Create a relationship manager for a pivot table
How do I create a relationship manager for a pivot table please?
Solution:Jump to solution
On
AmenityProperty
define 2 ->belongsTo()
relations (property
and amenity
). You should be able to access the data by property.name
then. Maybe pivot.property.name
or ->getStateUsing(fn ($record) => $record->pivot->property->name
18 Replies
The withPivot method returns an error
Share some code and the error
Please a little bit more and not just 3 lines 🙈
Not sure where you found
->withPivot()
as a table method. It is not. Those are Laravel relationship methods. And it is explained in the docs:
Please ensure that any pivot attributes are listed in the withPivot() method of the relationship and inverse relationship.
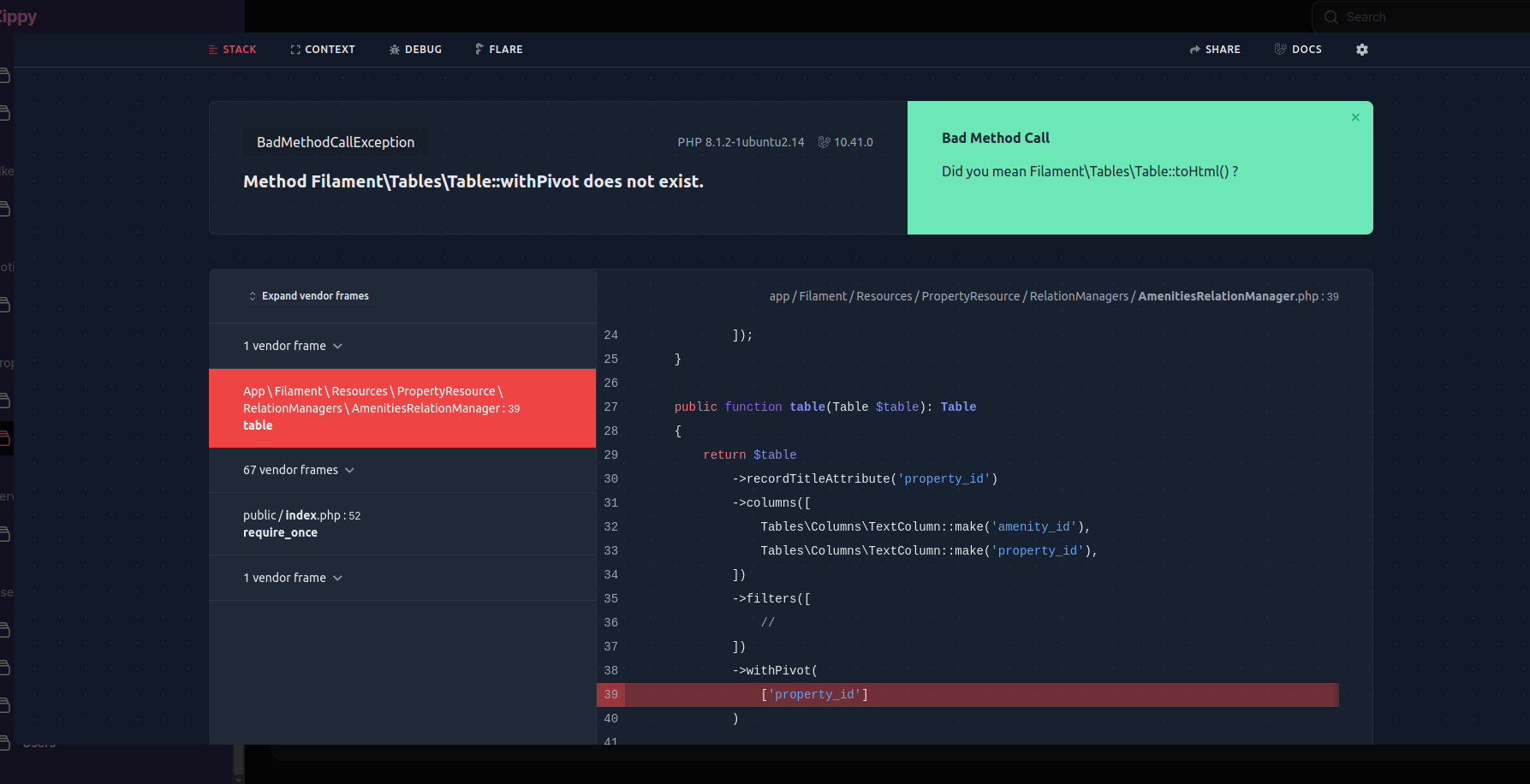
ok my bug let first check it out
Right now I have added the pivot on to the modal =>
But I want to retrieve the actual names not the ids any ideas on how best i can achieve that
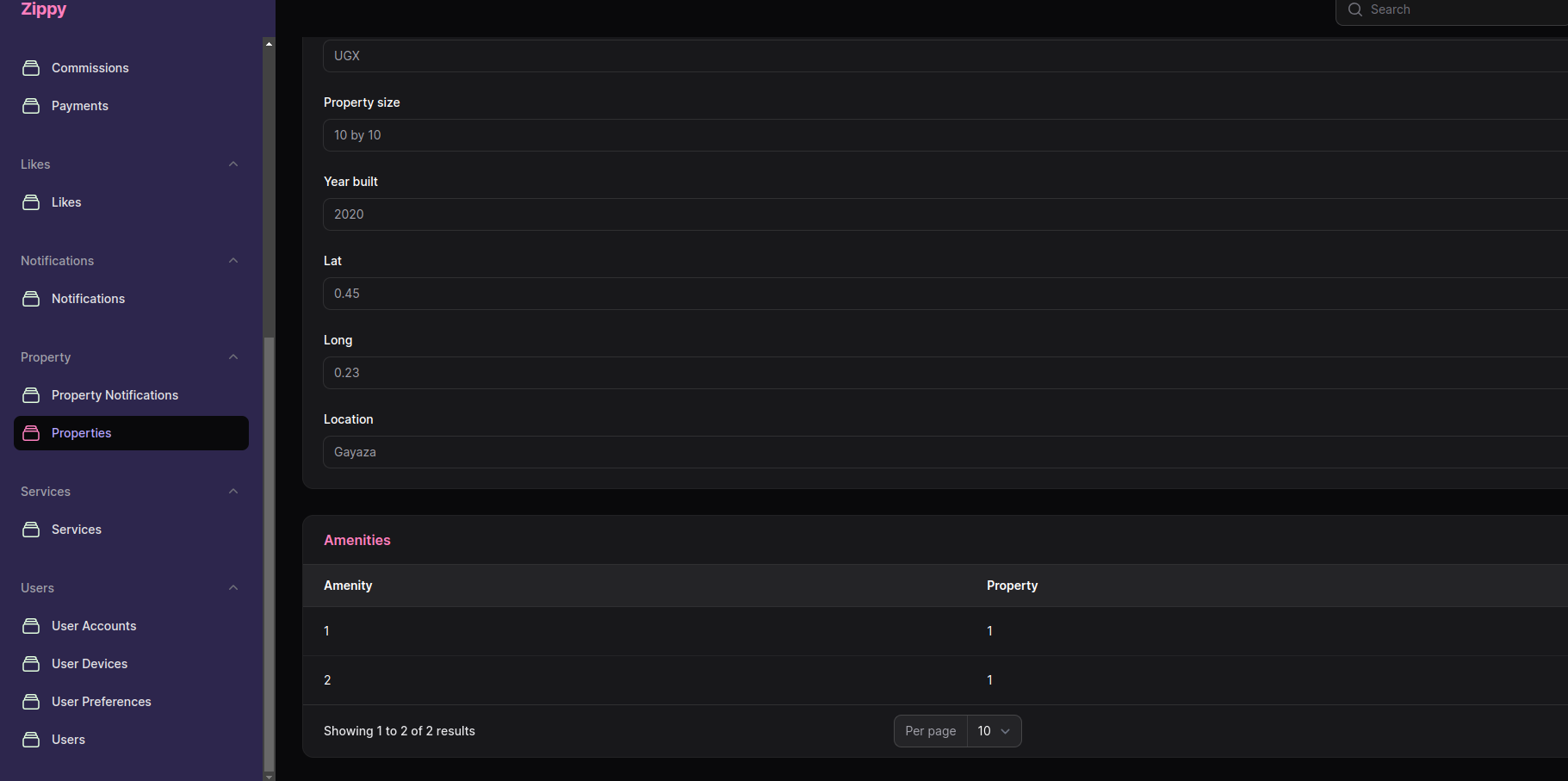
Create a pivot model and define relationships on that pivot model
My pivot modal
For some reason I get this error =>
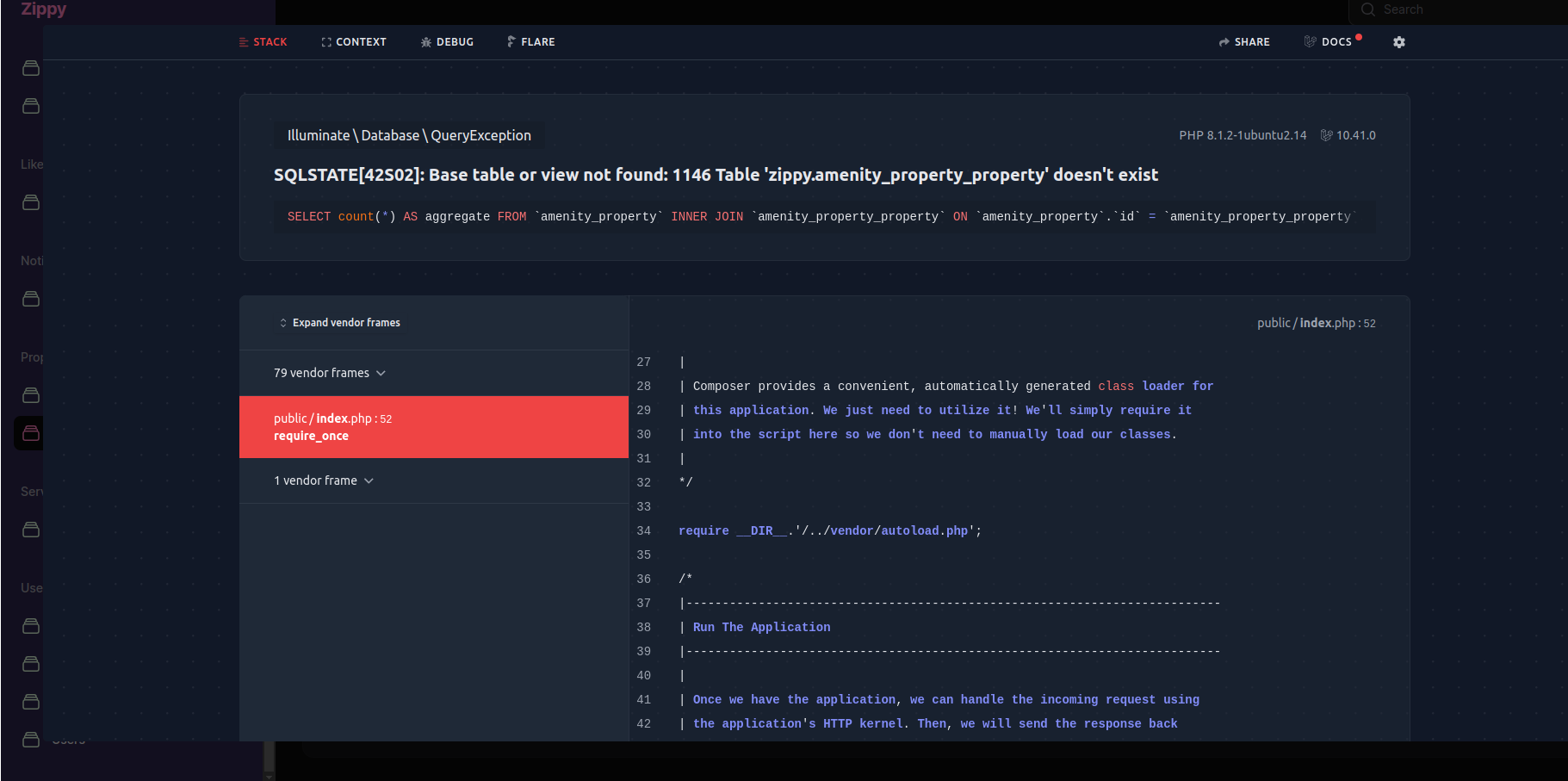
My Relationship Manager
Where do you think am going wrong
That's not how it works. See the Laravel docs:
https://laravel.com/docs/master/eloquent-relationships#defining-custom-intermediate-table-models
Laravel - The PHP Framework For Web Artisans
Laravel is a PHP web application framework with expressive, elegant syntax. We’ve already laid the foundation — freeing you to create without sweating the small things.
After reading through I have made an update now
thats for the property modal
sorry this
Now it does not crush as before
But i need to get the actual amenity and property name
Solution
On
AmenityProperty
define 2 ->belongsTo()
relations (property
and amenity
). You should be able to access the data by property.name
then. Maybe pivot.property.name
or ->getStateUsing(fn ($record) => $record->pivot->property->name
Thank you so much
it works