HTTPPut url
I'm praticing ASP.Net API, and in my program, I have a method that call Put request to update the api like this:
So when I run this method, it can do the update feature for my program and it return code 204, which is as what I wanted. But my question come when I change the line
[HttpPut("{categoryId}")]
to [HttpPut("{cateId}")]
. Now when I run it, my Swagger UI become a bit different, and when I tried to update the api, I got the error 404.
So what could be the reason for this cause ? And in case I want to use cateId
instead of categoryId
, where should I look at to make the change ?
p/s: I dont use categoryId
as variable for any thing else like dto or database table, if something like that, it would be CategoryId
instead.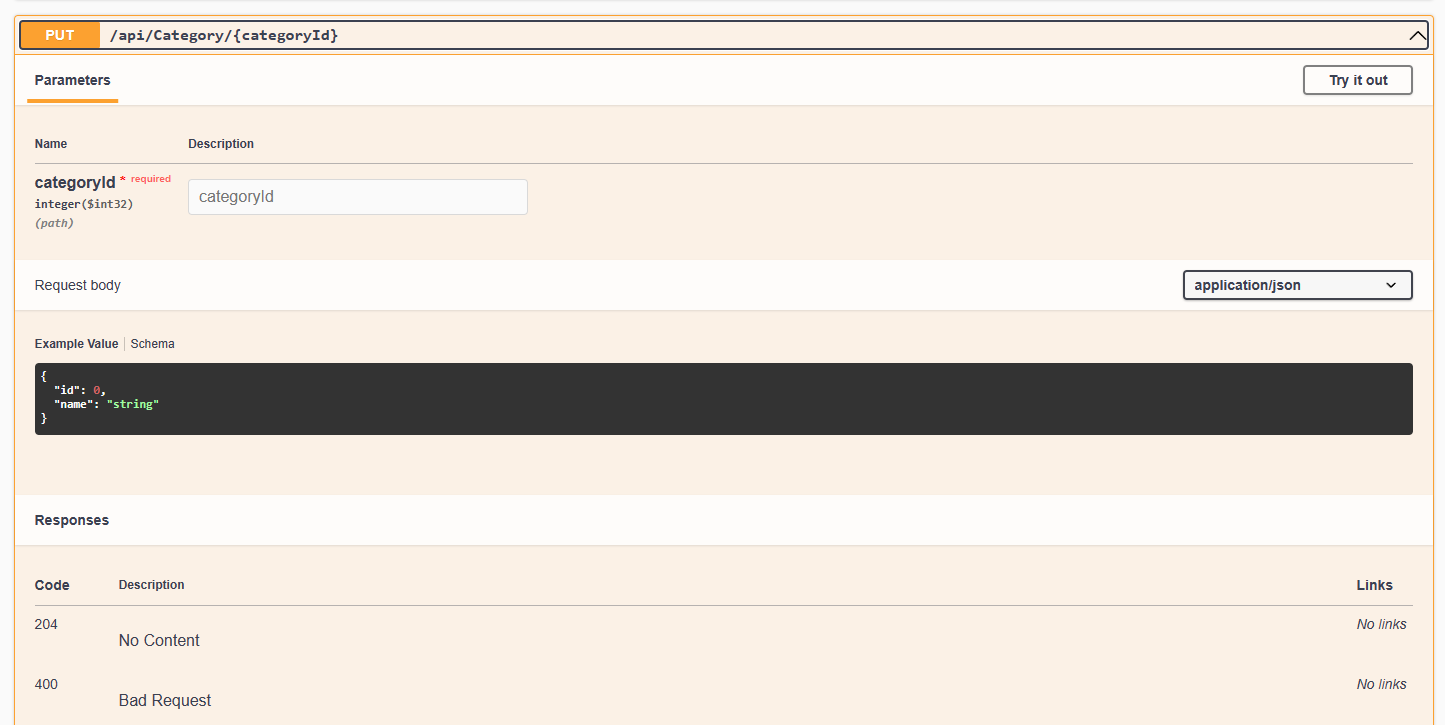
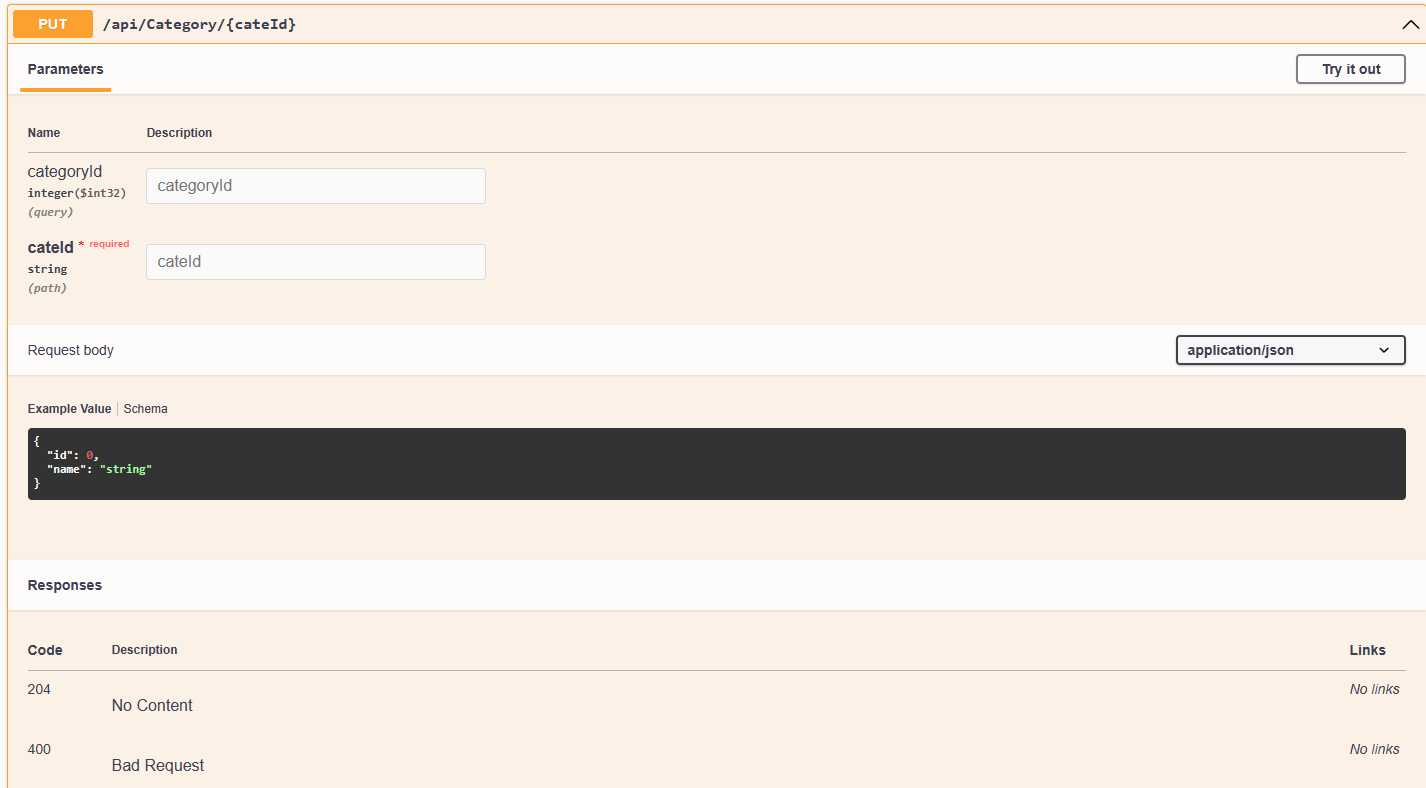
4 Replies
the 204 response with
[HttpPut("{categoryId}")]
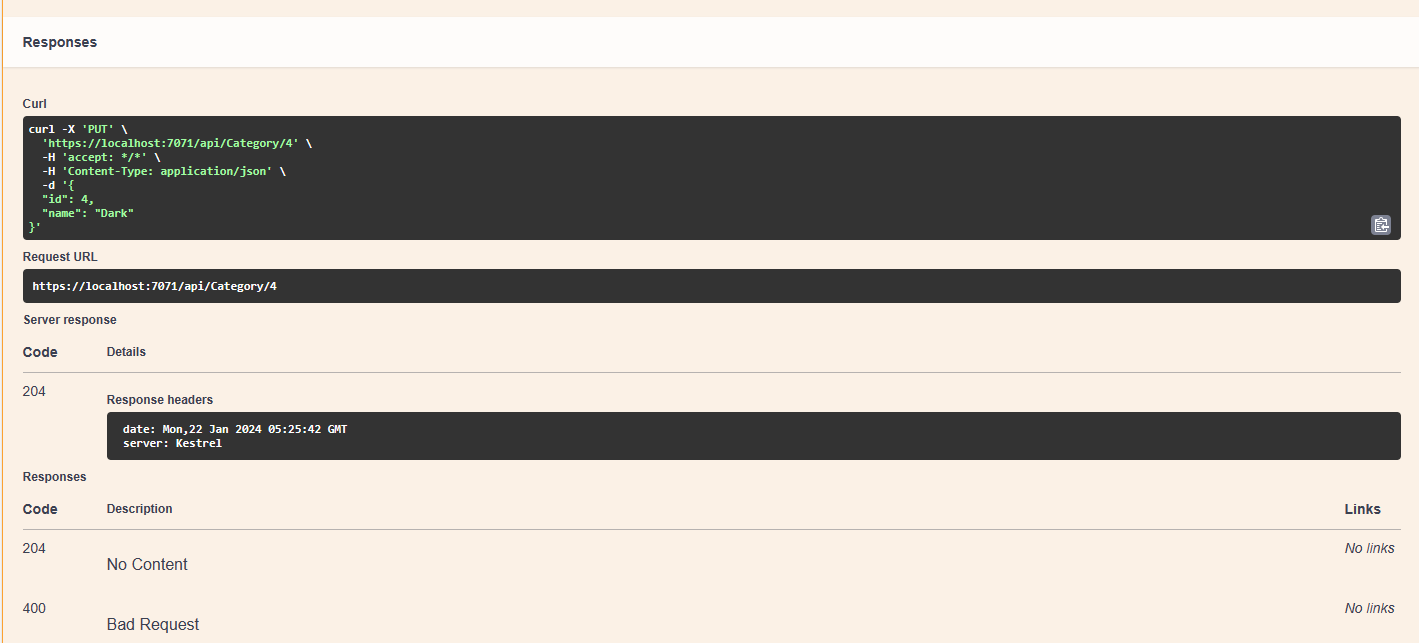
The 404 response with
[HttpPut("{cateId}")]
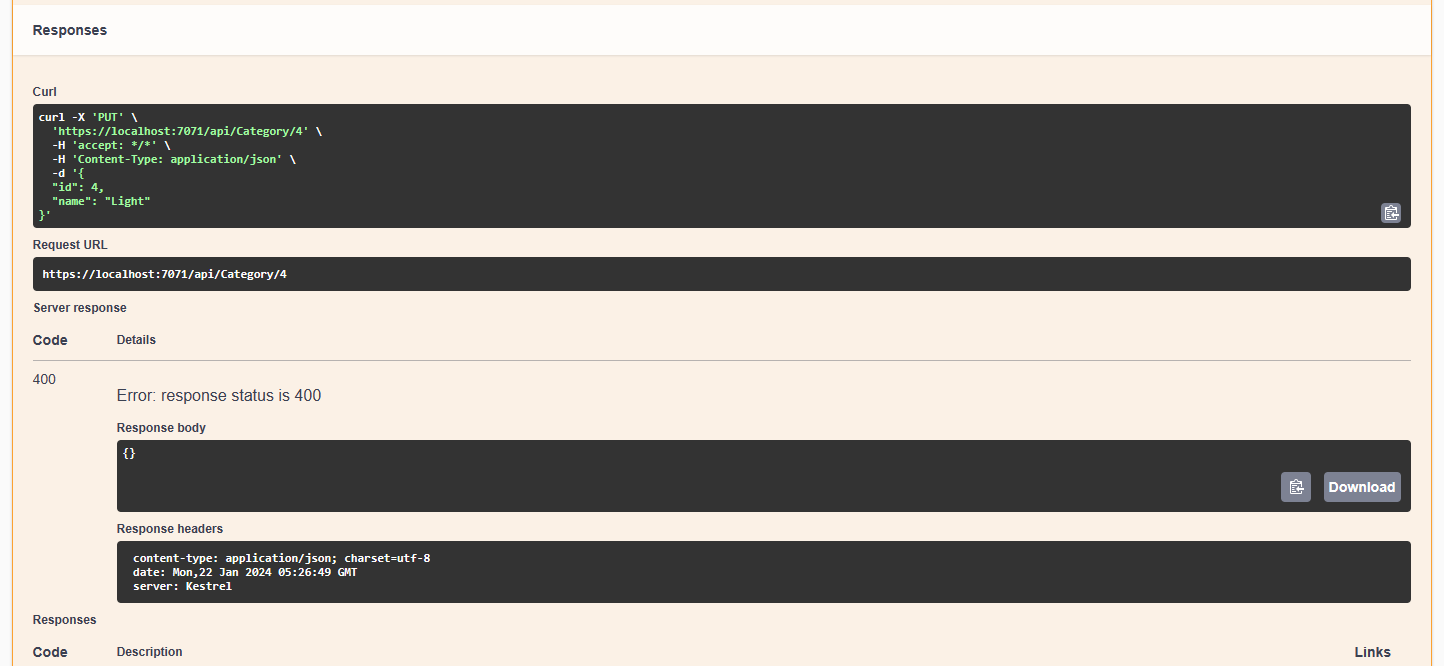
did you update the parameter name in the method signature?
because
categoryId
will be 0Hello,
I completely concur with the comment above, but there is something i do not understand. you have a CategoryDto. Why even bother to use a different Id and not use it within the DTO