16 Replies
The hashtags are getting created. When applying the hashtags to the post. It's giving me this error.
it says it right there
duplicate key value violates unique constraint "PK_Hashtags"you're trying to create a hashtag with a primary key that already exists
I'm not trying to create the hashtags though. The relationship between Post and Hashtag is many to many. I just want to create an entry in the joining table?
Or have I misunderstood something?
it will create a hashtag if
hashtag
is an untracked entityI see, is there a way I can tell it not to create?
you'd need to fetch those hashtags right there from the same dbcontext where you want to add them to the posts
this is no bueno, returning entities from somewhere, and then adding them to a different dbcontext
The think is that I don't want to rewrite the same code multiple times. There has to be a better way.
At least I now know what the issue is. Thanks mate.
there is always a way 😄 but might take a bit of reorganizing/refactoring
np
BTW i would recommend https://learn.microsoft.com/en-us/ef/core/change-tracking/
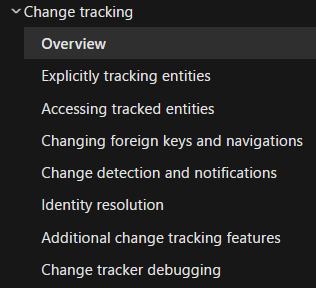
not everything is relatable/needed but good to know the on a base level
@tera Let's see if attach solves anything. Remmended by AI
:kekw:
Yep it fixed it
:A_pepelaugh:
using .Attach is a whole another can of worms, you probably should not be using it generally
cos it usually solves code smell thats caused by something else
so better fix root cause
Damn, I was so happy before Let me see if there is a better way.
well the only way is to refactor the code to fetch those hashtags right there in that method/from the same dbcontext you add them to
Yeah I will try that. Thanks for your help mate. I really appreciate it.
no worries