Struggling filtering a table
Lets say i have this filament table:
the objective is to show the songs with more plays.
eloquent relationships explanation:
tables:
songs: id, name
artists: id, name
artist_song: artist_id, song_id
user_song_play_history: user_id, song_id, is_played
relationships:
Song model:
Artist model:
ArtistSong pivot model:
UserSongPlayHistory pivot model:
at the moment works just fine. the problem is when i try to add a filter to filter by user. i get and so far havent found how to solve it:
Expected type 'Illuminate\Database\Eloquent\Builder'. Found 'Closure(mixed, mixed): void'
46 Replies
anyone ?
Does this works for you?
no, i get:
just when loading the dashboard
you're returning nothing from your relationship definition. You've probably forgot to add
return
on your relationship methodi have
cuz i set the return types on all the relationship fns
just checked it, yeah all seems fine in the models fns
you cannot use
plays.user
as a relationship name, you're probably want to create a HasManyThrough to point directly to the users
Song -> HasMany -> User -> Through -> ? plays
(i guess)but is belongstomany
not has many
can i build it around a hasmanythrough?
or there is a "belongstomanythrough" alternative? hmm
please describe the filtering you're trying to achive including the relation between them
GitHub
GitHub - staudenmeir/eloquent-has-many-deep: Laravel Eloquent HasMa...
Laravel Eloquent HasManyThrough relationships with unlimited levels - GitHub - staudenmeir/eloquent-has-many-deep: Laravel Eloquent HasManyThrough relationships with unlimited levels
in the filament dashboard im showing a table with the songs that have more plays
this table doesnt take into account who actually listened the song.
now, the user sees all plays from all the users. i want it to be able to see it filtering by them.
a song is related to a user when a user listens to a song, in that moment a UserSongPlayHistory model is created (which contains user_id, song_id, is_played (boolean))
thanks for the suggestion, im currently trying to setup the relationship
isnt
plays
in the song model the only thing you need? plays is pointing to the user already through playhistory
also, you dont need the is_played column, if there's a row in that table it already means that the user listened to the song. If the user never plays the song, the play history will never be created
you'll never have a is_played
that is false
anywaynot sure about this, can u elaborate more? yeah plays is the relationship between user and song (UserSongPlayHistory model)
so u say that when setting up the select filter, in the relationship ishould only put 'plays' instead of 'user'?
and about this, is needed, cuz i track the history, maybe the user arrived to one song, but skipped it, and they havent played it
understood
i think so, and you probably want to chain
->withPivot('is_played')
to the relationshipi was checking it out, u deleted it? there was some problem?
i've wrote a redundant aproach, you can use your model as is, just use
plays
in the filternot sure what im doing wrong now
doing:
i get:
Call to undefined method App\Models\User::plays()
fn ($query) => $query->where('is_listened', true)
i think it works 🎊
im still testing it out though
oh no, it doesnt. something weird happens:
i have created 3 rows in the user_song_play_history table:

but if u see the table:
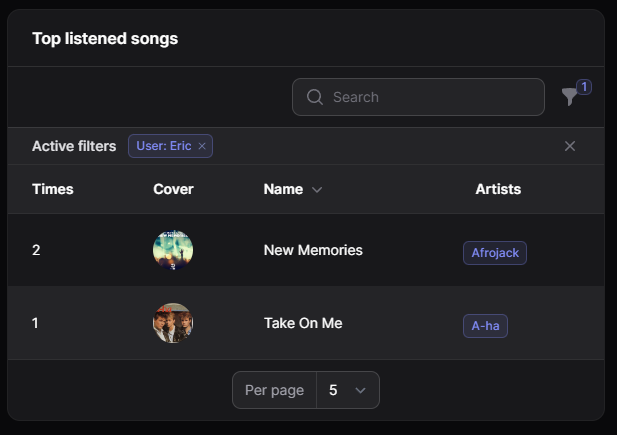
i might be missing something here:
dont answer yet, ill try to fix it
no, must be in the query itself:
somehow i have to tell that when i filter i want to actually filter 😂 🤦♂️
and this is the screenshot filtering by the other user (user id 2)
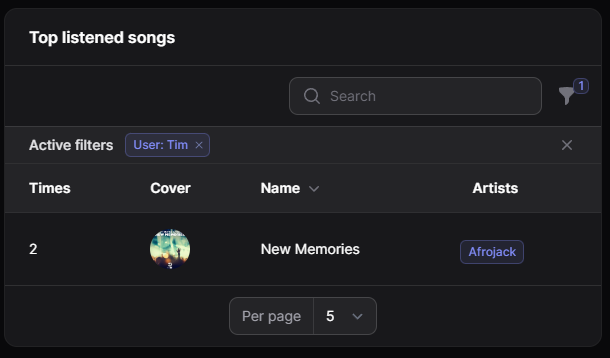
Cool
noo cool no, it doesnt work as expected xd, im near but no
user tim should see only 1 time the song
but is also grabbing the listen from the other user
and eric sees the song "new memories" - times 2, when should be only 1
i keep investigating hmmmmmmm
well, you're half way there, i need to leave for a couple of hour now, i can help you later if you didnt found a solution
okay go, yeah ill keep pushing lets see if i get it
brigado 🙌 🙌 🙌 🙌 🙌
so ive modified the table:
the issue is that the plays count doesnt get modified when filtering by the user
how can i achieve it?
any ideas?
What do you mean by plays_count is not modified when filtering by User?
i mean that i'd like to modify it when filtering the user. let me explain.
when i apply a filter by a user, that plays_count is wrong cuz is including all plays. and somehow i want to include only the user's, but idk how to 🤷♂️
How does the model 'user' looks like?
This is you Table?
yes
not sure if u mean the eloquent relationship:
inside user class:
Your Table is for Song Model and not User model, depends on how all is setup.
What is the query what is being used if you filter?
should i change the query to User model then? 🤔
What is the query what is being used if you filter?not sure how to check that, with debugbar?
Yes you can check that with debug bar.
to ur question about what is the query:
without filtering;

filtering by user with id of 1:

no, this is a songs table, not a users table
You are filtering the main query, you also need to filter the subquery
dont know if that is possible with filters.
hmm and from where i am suposed to get the
$user_id
variable?
not sure how to retrieve it
and seems the code u gave me was given from an ai (nothing wrong, but it seems that xd)True, it was ChatGPT inserted your SQL query and let me explain what is does and what the problem was. Than explains to me how to sole this. (and yes i know what it does but needed to know if the gpt know wat is was)
I see https://filamentphp.com/docs/3.x/tables/filters/getting-started#modifying-the-base-query dont know if this works on SelectFilter and how to get the SelectFilter value
i think i have to modify the filter
and there, apply custom query or something like that
but idk how to do it exactly
I think something like this
im trying it
Undefined array key "user"
also tried to say:
but then seems it doesnt do any filterif you
dd($data)
What is in the data?
i guess we need to get
value
instead of user
?
now the query looks like this:
and the filter:
i cant see where is filtering by the user here 🤷♂️
though im filtering in the table
and the condition is true
and $data['value']
is 1
(user id 1)
might be missing something
now ive done this test:
in the table query function i hardcoded it:
so now im filtering by the user with id 1
and this way works
but with the filters it doesnt
i guess it has something to do with withCount
, which doesnt work on filters or im doing something wrong
any ideas?