Can anyone explain this piece of code for me please ?
I've just started learning how to use Action in Unity, this seem advanced
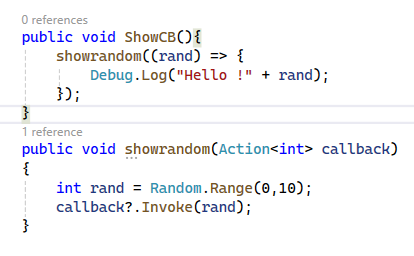
4 Replies
First, you need to understand variable that points to a method
Second, you need to understand lambda expression.
The right hand side of the following assignment is a lambda expression.
You can read
x => Console.WriteLine(x * x * x)
as a function that takes one argument x
of type int
and prints the cube of x
.
Why is the type of x
int
? Because variable_pointing_to_method
is of type Action<int>
.to add on to the last part
Action<int>
expects a method that accepts and int and return nothing aka void method
so the lambda
x => Console.WriteLine(x * x * x);
translates to
The previous examples show how to create a local variable pointing to methods which can be a lambda expression too.
Now we pass the local variable as an argument of
ShowRandom()
.
This argument is called callback because it is called or invoked in ShowRandom()
.
The code below is just a trivial wrapping.
Another non-trivial example.
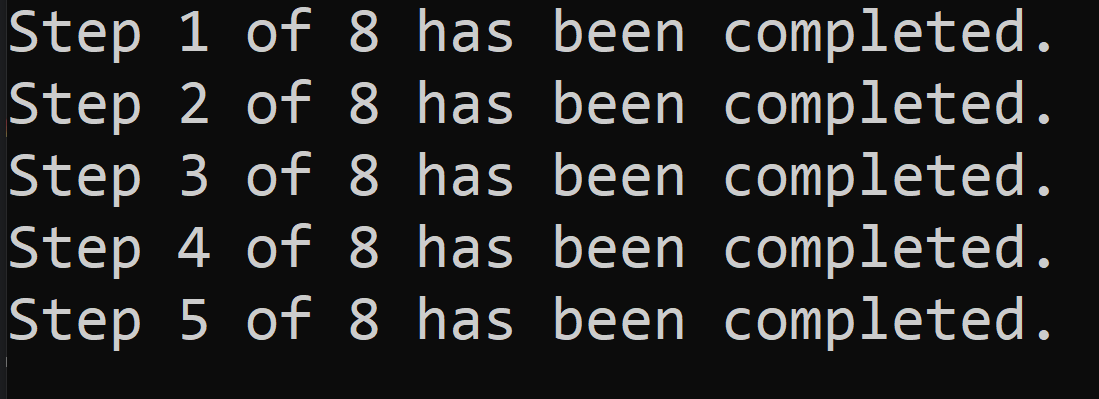