how to upload a file in .net project
Im trying to fill a form with some attributes but when it comes to select a photo it doesnt work , who may cause the problem ?
108 Replies
in fact , when i debug all fields are valid , only ImageUrl is Invalid
ASP.NET Core I assume?
Show your $code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/To send a file you would usually use
IFormFile
yes im actually using , only the imageurl attribute is invalid now ill send the code
this is the method in my controller
and this is the form im trying to sumbit :
and the Punet model :
in my wwwroot folder i have a subfolder "image/fotot" , when i want to save the photos
but this attribute isnt validating
when i Debug to if(ModelState.isValid) all fields are Valid only ImageUrl is Invalid ?!
It's... not how I'd write most of the code, but aight. First and foremost, I'd use the viewmodel as actual viewmodel, not a useless wrapper around the database model
That way you can have your
IFomFile
there and bind to itso which is the best way to store this and submit the form cuz im stuck
i want to display the image too
Does the file actually get saved?
Does it appear where it should, and does the database entry look like it should?
nah just the form repeats itself with all fields filled only imageurl isnt filled and says choose one file , so isnt submited
So modelstate is not valid?
and no photos are going to images/fotot
no its not valid
cuz of image
when i Debug to if(ModelState.isValid) all fields are Valid only ImageUrl is Invalid ?!
I guess I'd start by making the viewmodel an actual viewmodel, then
so what to change to the code
i think Upsert Method isnt functional
The VM should contain everything you need, and only the things you need
so you think this one to put in my ViewModel folder
Then you can bind your file field to it
but when i access to the PunetVM i have this attribute : public Punet Punet { get; set; }
Well, don't
As I said
A viewmodel should be a viewmodel
Not a wrapper for the database model
okay but IFormFile
how will it save in the database
as a string ?
Well, in your viewmodel, you will get the file
You will then take it, save it somewhere on disk, like you're doing now
Then you will get a path to it, on disk
And save that in the database
let me try it
You will create a new
Punet
, and save that in the db
The PunetVM
will, at no point whatsoever, even touch the databaseso this code that i wrote wont work in anyway?
The file saving code and the rest is fine
It's just what you bind to is the issue
That you're trying to use a wrapped up database model instead of an actual VM
The rest looks fine
so in this file that i have i should pass all the attributes that u send
Yes
Thew viewmodel should describe the data you want to send in or out
It should be completely separate from the database models
Id is required or not ?
I would assume your database generates the IDs on insert, doesn't it?
yes it does
okay
now there are error after i use the method u sent :
file doesnt exist in the current context
Well, your
punetVM
no longer contains a Punet
yes true
It does contain the file, though
So, no, there is not an individual
file
parameter
There's a punetVM.File
so instead to use
Yep
this one : punetVM.Punet.ImageUrl = @"\images\fotot" + fileName; cuz it shows errors
instead of Punet ?
Ah, well, you have a viewmodel, but you don't have the database model now, right?
You will have to create it, based on the viewmodel
i did this : punetVM.File = @"\images\fotot" + fileName;
For example,
punetVM
is not your database model
It will not, at any point, touch your database
Punet
is yor database modelno only Punet is in my database
Correct
So you will need to create a new instance of
Punet
and fill it with data
Some of that data you can take directly from the VM
Some of it, you will have to create based on that. Like the image URLso what should i change to this method :
then i should change to Upsert.cshtml
Ah, wait, it's an upsert
In that case, I guess the viewmodel should have an ID as well
And... I told you what to do
Create a punet based on the vm
For example like here
Then you can
_unitOfWork.Punet.Add(punet)
Or _unitOfWork.Punet.Update(punet)
But you need to create that punet first
Based on the data from the VM
And the data you create later, like a URL to the filecan i share the screen cuz im new to this and dont understands those concepts so clearly
if u have some time
free
okay now im adding other attributes
so i shouldnt include imageUrl attribute to the imageUrl
?
Also, just so we're clear, the
[HttpPost]
you have there is an example of an attribute
What you mean is, I assume, a property
$structureJust so you're aware of the nomenclature
Correct
At this point in time, you don't have the URL after all
You have to save the file first, then get its location
Only then can you set
.ImageUrl
property of your punet
but i have some other errors in my Upsert Method which accepts a parameter int? id
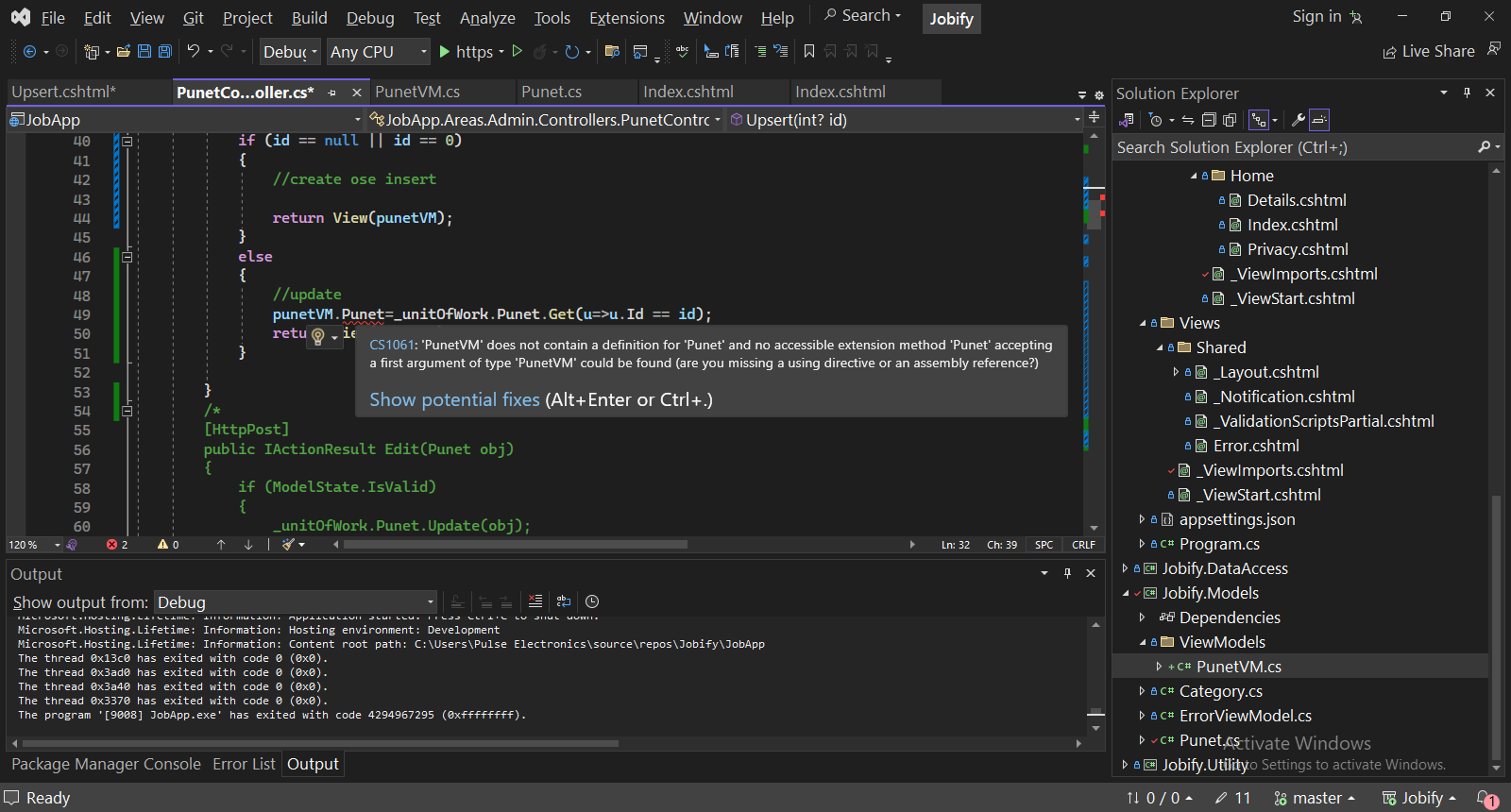
Well, yeah
Your VM now looks different
Again
Again
Again
It no longer contains a
Punet
It has its own set of properties
That you need to set
So, in this case, you would do the inverse
Instead of creating a punet based on the VM, you would create the VM based on your punet
in this : , should i make this one
Yes, my bad
and those :
is completed ?
should i include the image ?
Do you want to display the image?
If so, then yes, you should add a property with the image's URL
but
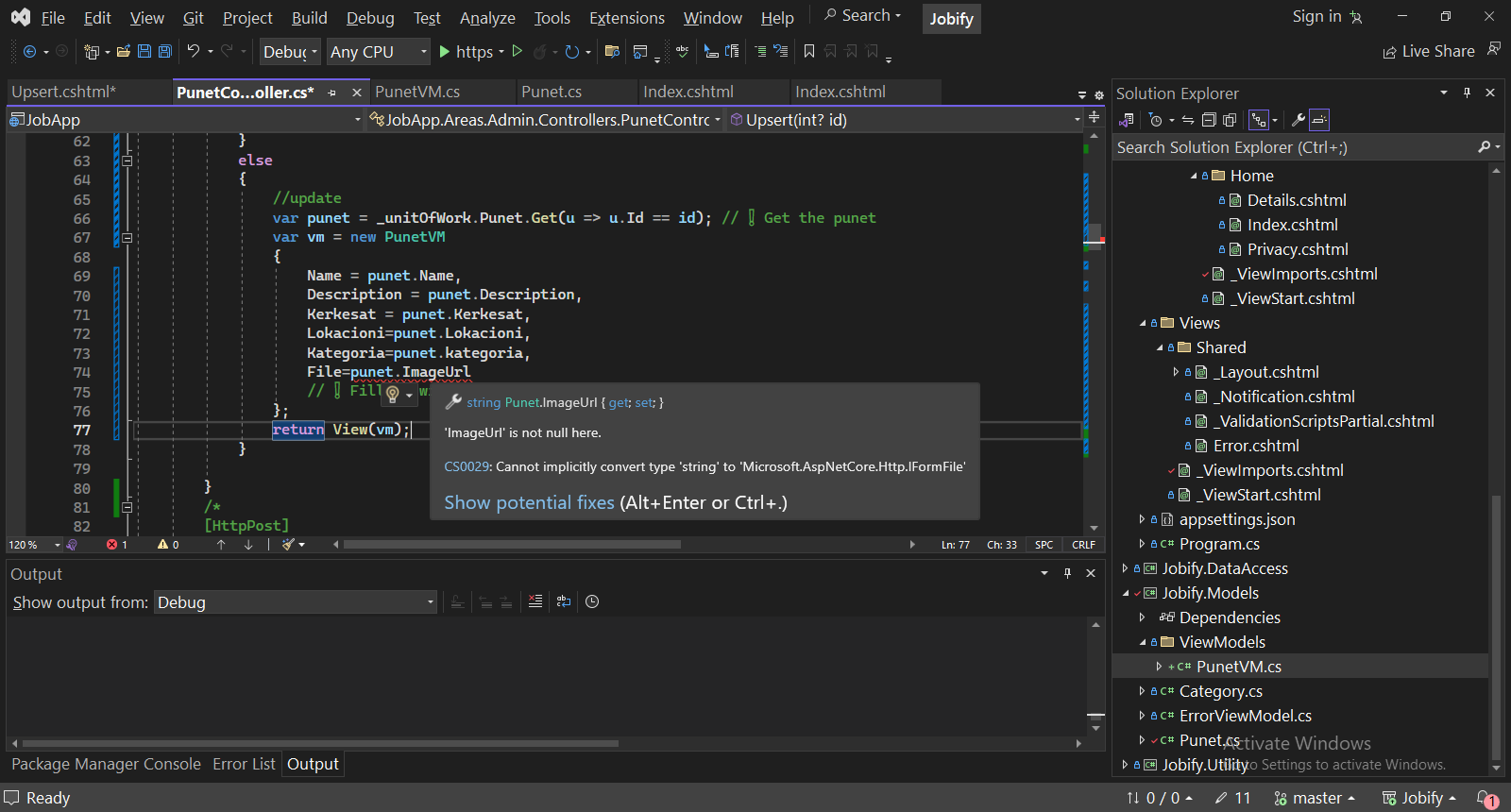
should i cast to smth
how to convert this
im trying like this: File = (IFormFile)punet.ImageUrl
but it doesnt work
🥺
@jIMMACLE
so where are you trying to display an image?
and why do you think that means you need to cast a string to an IFormFile?
cuz it wont compile
cuz i need to cast or smth
have you considered that that is a symptom of a more fundamental problem
why would casting a string to an IFormFile work?
a string is not an IFormFile
i know
so i'll ask for the 3rd time
where are you trying to display the image?
i have a Model called Punet , in this model i have some attributes and a file called ImageUrl as a string , then i have a ViewModel PunetVM and have the image as IFormFile , i want that image to display to Index.cshtml i mentioned above the conversation
but i cant cast from string to IFormFile
i have 2 methods Upsert
in my Controller
so how do you normally display an image in HTML?
in ,img tag
yes, and what do you put in the img tag to tell it to show a specific image?
src
and what does a
src
value look like?random url
yes
so you don't need an iformfile
you just need a url
look my code , i think its a bit complicated
.
it's not complicated
if you want to display an image that your website is serving, you need to provide the URL of the image to the page
which could be either a direct URL to a statically hosted image in wwwroot or a controller that can return the correct image based on something else
all the fields are submited successfully only image isnt
so when i debug
well you aren't asking me about submitting anything
you're asking me about displaying an image that's already on your site
im trying to display that
but i cant
cuz the form isnt
submited succesfully
Submitting an image ->
IFormFile
Displaying an image -> URL of that imagewell that's an entirely different problem
If you want a single viewmodel for both, it needs to contain both
IFormFile File
for submission
string Url
for displayso what to write instead of : File=punet.ImageUrl cuz i cant cast
You need another property
you don't use IFormFile for the response
A string property
period
Named
Url
or ImageUrl
or something
And put the URL of that image there
When uploading the image:
When displaying the image, so going the other way:
why is the request and response model shared anyway
im not understanding , im a bit frustrated
IFormFile is for submitting images from the frontend
a plain string with a URL is all you need to send an image back
in which place i need to change the code ?
please
data go browser to server -> you need iformfile
data go server to browser -> you need string url
im new as i mentioned
the part where youre trying to cast your URL to an IFormFile for no reason
Add a string property named
ImageUrl
to the viewmodelokay
When sending data server to browser set that property to the file url
Do NOT touch the
IFormFile
property thenvar vm = new PunetVM
{
Name = punet.Name,
Description = punet.Description,
Kerkesat = punet.Kerkesat,
Lokacioni = punet.Lokacioni,
Kategoria = punet.kategoria,
ImageUrl=punet.ImageUrl
// ❗ Fill it with data }; now there is ok i think i added ImageUrl to ViewModel
// ❗ Fill it with data }; now there is ok i think i added ImageUrl to ViewModel
Yes
now im gonna try this one
errors went away
in the upsert i removed "Punet.Name" only left "Name"
when i click "Krijo " button , still it doesnt work ?
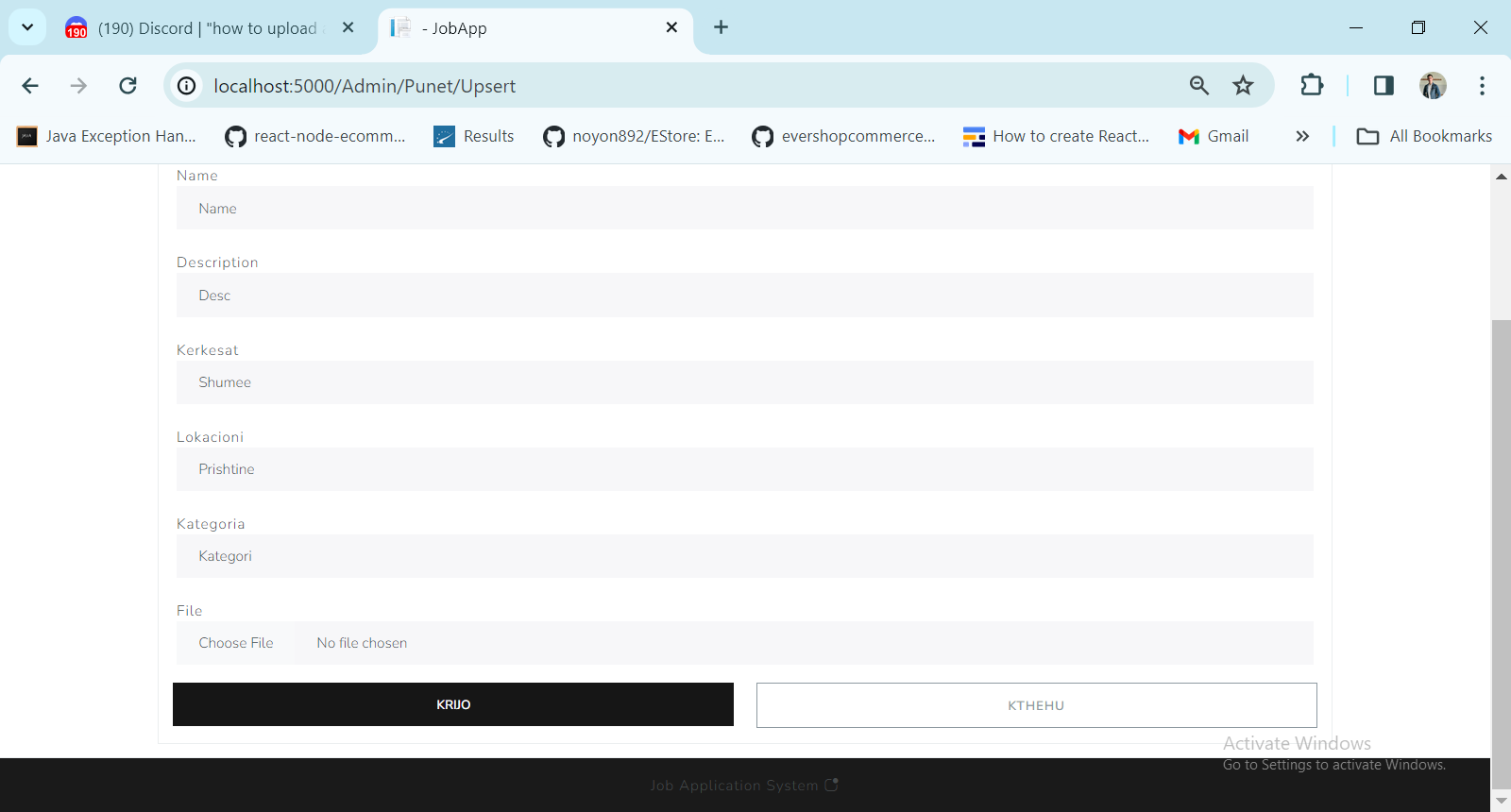
it repeats all the fields
Make sure the optional properties of the VM are nullable, I guess? So
Id
, File
, and ImageUrl
?
It probably complains because you don't send a new ImageUrl
value
And whether you send an ID is debatable
That's why I don't like upsert formsso you think to make one for insert one for update
That's how I'd do it, yes
it takes time i think
i have these methods
but i need to modify them
thank u anyway
respect