EF Core Nested Projections
I am trying to find out a good way to perform a projection on a model with multiple relationship levels. Here is an example:
This works but I have a lot more levels in my code and writing this out every time is super tedious. I'l looking for a way to reuse the projections so I don't have to write them out every time.
7 Replies
I found two suggestions:
Explicit Operator
This makes life a lot easier but unfortunately I found out that the SQL generated by this does not use projection but always gets the all properties.
Expression Functions
But this gives me the following error:
Can you elaborate on this
This works but I have a lot more levels in my code and writing this out every time is super tedioushow many places are you having to write the same code?
Mapperly can generate reusable projections for you, maybe take a look what their Source Gen outputs?
For example I have a lot more Models that have a relationship with User so I need to write the same code multiple times for every relationship
@Joschi As far as I can see the mapper just auto generates mappings https://mapperly.riok.app/docs/getting-started/generated-mapper-example/#the-generated-code
But I don't think I can directly use it in the projection.
The next best thing I found was this:
https://mapperly.riok.app/docs/configuration/queryable-projections/
But I will run into the same issue there because I would have to do something like this:
I mean how many entities do you actually have? I feel like you're overthinking to avoid writing some basic mapping code.
Here is one of them: Yes I can copy paste but I feel like it is error prone. Especially when I have to add/remove properties and the forget to update it somewhere
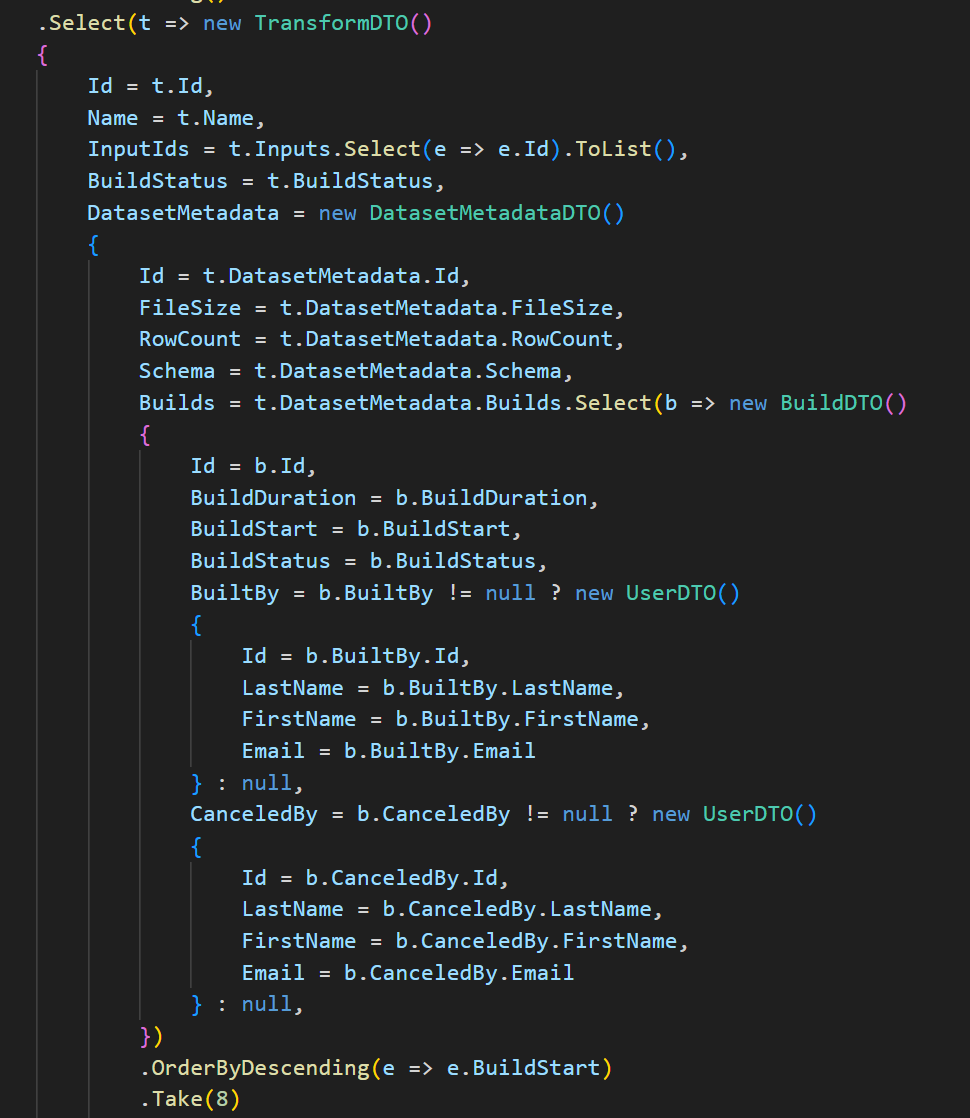
I don't see the problem.
Unless you want different sets of properties each time, but then I guess you will have to do it by hand.