Adding a Filament form (select component) to a Livewire(v3.x) component
Hi everyone,
I'm trying to implement Filament forms in my livewire component.
What I'm trying to do?
I'm using a filament select component with multiple choices linked to a relation. After saving, the 'interests' aren't displayed upon page refresh, despite being correctly synced in the database. However, when viewed through the filament admin panel, the selected interests are displayed correctly.
Relation in the User model:
Relation in the Interest model:
Note: this very code, Select::make('interests').. in my UserResource filament works just fine, the problem is in the Livewire component.
Filament - UserResource
!image
Livewire -
!image
11 Replies
You never populate the interests array on mount()?
I've tried to use
$this-interests = $this->user->interest;
but it doesn't change anything, just when I click save it doesn't remove the records from the db. But when saving and refreshing the page select input resets.Actually, you shouldn't need
$interests
at all, as you use ->relationship()
. But this ->model(User::class);
should be the actual model in an update form, not the class.Mhm, can you give me an example if that's not a problem. I'll try just now to do the change
Sorry, I am in a meeting now.
You should just change
->model($this->user)
I've changed it, still the select input didnt change its behavior
I've also tried to use CheckBoxList it has similar behavior, it list all interests, and none are checked, then when I check an item every available checkbox is checked... Not exactly sure what I'm doing wrong here.
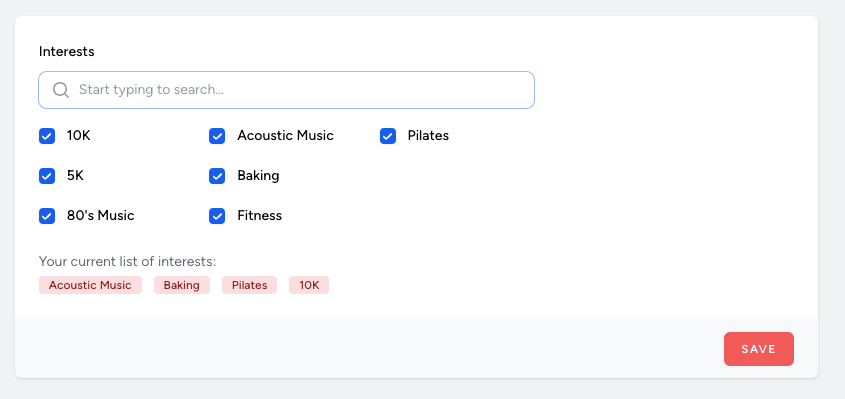
I found a solution.
Thank you Dennis for point out the mount method.
Hm, I thought that this would happen automatically with the
->relationship()
but maybe I was wrongI am fairly sure it should too
Maybe my approach is/was different or wrong, but at the end of the day I've got it working 😅 . If I find any other solution related to this I will update my comment.
I can share the files if you guys want to check?