✅ Get delegate from event
I got class
and PlayerEvents class contains
I want to get delegate from Leaving/Left event but seems like while trying to get value its null? idk
14 Replies
Someone will ask why I didnt add like method in PlayerEvents class which just for example Uses Leaving?.Invoke(args) what I want to create single static method for calling any event with that argument without needing to create x amount of methods just for calling these events
something like
when u add/remove an event listener to the event, the underlying event delegate will change, if its value is
null
, it means that there is currently no listener listening on the event
if u look at the lowered code of
this becomes more obvious:
and if u look at the Delegate.Remove(Delegate, Delegate) method's documentation, it says:
A new delegate with an invocation list formed by taking the invocation list of source and removing the last occurrence of the invocation list of value, if the invocation list of value is found within the invocation list of source. Returns source if value is null or if the invocation list of value is not found within the invocation list of source. Returns a null reference if the invocation list of value is equal to the invocation list of source or if source is a null reference.the highlighted code basically means if the invocation list result would be empty afterwards, it returns
null
seems like without doing something like this its not possible really to easily create single method to invoke x event?
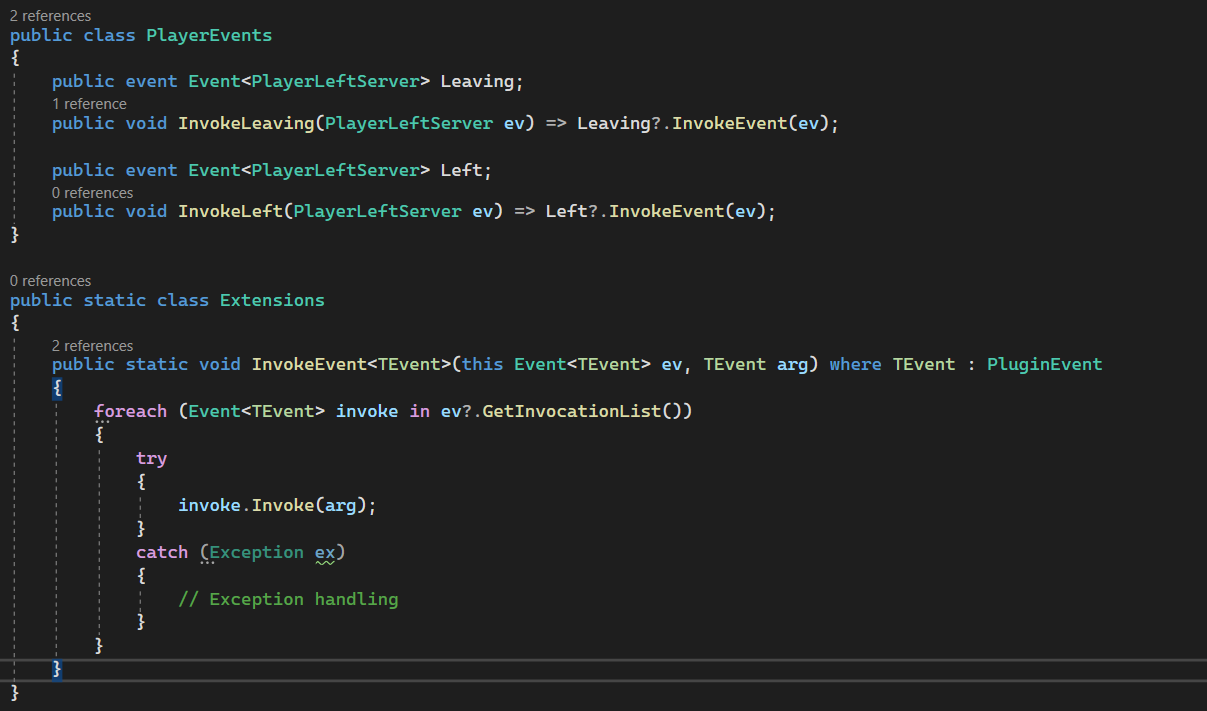
yeah, seems like the only way.
note that usually events are only invocable by the owning type and not from "the outside".
so u would have to write these
InvokeLeft
and alike methods anyway if u want to make that possibleidk if this method is way slower than last one
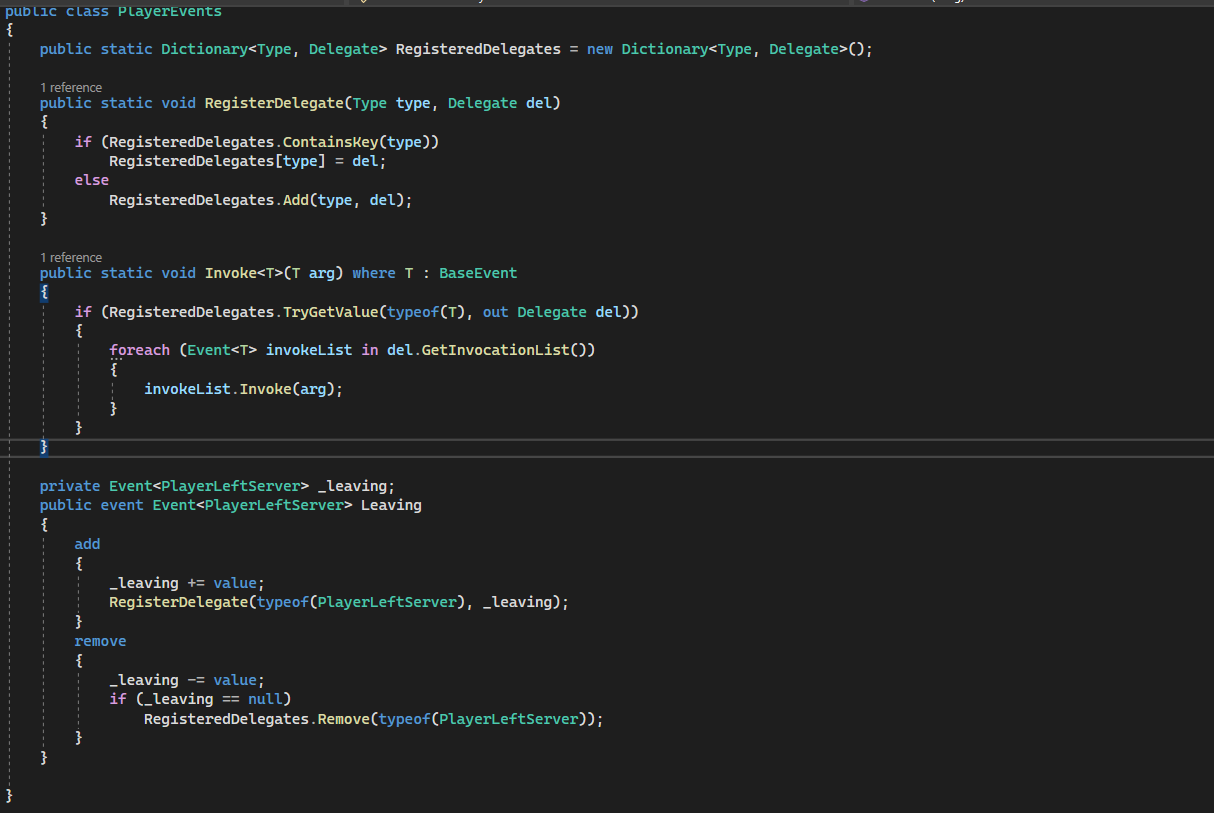
but somehow works
yea first method is better for sure
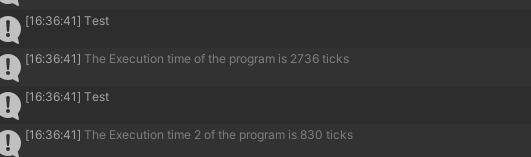
im not really sure what that is supposed to solve
idk I just wanted to have 1 method to execute any event which in that class
its not thread safe, has a lot of dictionary overhead and doesnt handle exceptions per registered listener as ur extension method would do
thanks for help I will just use my extension method
if ur question is answered, please $close the thread 🙂
Use the /close command to mark a forum thread as answered
there is no need to use GetInvocationList. there is no need for
InvokeEvent
at all
you just do Leaving?.Invoke(arg)