Limit command to specified roles?
I honestly haven't done this in a while (not since pre-application commands) and am new to using Sapphire. Looking to restrict a command to only be visible/usable by the server's owner and any roles they define. How is this best handled with Sapphire and application commands? The bot in question has a database to store roles if that's needed.
Using TypeScript, here's a basic command I wrote that I want to restrict as described:
Already looked over docs and previous questions, though maybe I've missed something, call me out if I have haha
Solution:Jump to solution
You'll have to write and handle your own precondition https://sapphirejs.dev/docs/Guide/preconditions/what-are-preconditions
Sapphire Framework
What are preconditions and how do they work? | Sapphire
Preconditions are classes that will determine whether or not a command should be ran according to certain conditions.
9 Replies
Solution
You'll have to write and handle your own precondition https://sapphirejs.dev/docs/Guide/preconditions/what-are-preconditions
Sapphire Framework
What are preconditions and how do they work? | Sapphire
Preconditions are classes that will determine whether or not a command should be ran according to certain conditions.
I assume just storing role IDs in the database, then checking if the user has one of those roles?
I was hoping there was a way to tell Discord to hide the command from people that didn't have the roles, though I have no idea what exists
the only way with to do it using a role is through discords UI, but that's down to server admins to do, so if its multi-server probably not worth that way
another way is using
default_member_permissions
and then setting a permission only that role should have, e.g. adminThis is only for one server, is that using the intergrations UI in server settings?
yes
if you go in there, find your application itll give you a list of commands. you can select the one that's needed and make it only usable by that role ^^
as a small example, "the gang" is the role i want to be able to use this command, so i enable it for that and disable it for
@everyone
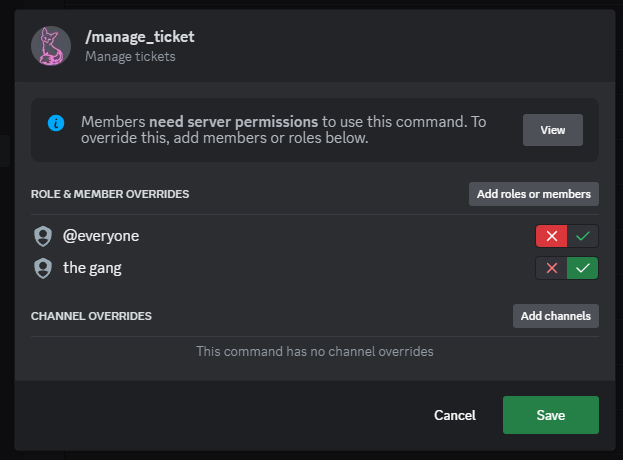
Ahh, very cool. Would this suffice instead of a preconditon, or should I do it just in case?
i'd say it would suffice, its how i handle it where needed
if you wanted you could combine them both but that's down to you
although if you are also using message based commands as well as application commands, you'd need the precondition or atleast some sort of check
Nah, just application commands. I might do it as a precondition still for when the bot's been added but it hasn't been set up in the settings anyway
Might be useful to have a list of authorized roles for that later on anyway