title &&
loan.BorrowerName != null &&
loan.BorrowerName.Equals(borrowerNameToFind, StringComparison.OrdinalIgnoreCase))
c#
if (loan.BorrowedBook != null &&
loan.BorrowedBook.Title == selectedLoan.BorrowedBook.Title &&
loan.BorrowerName != null &&
loan.BorrowerName.Equals(borrowerNameToFind, StringComparison.OrdinalIgnoreCase))
After some more hours of work I have fixed everything)
Thank you for trying to help tho)
$close
Use the /close command to mark a forum thread as answered