Parse Rust Enum Json in C#
Rust enums are a cool feature for Rust devs, but now that I gotta parse them in C# I hate them.
I got a JSON input that looks like this:
Key1, Key2, Key3 are 3 possible Enum values in Rust. But I cannot seem to find any reasonable way to parse this in C#.
Yes I could write a JsonConverter for this simple case, in practice I am working on a source generator that has to work on any input where often times enums like this are nested in each other and having to create a JsonConverter for that entire tree is just pain :/
18 Replies
hm
that json is an object with three properties - not a structure I'd expect for an object deserialized with a rust enum value on it
Well, some more context. The input for my source generator is a Json schema. The schema that allows for this JSON comes from an enum looking like this
https://i.imgur.com/vR1DgE0.png
Ah I see, so this is the schema and not an actual value
that complicates things 😛
Yeah 😅
I was about to suggest using
[JsonDerived]
but that wont help here thenYeah already looked at that. There isn't a clear discriminator to use here
exactly
dunno if you can get around this without a custom converter then
Well only if I go and parse the entire tree with the custom converter. This is a simple case cause there's just primitives in the enum. But that is not a requirement, there can be more objects and more such enums inside one.
And at that point complexity of the generator just explodes
Not something I would be comfortable with generating in a source gen
true
Rust, why you gotta do this to me 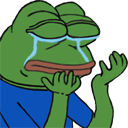
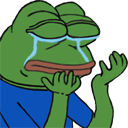
more like, C# why you no have proper DUs yet?
but yeah, its an awkward structure to parse
I think I have something
Parse with JsonDocuments, then do some processing, but the processing is fairly minimal and most importantly low complexity so I can source generate that
Won't this also explode if the enums have complicated objects in them?
Hmm, probably
At least the simple Deserialize call will not work
Would have to call some variant of this recursively instead of calling that Deserialize there
Performance 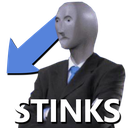
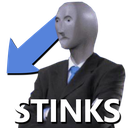
Adding a ok ish solution to this in case anyone stumbles across this later on:
- Use the method I've sent above for doing the parsing from JsonDocument -> Enum type
- Add a JsonConverter to the Enum type that looks like this https://i.imgur.com/fu9226J.png
Only thing that differs between converters is the cases in the Write method. You'll want to manually map the cases of the enum that are just a string to that string instead of using the default converter
Also you can ofc do something smarter than looking for that method via reflection on each Write just a PoC