AutoMapper best practices
Hey guys! I am using AutoMapper to map my service objects to DTOs, but i was wondering if I am doing it clean.
[HttpPost]
public IActionResult Get()
{
var map = _mapService.GetMap();
var result = _mapper.Map<MapDto>(map);
return Ok(result);
}
How do I make sure that this works? I have created some tests for the controller but they don't feel quite right...
Test startup method:
public MapControllerTests()
{
mapService = new Mock<IMapService>();
mapper = new Mock<IMapper>();
var serviceResponse = new Map { Points = [new Point()] };
mapService.Setup(m => m.GetMap()).Returns(serviceResponse);
mapper.Setup(m => m.Map<MapDto>(serviceResponse)).Returns(new MapDto { Points = [] }); // <-- Feels like too much logic in test
mapController = new MapController(mapper.Object, mapService.Object);
}
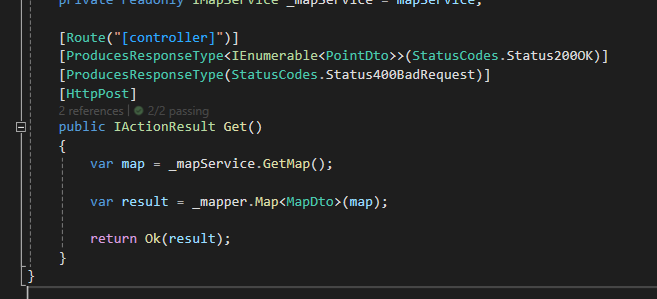
24 Replies
The best practice is to use the IQueryable extension
Instead of fetching everything and the kitchen sink, and then discarding half of the data in mapping
Hmmn how do i do that? 😵💫
https://docs.automapper.org/en/stable/Queryable-Extensions.html
You make it a part of your query
Something like that, IIRC
(note the use of async/await as well, btw)
But isn't that if i am using entity framework?
What else are you using?
I am trying to map an object "map" that is used in the service layer to "mapDto" that will only be used for the output of the controller
for reference i have this config for the mapper
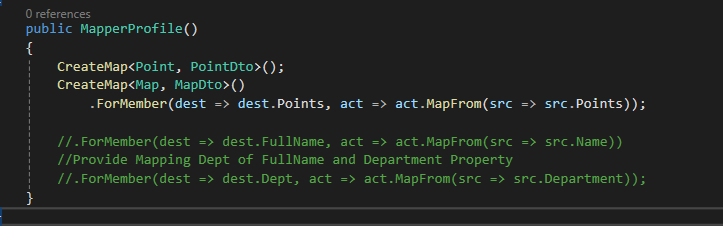
But... you are using EF somewhere, no?
no :/
What are you using, then?
I don't have a database yet
just wanted to separate DTOs from the models used in the service
or is that stupid?
So, uh, you're buidling your app outside-in...?
First the API, then the services, lastly the database...?
yea xD
Well if you don't have the database then do whatever
Makes no difference
I started with some logic and started to be fun and all
no mapping?
Just be prepared to rip it all out and rewrite it once you do hook a database into it
I mean, sure, use the mapper wherever
Without a database it makes no difference where or how
to be honest, I don't think that will be an issue... I am using a object as bd (while running) and when its time to have persistence just need to have that type on the bd split by tables or whatever
its a singleton
have u ever worked with PostgreSQL, MySQL, MSSQL?
I have, MySQL, MSSQL and noSql too
ok I got a lil worried when u said split by tables or whatever
Dont worry, this is in early stages...
i will create the bd soon
im not worried I just relate to what Z said above and can see it happening here
just was trying to understant the best practices
Best practice is not to use automapper